자바로 BMI 계산기 만들기
Sheeraz Gul
2023년10월12일
Java
Java Calculator
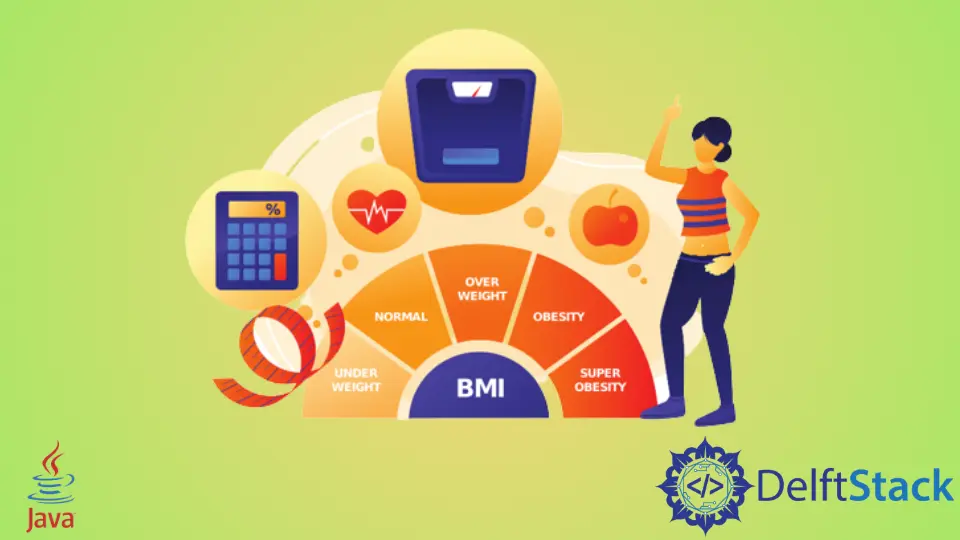
BMI는 체질량 지수를 나타냅니다. 이 튜토리얼은 자바에서 BMI 계산기를 만드는 방법을 보여줍니다.
자바로 BMI 계산기 만들기
체질량 지수 BMI는 키와 체중을 기반으로 건강을 측정합니다. BMI는 체중(kg)을 키(m)의 제곱으로 나누어 계산합니다.
BMI를 측정하는 공식은 다음과 같습니다.
BMI = (Weight in Kilograms) / (Height in Meters * Height in Meters)
BMI에는 아래 표에서 볼 수 있는 범위가 있습니다.
BMI 범위 | 카테고리 |
---|---|
> 30 | 비만 |
25 – 30 | 과체중 |
18.5 – 25 | 보통의 |
< 18.5 | 저중량 |
Java에서 BMI 지수 계산기를 구현해 보겠습니다.
package delftstack;
import java.util.Scanner;
public class Calculate_BMI {
// method to check BMI
public static String BMIChecker(double Weight, double Height) {
// calculate the BMI
double BMI = Weight / (Height * Height);
// check the range of BMI
if (BMI < 18.5)
return "Underweight";
else if (BMI < 25)
return "Normal";
else if (BMI < 30)
return "Overweight";
else
return "Obese";
}
public static void main(String[] args) {
double Weight = 0.0f;
double Height = 0.0f;
String BMI_Result = null;
Scanner scan_input = new Scanner(System.in);
System.out.print("Please enter the weight in Kgs: ");
Weight = scan_input.nextDouble();
System.out.print("Pleae enter the height in meters: ");
Height = scan_input.nextDouble();
BMI_Result = BMIChecker(Weight, Height);
System.out.println(BMI_Result);
scan_input.close();
}
}
위의 코드는 체중과 키에 대한 입력을 받은 다음 BMI 범주를 확인합니다. 출력 참조:
Please enter the weight in Kgs: 79
Please enter the height in meters: 1.86
Normal
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
작가: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook