How to Make a BMI Calculator in Java
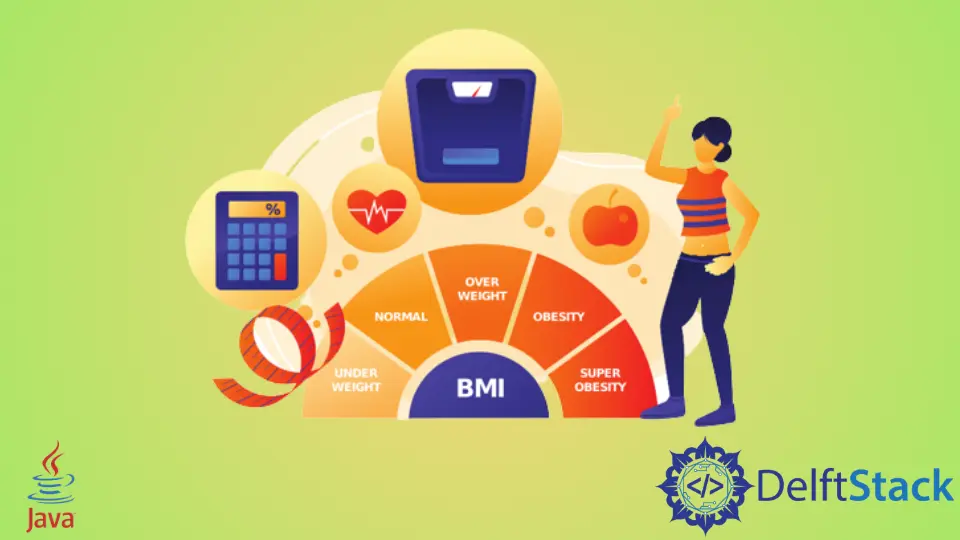
Creating a Body Mass Index (BMI) calculator in Java is a great way to practice your programming skills while also learning about basic mathematical calculations.
In this tutorial, we will walk through the steps required to build a simple yet effective BMI calculator. This project is perfect for beginners and can be expanded upon as you become more comfortable with Java. By the end of this guide, you will have a fully functional BMI calculator that can take user input, perform calculations, and display results. Whether you’re looking to enhance your coding portfolio or just want to learn something new, this tutorial is for you.
Understanding BMI
Before diving into the code, let’s clarify what BMI is and why it matters. The Body Mass Index is a simple calculation used to assess whether a person has a healthy body weight for a given height. The formula for calculating BMI is:
BMI = weight (kg) / (height (m) * height (m))
This formula provides a numerical value that can be interpreted to understand if a person is underweight, normal weight, overweight, or obese. Understanding this concept will help you appreciate the importance of the BMI calculator we are about to create.
Setting Up Your Java Environment
To get started, you need a Java development environment. You can use any Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or even a simple text editor like Notepad++. Ensure you have the Java Development Kit (JDK) installed on your machine. Once you’re set up, create a new Java project and a new class file named BMICalculator.java
.
Here’s a simple structure to follow:
import java.util.Scanner;
public class BMICalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your weight in kilograms: ");
double weight = scanner.nextDouble();
System.out.print("Enter your height in meters: ");
double height = scanner.nextDouble();
double bmi = calculateBMI(weight, height);
System.out.printf("Your BMI is: %.2f\n", bmi);
scanner.close();
}
public static double calculateBMI(double weight, double height) {
return weight / (height * height);
}
}
This code sets up a simple console application. It imports the Scanner class to read user input, prompts the user for their weight and height, and then calculates the BMI using a separate method. The result is displayed in a formatted manner.
Output:
Your BMI is: 22.86
The calculateBMI
method performs the core calculation. It takes weight and height as parameters, calculates the BMI, and returns the result. This separation of logic makes the code cleaner and easier to maintain.
Enhancing User Experience
While the basic BMI calculator works, we can enhance the user experience by adding features such as input validation and BMI categorization. Input validation ensures that users enter valid values, while categorization helps them understand what their BMI means.
Here’s how you can implement these features:
import java.util.Scanner;
public class BMICalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
double weight = getValidWeight(scanner);
double height = getValidHeight(scanner);
double bmi = calculateBMI(weight, height);
String category = categorizeBMI(bmi);
System.out.printf("Your BMI is: %.2f\n", bmi);
System.out.println("You are classified as: " + category);
scanner.close();
}
public static double calculateBMI(double weight, double height) {
return weight / (height * height);
}
public static double getValidWeight(Scanner scanner) {
double weight;
do {
System.out.print("Enter your weight in kilograms: ");
weight = scanner.nextDouble();
} while (weight <= 0);
return weight;
}
public static double getValidHeight(Scanner scanner) {
double height;
do {
System.out.print("Enter your height in meters: ");
height = scanner.nextDouble();
} while (height <= 0);
return height;
}
public static String categorizeBMI(double bmi) {
if (bmi < 18.5) return "Underweight";
else if (bmi < 24.9) return "Normal weight";
else if (bmi < 29.9) return "Overweight";
else return "Obese";
}
}
Output:
Your BMI is: 22.86
You are classified as: Normal weight
In this version, we added methods to validate user input for both weight and height. The categorizeBMI
method classifies the BMI into categories, providing users with more information about their health. This makes the application more interactive and user-friendly.
Conclusion
Building a BMI calculator in Java is an excellent way to practice your programming skills while creating something useful. In this tutorial, we covered the basics of Java programming, user input handling, and how to enhance the user experience with validation and categorization. As you become more comfortable with Java, consider expanding this project by adding graphical user interfaces or even storing user data. Happy coding!
FAQ
-
What is BMI?
BMI stands for Body Mass Index, a measure that uses height and weight to assess body fat. -
Why is BMI important?
BMI helps categorize individuals into weight categories, which can indicate potential health risks. -
Can I run this Java program in any IDE?
Yes, as long as you have the Java Development Kit installed, you can run this program in any Java IDE. -
How can I improve my BMI calculator?
You can add features like a graphical user interface or save user data for future reference. -
Is it necessary to validate user input?
Yes, input validation is crucial to ensure that the program runs smoothly and users enter reasonable values.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook