How to Create Button Click Event in Java
- Java Button Click Events
-
Java Button Click Event With
ActionListener()
-
Java Button Click Event With
ActionListener()
andLambda
-
Java Button Click Event With
ActionListener()
andAnonymous Inner Class
- Conclusion
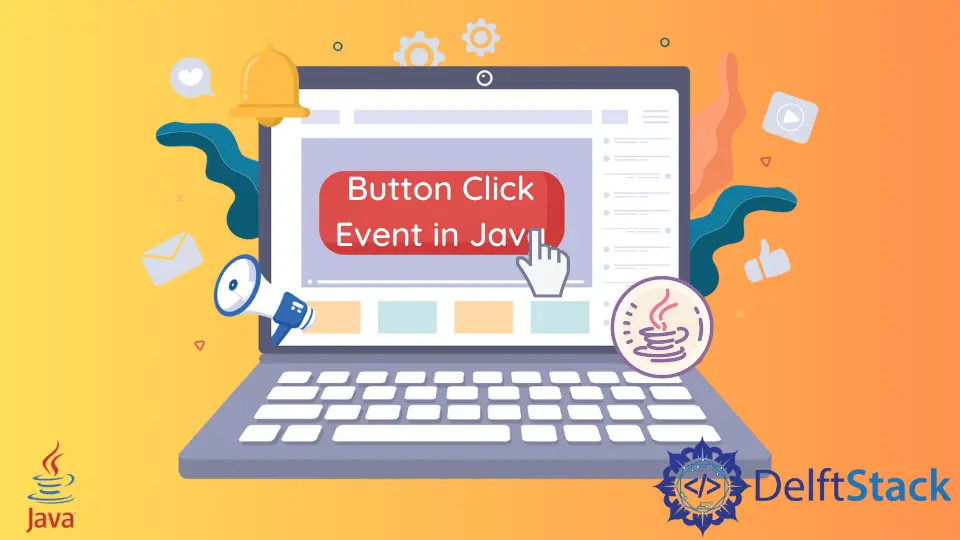
We use an event listener to create a button click event in Java.
This tutorial demonstrates how to create a button click event in Java.
In the world of Java GUI programming, creating responsive user interfaces is essential. One common scenario involves handling button clicks, a fundamental aspect of user interaction.
This article delves into the diverse techniques for handling Java button click events, encompassing direct ActionListener
implementation, lambda expressions
, and anonymous inner classes
. Exploring these methods provides insights into their distinct advantages and empowers developers to choose the most fitting approach for their specific requirements.
Java Button Click Events
Java button click events are crucial for creating interactive and responsive user interfaces in Java applications.
When a user interacts with a graphical user interface (GUI) through buttons, these events trigger specific actions, enabling dynamic functionality. This interactivity enhances user experience by allowing seamless navigation, form submissions, or data processing.
Java button click events facilitate the integration of user input, enabling developers to capture and respond to actions like button presses. This responsiveness is essential for creating intuitive and user-friendly applications, ensuring that users can actively engage with the software.
The table below summarizes the characteristics and considerations for using a direct implementation of ActionListener
, lambda expressions
, and anonymous inner classes
in Java for handling button click events.
Aspect | ActionListener() Direct Implementation |
Lambda Expressions |
Anonymous Inner Classes |
---|---|---|---|
Syntax | Verbose | Compact and Expressive | Flexible and Concise |
Method | Single (actionPerformed ) |
N/A (Handled implicitly) | Multiple methods can be encapsulated |
Readability | Potentially less readable | Improved readability | Readable, especially for complex scenarios |
Boilerplate Code | May introduce boilerplate | Reduces boilerplate | May introduce boilerplate, but less verbose |
Flexibility | Limited | Limited (for simple actions) | High flexibility, especially for complexity |
Use Case | Straightforward cases | Simple, short-lived actions | Complex scenarios requiring multiple methods |
Java Button Click Event With ActionListener()
Making a Java button event using ActionListener
involves implementing the ActionListener
interface, which allows you to define specific actions that should be executed when the button is clicked.
With ActionListener
, developers can encapsulate the desired actions within the actionPerformed
method, promoting a modular design. This modularity enhances code reuse, allowing the same action logic to be easily associated with multiple buttons if needed.
Whether it’s updating the user interface, processing data, or triggering other events, ActionListener
simplifies the process, making button event handling efficient and intuitive in Java applications.
Let’s start with a complete working code example that demonstrates the implementation of a button click event using the ActionListener
interface. We’ll be using the Swing framework for this illustration:
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
public class ButtonClickExample extends JFrame implements ActionListener {
public ButtonClickExample() {
JButton button = new JButton("Click Me");
// Registering ActionListener
button.addActionListener(this);
// Adding button to the frame
add(button);
// Setting frame properties
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button Clicked!");
}
public static void main(String[] args) {
new ButtonClickExample();
}
}
In the initial steps of the Java button click event setup, we begin by importing essential packages such as JButton
, JFrame
, ActionEvent
, and ActionListener
.
Following this, we define a class named ButtonClickExample
, extending JFrame
and implementing the ActionListener
interface. The constructor of this class initializes the graphical components and establishes the main frame.
A JButton
labeled Click Me
is then created to serve as the trigger for the button click event. The ActionListener
interface is registered with the button, using this
to refer to the current class instance.
The button is subsequently added to the frame. To configure the frame properties, we set its size, default close operation, and make it visible.
The actionPerformed
method is overridden within the class to specify the action upon button click, which, in this example, entails printing a message to the console.
Finally, the main method is responsible for creating an instance of the ButtonClickExample
class, initiating the construction of the graphical user interface.
Output:
Button Clicked!
Upon running the program, a window with a button labeled Click Me
will appear. Clicking the button will result in the message Button Clicked!
being printed to the console.
Java Button Click Event With ActionListener()
and Lambda
Instead of directly implementing the ActionListener
interface, lambda expressions allow you to define the action inline, reducing boilerplate code. The syntax is straightforward, using the arrow (->
) to specify the action to be performed when the button is clicked.
This approach is especially suitable for short-lived actions, enhancing code readability and maintainability.
Let’s delve into a complete working code example that demonstrates the implementation of a button click event using lambda expressions. For this illustration, we’ll use the Swing framework:
import javax.swing.JButton;
import javax.swing.JFrame;
public class ButtonClickExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Button Click Example");
JButton button = new JButton("Click Me");
// Lambda Expression for ActionListener
button.addActionListener(e -> System.out.println("Button Clicked!"));
// Adding button to the frame
frame.add(button);
// Setting frame properties
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
We import essential packages to facilitate the creation of GUI components.
Following that, a JFrame
titled Button Click Example
and a JButton
labeled Click Me
are instantiated.
The implementation of a Lambda Expression
directly within the addActionListener
method call succinctly defines the action to be executed upon the button click.
Subsequently, the button is added to the frame. In order to configure the frame, its size is set, the default close operation is specified, and the frame is made visible.
Output:
Button Clicked!
Upon running the program, a window with a button labeled Click Me
will appear. Clicking the button will result in the message Button Clicked!
being printed to the console.
Java Button Click Event With ActionListener()
and Anonymous Inner Class
The anonymous inner class
method provides a concise and localized way to define the action to be performed when a button is clicked.
Creating Java button click events using anonymous inner classes
involves defining the ActionListener
interface inline.
This approach is useful for scenarios where a more complex action or multiple methods need encapsulation within the ActionListener
. The syntax is concise, allowing you to declare the anonymous inner class directly when registering the action.
Let’s delve into a complete working code example that showcases the implementation of a button click event using an anonymous inner class
. For this illustration, we’ll be using the Swing framework:
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
public class ButtonClickExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Button Click Example");
JButton button = new JButton("Click Me");
// Anonymous Inner Class for ActionListener
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button Clicked!");
}
});
// Adding button to the frame
frame.add(button);
// Setting frame properties
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
We import essential packages, including JButton
for creating buttons, JFrame
for the main application window, and ActionEvent
and ActionListener
for handling button events.
Subsequently, a JFrame
titled Button Click Example
and a JButton
labeled Click Me
are created, with the latter set to trigger the button click event.
In order to handle this event, an anonymous inner class
is defined, implementing the ActionListener
interface directly within the addActionListener
method call. Within this class, the actionPerformed
method is overridden to specify the action when the button is clicked.
The button is then added to the frame, and frame properties are set to determine its size, default close operation, and visibility.
Output:
Button Clicked!
Upon running the program, a window with a button labeled Click Me
will appear. Clicking the button will result in the message Button Clicked!
being printed to the console.
Conclusion
Creating Java button click events offers flexibility through various approaches, each with its advantages.
Utilizing the ActionListener
interface provides a traditional yet straightforward method, allowing developers to implement the desired actions directly.
On the other hand, employing lambda expressions
with ActionListener
brings conciseness and expressiveness, particularly beneficial for simple actions, enhancing code readability.
Additionally, utilizing an anonymous inner class
with ActionListener
offers a more flexible approach, accommodating complex scenarios where multiple methods may need encapsulation.
The choice among these methods often depends on coding style, preferences, and the specific requirements of the project, highlighting the adaptability of Java’s event handling mechanisms.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook