How to Fix Java Error java.util.InputMismatchException
-
the
java.util.InputMismatchException
in Java -
Causes of the
java.util.InputMismatchException
Error -
Fix the
java.util.InputMismatchException
Error
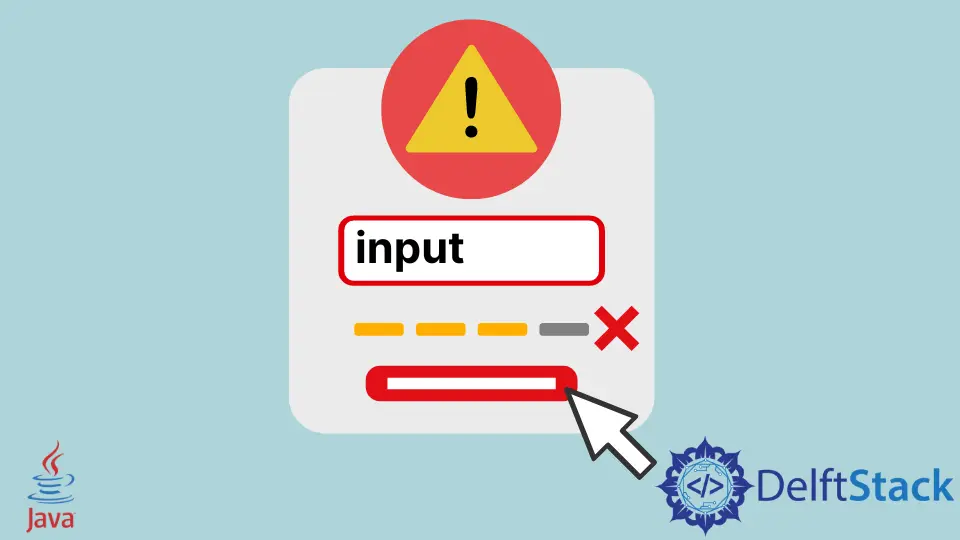
This article will discuss the java.util.InputMismatchException
in the main
thread of the Java
programming language.
the java.util.InputMismatchException
in Java
The JVM will throw java.util.InputMismatchException
whenever it receives one kind of data as input but expects another type of value, which indicates a mismatch in the data. That denotes that the value does not match the regular expression pattern or is outside the acceptable range.
For instance, the program is looking for an integer
value as input to check if it’s even or odd, as shown below.
Code Example:
import java.util.Scanner;
public class example {
public static void main(String[] args) {
int n = 0;
Scanner s = new Scanner(System.in);
for (int i = 0; i < 10; i++) {
System.out.println("Enter a number to check Even/Odd");
n = s.nextInt();
if (n % 2 == 0) {
System.out.println(n + " = EVEN ");
} else {
System.out.println(n + " = ODD ");
}
}
}
}
Output:
Enter a number to check Even/Odd
5
5 = ODD
Enter a number to check Even/Odd
6
6 = EVEN
Enter a number to check Even/Odd
z
Exception in thread "main" java.util.InputMismatchException
at java.util.Scanner.throwFor(Scanner.java:864)
at java.util.Scanner.next(Scanner.java:1485)
at java.util.Scanner.nextInt(Scanner.java:2117)
at java.util.Scanner.nextInt(Scanner.java:2076)
at example.main(example.java:8)
Causes of the java.util.InputMismatchException
Error
The reason that caused java.util.InputMismatchException
in the example is when a Scanner
class is used to receive user input. An InputMissMatchException
will be issued if the parameters given to the method are not valid.
If the value supplied into the nextInt()
function were a String
exception would be thrown. The nextInt()
function can only handle integer values not string values.
Fix the java.util.InputMismatchException
Error
This error occurs due to inaccurate data, and to repair it, we need to rectify the data. In the example, we used the nextInt()
function.
However, it does not support the string parameter. To resolve the java.util.InputMismatchException
, we must check that the input validation is functioning properly and that we are supplying the appropriate data type values.
We’ll use the try catch
method to validate user input. The program returns the following error if the user inputs an invalid value other than an integer.
Error Output:
Input value is Invalid please provide an integer value:
Complete Source Code:
import java.util.Scanner;
public class example {
public static void main(String[] args) {
int n = 0;
try {
Scanner s = new Scanner(System.in);
for (int i = 0; i < 10; i++) {
System.out.println("Enter a number to check Even/Odd");
n = s.nextInt();
if (n % 2 == 0) {
System.out.println(n + " = EVEN ");
} else {
System.out.println(n + " = ODD ");
}
}
} catch (Exception exp) {
System.out.println(" Input value is Invalid please provide an integer value: ");
}
}
}
Output:
Enter a number to check Even/Odd
6
6 = EVEN
Enter a number to check Even/Odd
z
Input value is Invalid please provide an integer value:
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack