How to Draw a Triangle in Java
-
Use
java.awt
andjavax.swing
WithPath2D
to Draw a Triangle in Java -
Use
java.awt
,javax.swing
anddrawPolygon
to Draw a Triangle in Java -
Draw a Triangle Using
drawLine()
in Java
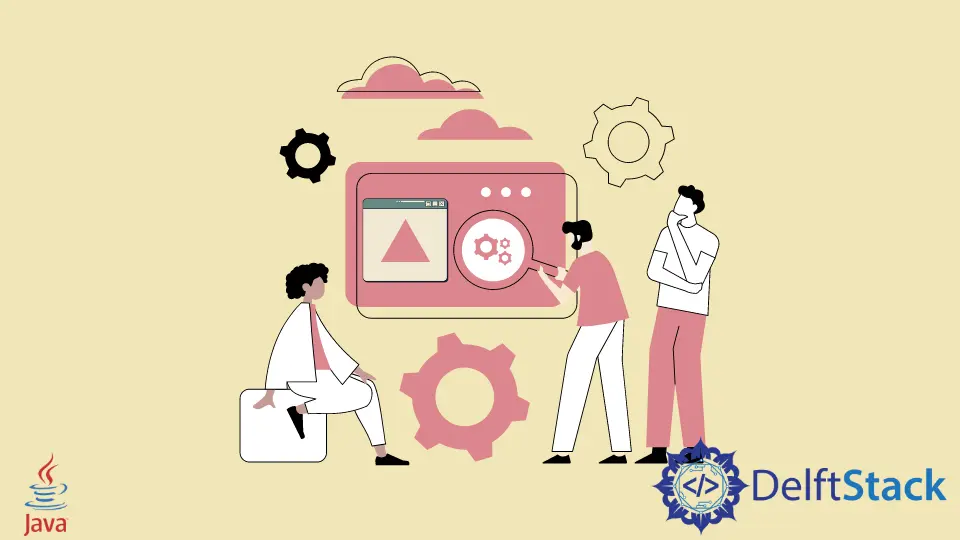
In this article, we will learn how to draw a triangle in Java. We use AWT
(Abstract Window Component Toolkit), the earliest java development packages for graphical programming applications. Swing
is a newly developed GUI widget kit for Java, based on AWT
and has more functionalities.
Use java.awt
and javax.swing
With Path2D
to Draw a Triangle in Java
We use JFrame
in the main
class to create the main window where components like buttons, text fields are added to create a GUI (Graphical User Interface). The class Draw_A_Triangle
extends JPanel
, which is a lightweight container used to organize GUI components. JPanel
is added onto JFrames
using the frame.add()
method.
The Triangle_Shape
class extends the Path2D
class that provides a simple yet flexible shape that represents an arbitrary geometric path. We place the first point using the moveTo()
method and additional points using the LineTo()
method. The close()
method makes sure that the triangle is closed properly.
The paintComponent()
method is called automatically when the panel is created or when the user does something in the user interface that requires redrawing. The Graphics2D
provides more control over color management, geometry, and text layout. Finally, we use g2d
and call draw
to draw a triangle.
import java.awt.*;
import java.awt.geom.Path2D;
import java.awt.geom.Point2D;
import javax.swing.*;
public class Draw_A_Triangle extends JPanel {
public void paintComponent(Graphics g) {
Triangle_Shape triangleShape = new Triangle_Shape(
new Point2D.Double(50, 0), new Point2D.Double(100, 100), new Point2D.Double(0, 100));
Graphics2D g2d = (Graphics2D) g.create();
g2d.draw(triangleShape);
}
public static void main(String[] args) {
JFrame.setDefaultLookAndFeelDecorated(true);
JFrame frame = new JFrame("Draw Triangle");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setBackground(Color.white);
frame.setSize(200, 200);
Draw_A_Triangle panel = new Draw_A_Triangle();
frame.add(panel);
frame.setVisible(true);
}
}
class Triangle_Shape extends Path2D.Double {
public Triangle_Shape(Point2D... points) {
moveTo(points[0].getX(), points[0].getY());
lineTo(points[1].getX(), points[1].getY());
lineTo(points[2].getX(), points[2].getY());
closePath();
}
}
Output:
Use java.awt
, javax.swing
and drawPolygon
to Draw a Triangle in Java
We use JFrame
to create a top-level container, and then add a panel, which is our DrawATriangle
class that extends JPanel
, to it.
As shown in the code below, we call the drawPolygon
method inside the paintComponent
to create a triangle on the Graphics
object g
.
The drawPolygon(int x[], int y[], int numOfPoints)
method draws an outline polygon as per the coordinates specified in the x[]
and y[]
arrays. The numOfPoints
gives the number of points that is 3 in the case of a triangle.
import java.awt.*;
import javax.swing.*;
public class DrawATriangle extends JPanel {
public void paintComponent(Graphics g) {
int[] x = {50, 100, 0};
int[] y = {0, 100, 100};
g.drawPolygon(x, y, 3);
}
public static void main(String[] args) {
JFrame.setDefaultLookAndFeelDecorated(true);
JFrame frame = new JFrame("Draw a Polygon");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setBackground(Color.white);
frame.setSize(300, 200);
DrawATriangle panel = new DrawATriangle();
frame.add(panel);
frame.setVisible(true);
}
}
Output:
Draw a Triangle Using drawLine()
in Java
In the DrawTriangle
class, we extend JComponent
to use the Swing
components like paintCompnent()
and JFrame
. We override paintComponent(Graphics g)
with the Graphics
parameter g
that we can use to call several draw functions.
We call the drawLine()
method to draw a line. As we want to create a triangle that three lines, we need to call drawLine()
three times. drawLine()
takes four arguments, the x and y coordinates of both the first and second points of the line.
The getPreferredSize()
method is called with the return type of Dimension
to specify the window’s dimensions. Finally, to display the triangle, we create a JFrame
object in the main
method and add DrawTriangle
class’s object to it. Note that we have to call jFrame.setVisible(true)
as the frame is initially invisible.
import java.awt.*;
import javax.swing.*;
public class DrawTriangle extends JComponent {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawLine(120, 130, 280, 130);
g.drawLine(120, 130, 200, 65);
g.drawLine(200, 65, 280, 130);
}
public Dimension getPreferredSize() {
return new Dimension(500, 300);
}
public static void main(String[] args) {
JFrame jFrame = new JFrame();
jFrame.add(new DrawTriangle());
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jFrame.pack();
jFrame.setVisible(true);
}
}
Output:
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn