How to Fix Java Cannot Instantiate the Type Error
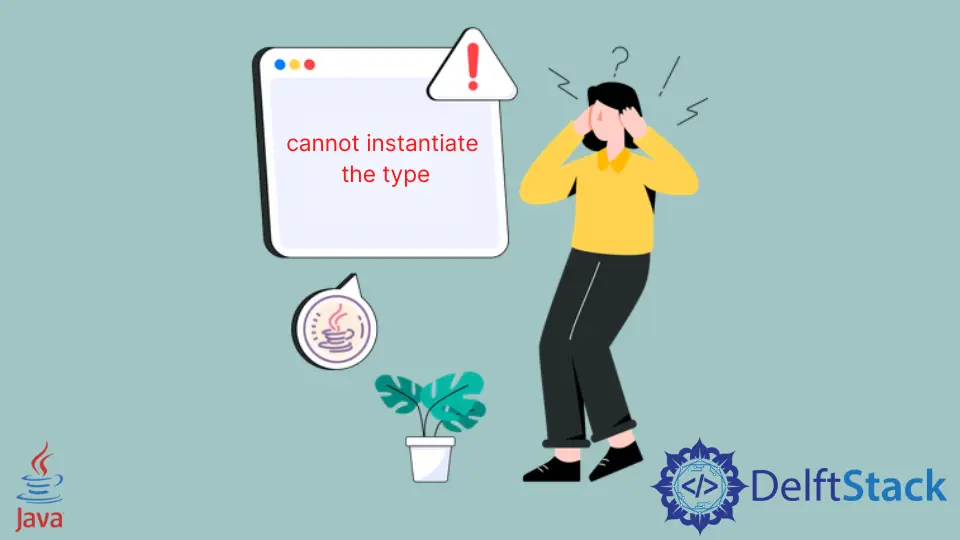
Today, we will learn how to fix the error cannot instantiate the type error
in Java.
This type of error occurs when you try to make an instance of an abstract class. Let’s learn a bit about abstract classes in Java.
Fix cannot instantiate the type
Error in Java
We usually use an abstract class when we need to provide some common functionalities among all its components. You’ll be able to implement your class partially.
You will be able to generate functionalities that all subclasses will be able to override or implement. However, you cannot instantiate the abstract class.
Look at the following code:
abstract class Account { // abstract class Cannot Be initiated...
private int amount;
Account() {
// constructor............
}
public void withDraw(int amount) {
this.amount = this.amount - amount;
}
}
The above abstract class Account
cannot be instantiated. Meaning you cannot write the following code.
Account acc = new Account(); // Abstract Cannot Intialized......
So, what’s the solution? You can create a concrete/child class of this abstract class and make an instance of that.
For instance, there are so many types of accounts. They could be savings, business, debit, and much more.
However, all of them are actual accounts, and that is something that they have in common. That’s why we use abstract methods and classes.
Take a look at the following code.
class BusinessAccount extends Account {
private int Bonus;
public void AwardBonus(int amount) {
this.Bonus = Bonus + amount;
}
}
BusinessAccount
class is a concrete and child class of the abstract Account
class. You can make an instance of this class and get your work done.
BusinessAccount bb = new BusinessAccount();
// Bussiness Account Can Be intiated Because there is concreate defination..........
So, the conclusion is that you cannot instantiate the abstract class; instead, you can create its child class and instantiate it for the same functionality.
The following is a complete code that you can run on your computer.
abstract class Account { // abstract class Cannot Be intiated...
private int amount;
Account() {
// constructor............
}
public void withDraw(int amount) {
this.amount = this.amount - amount;
}
}
class BusinessAccount extends Account {
private int Bonus;
public void AwardBonus(int amount) {
this.Bonus = Bonus + amount;
}
}
public class Main {
public static void main(String[] args) {
// Account acc = new Account(); // Abstract Cannot Intialized......
BusinessAccount bb = new BusinessAccount();
// Bussiness Account Can Be intiated Because there is concreate defination..........
}
}
To learn more about Abstract Class, click here.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack