用 Java 製作一個 BMI 計算器
Sheeraz Gul
2023年10月12日
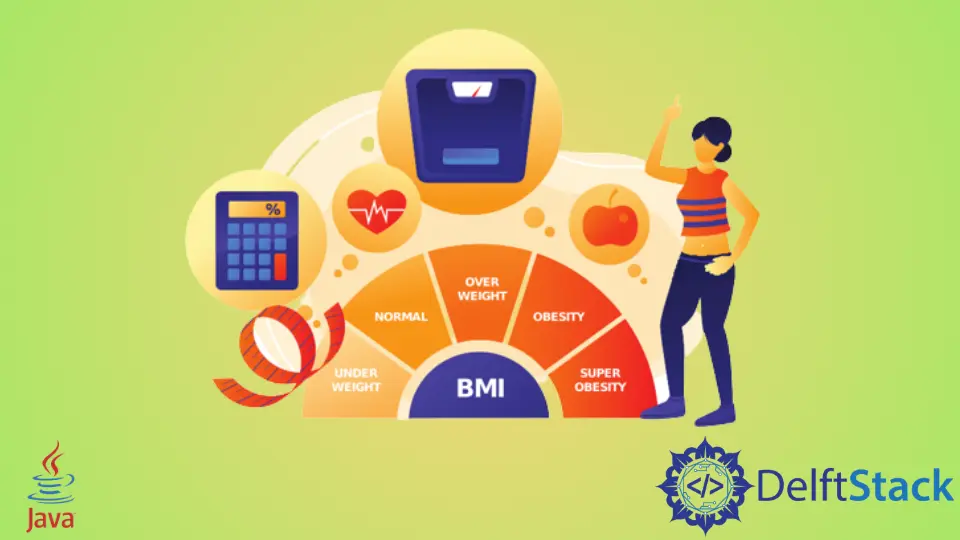
BMI 代表體重指數。本教程演示瞭如何在 Java 中建立 BMI 計算器。
用 Java 製作一個 BMI 計算器
體重指數 BMI 是基於身高和體重的健康指標。BMI 的計算方法是將體重(公斤)除以身高(米)的平方。
計算 BMI 的公式是:
BMI = (Weight in Kilograms) / (Height in Meters * Height in Meters)
BMI 的範圍如下表所示:
BMI 範圍 | 類別 |
---|---|
> 30 | 肥胖 |
25 - 30 | 超重 |
18.5 - 25 | 正常 |
< 18.5 | 體重不足 |
讓我們用 Java 實現 BMI 指數計算器:
package delftstack;
import java.util.Scanner;
public class Calculate_BMI {
// method to check BMI
public static String BMIChecker(double Weight, double Height) {
// calculate the BMI
double BMI = Weight / (Height * Height);
// check the range of BMI
if (BMI < 18.5)
return "Underweight";
else if (BMI < 25)
return "Normal";
else if (BMI < 30)
return "Overweight";
else
return "Obese";
}
public static void main(String[] args) {
double Weight = 0.0f;
double Height = 0.0f;
String BMI_Result = null;
Scanner scan_input = new Scanner(System.in);
System.out.print("Please enter the weight in Kgs: ");
Weight = scan_input.nextDouble();
System.out.print("Pleae enter the height in meters: ");
Height = scan_input.nextDouble();
BMI_Result = BMIChecker(Weight, Height);
System.out.println(BMI_Result);
scan_input.close();
}
}
上面的程式碼將輸入體重和身高,然後檢查 BMI 的類別。見輸出:
Please enter the weight in Kgs: 79
Please enter the height in meters: 1.86
Normal
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook