Java で BMI 計算機を作成する
Sheeraz Gul
2023年10月12日
Java
Java Calculator
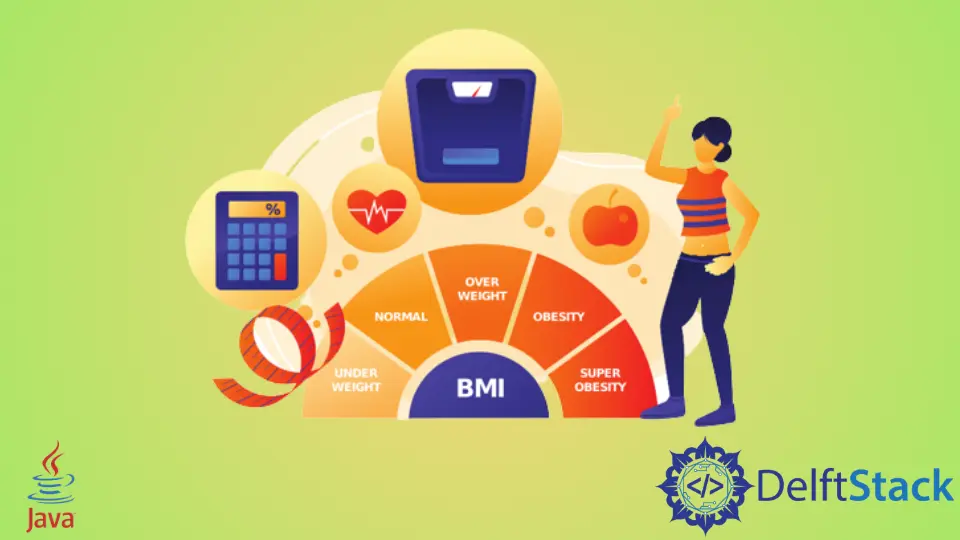
BMI はボディマスインデックスの略です。このチュートリアルでは、Java で BMI 計算機を作成する方法を示します。
Java で BMI 計算機を作成する
Body Mass Index BMI は、身長と体重に基づく健康の測定値です。BMI は、キログラム単位の重量を取得し、メートル単位の高さの 2 乗で割ることによって計算されます。
BMI を取得する式は次のとおりです。
BMI = (Weight in Kilograms) / (Height in Meters * Height in Meters)
BMI には、以下の表に示す範囲があります。
BMI の範囲 | カテゴリー |
---|---|
> 30 | 肥満 |
25 - 30 | 過体重 |
18.5 - 25 | 普通 |
< 18.5 | 低体重 |
Java で BMI インデックス計算機を実装しましょう:
package delftstack;
import java.util.Scanner;
public class Calculate_BMI {
// method to check BMI
public static String BMIChecker(double Weight, double Height) {
// calculate the BMI
double BMI = Weight / (Height * Height);
// check the range of BMI
if (BMI < 18.5)
return "Underweight";
else if (BMI < 25)
return "Normal";
else if (BMI < 30)
return "Overweight";
else
return "Obese";
}
public static void main(String[] args) {
double Weight = 0.0f;
double Height = 0.0f;
String BMI_Result = null;
Scanner scan_input = new Scanner(System.in);
System.out.print("Please enter the weight in Kgs: ");
Weight = scan_input.nextDouble();
System.out.print("Pleae enter the height in meters: ");
Height = scan_input.nextDouble();
BMI_Result = BMIChecker(Weight, Height);
System.out.println(BMI_Result);
scan_input.close();
}
}
上記のコードは、重量と高さの入力を受け取り、BMI のカテゴリを確認します。出力を参照してください:
Please enter the weight in Kgs: 79
Please enter the height in meters: 1.86
Normal
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook