How to Get the Last Element From an ArrayList in Java
- Importance of Retrieving the Last Element
-
Effective Ways to Retrieve the Last Element from an
ArrayList
-
Best Practices for Last Element Retrieval in
ArrayList
- Conclusion
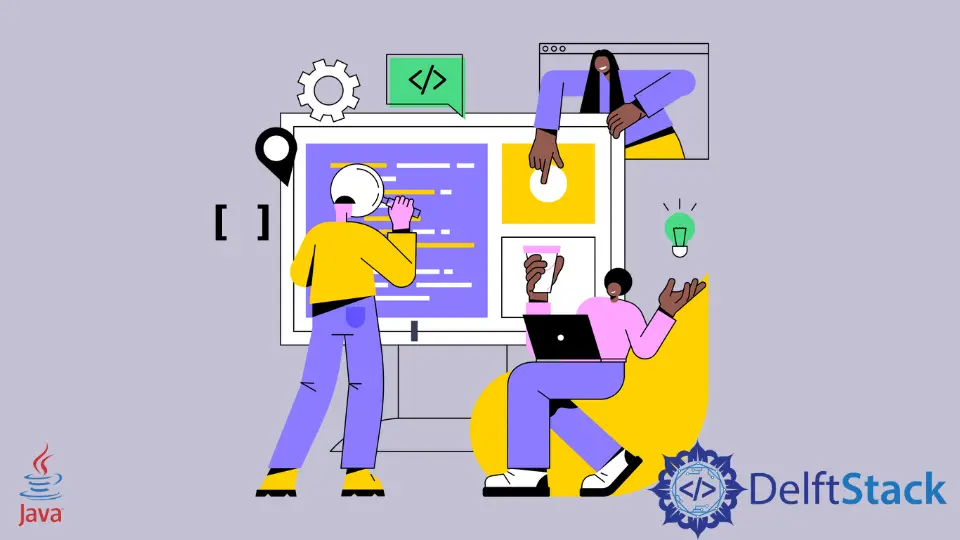
Exploring the significance of retrieving the last element from an ArrayList
, this article discusses effective methods and best practices to optimize this operation, ensuring streamlined and reliable Java code.
An ArrayList
is a dynamic array that can be used to store similar data in an ordered collection. A great thing about Arrays
and ArrayList
is that they allow random access to any element stored in them, given that we know the index where it is stored.
In this tutorial, we will learn how to get the last element from an ArrayList
in Java.
Importance of Retrieving the Last Element
Retrieving the last element in an ArrayList
is crucial for efficient data manipulation and analysis. It allows quick access to the most recent data entry, facilitating tasks such as real-time processing, updating user interfaces, or implementing algorithms that rely on the latest information.
This operation is essential for optimizing code performance and ensuring accurate outcomes in various programming scenarios.
Effective Ways to Retrieve the Last Element from an ArrayList
Using the size()
and the get()
Methods
Using the size()
and get()
methods to retrieve the last element of an ArrayList
is straightforward and commonly employed. It calculates the index of the last element directly, making the process simple and efficient.
While other methods may offer alternative approaches, this technique is often preferred for its clarity, readability, and ease of understanding, especially for those new to Java programming. It provides a direct and reliable way to access the last element, making the code more concise and accessible.
Let’s consider a scenario where you have an ArrayList
of integers named numberList
, and you want to retrieve the last element from it. The following Java code demonstrates how to achieve this using the size()
and get()
methods:
import java.util.ArrayList;
public class LastElementRetrieval {
public static void main(String[] args) {
// Creating an ArrayList of integers
ArrayList<Integer> numberList = new ArrayList<>();
numberList.add(10);
numberList.add(20);
numberList.add(30);
numberList.add(40);
// Retrieving the last element using size() and get() method
int lastIndex = numberList.size() - 1;
int lastElement = numberList.get(lastIndex);
// Displaying the result
System.out.println("Original ArrayList: " + numberList);
System.out.println("Last Element: " + lastElement);
}
}
The code involves creating an ArrayList
named numberList
to store integer values. Subsequently, the ArrayList
is populated with sample integer values.
The last element is retrieved by calculating the index using numberList.size() - 1
and then obtaining the element at that index using numberList.get(lastIndex)
. The result, comprising the original ArrayList
and the last element, is printed to the console.
Upon running the code, the output would be:
Original ArrayList: [10, 20, 30, 40]
Last Element: 40
By employing the size()
and get()
methods, we have efficiently obtained the last element from an ArrayList
. This method is not only concise but also provides a clean and readable way to access elements, contributing to the maintainability of your Java code.
Incorporating such techniques enhances your programming arsenal and promotes effective handling of ArrayLists
in various applications.
Using the Direct Index Method
Utilizing the get()
method with a direct index to retrieve the last element of an ArrayList
is favored for its simplicity and clarity. By directly specifying the index (list.size() - 1
), it offers a concise and easy-to-understand solution.
This method is often preferred over alternatives for its straightforwardness, making the code more readable, especially for developers who value simplicity and directness in their approach.
Consider a scenario where you have an ArrayList
named dataList
containing strings, and you want to fetch the last element from it. Let’s walk through the Java code that accomplishes this using the get()
method with a direct index:
import java.util.ArrayList;
public class LastElementRetrieval {
public static void main(String[] args) {
// Creating an ArrayList of strings
ArrayList<String> dataList = new ArrayList<>();
dataList.add("Apple");
dataList.add("Banana");
dataList.add("Orange");
dataList.add("Grapes");
// Retrieving the last element using get() with direct index
String lastElement = dataList.get(dataList.size() - 1);
// Displaying the result
System.out.println("Original ArrayList: " + dataList);
System.out.println("Last Element: " + lastElement);
}
}
The code begins by initializing an ArrayList
named dataList
designed to store strings. Subsequently, sample string elements are added to the ArrayList
for illustrative purposes.
The last element retrieval involves calculating the index of the last element using dataList.size() - 1
and obtaining the element at that index with dataList.get(lastIndex)
. The result, consisting of the original ArrayList
and the last element, is then printed to the console.
Upon executing the code, the output would be:
Original ArrayList: [Apple, Banana, Orange, Grapes]
Last Element: Grapes
By employing the get()
method with a direct index, we have successfully obtained the last element from the ArrayList
. This technique offers a concise and readable solution for retrieving specific elements based on their position within the list.
Understanding and incorporating such methods enhance your ability to work efficiently with ArrayLists
in Java, contributing to the clarity and effectiveness of your code.
Using the Java 8 Stream API
Leveraging Java 8 Stream API for retrieving the last element of an ArrayList
offers a concise and modern approach. The reduce
operation simplifies the code, making it more expressive.
While other methods exist, the Stream API provides a functional and streamlined solution, aligning with a more modern coding style. This method is favored for its elegance and conciseness, particularly by developers who appreciate the functional programming paradigm introduced in Java 8.
Let’s consider a scenario where we have an ArrayList
of doubles named priceList
, and we aim to retrieve the last element from it. Below is the Java code demonstrating the usage of the Stream API for this task:
import java.util.ArrayList;
public class LastElementRetrieval {
public static void main(String[] args) {
// Creating an ArrayList of doubles
ArrayList<Double> priceList = new ArrayList<>();
priceList.add(49.99);
priceList.add(29.99);
priceList.add(39.99);
priceList.add(59.99);
// Retrieving the last element using Java 8 Stream API
Double lastElement = priceList.stream().reduce((first, second) -> second).orElse(null);
// Displaying the result
System.out.println("Original ArrayList: " + priceList);
System.out.println("Last Element: " + lastElement);
}
}
The process begins by initializing an ArrayList
named priceList
intended for storing double values. Subsequently, sample double values are added to the ArrayList
for illustration.
Retrieving the last element using the Stream API involves converting the ArrayList
into a Stream with priceList.stream()
. The reduction operation .reduce((first, second) -> second)
is then applied to obtain the last element, and .orElse(null)
handles cases where the ArrayList
might be empty.
The result, comprising the original ArrayList
and the last element, is printed to the console.
Upon running the code, the output would be:
Original ArrayList: [49.99, 29.99, 39.99, 59.99]
Last Element: 59.99
The Java 8 Stream API provides an expressive and concise solution for retrieving the last element from an ArrayList
. By leveraging streams and reduction operations, we enhance the readability of our code and promote a functional programming approach.
This method not only simplifies the syntax but also contributes to more efficient and maintainable code. As Java developers, embracing modern features like the Stream API empowers us to write elegant and effective code.
Using the sublist()
Method
Utilizing the subList()
method to retrieve the last element of an ArrayList
provides a specific and focused way to access the desired element. While other methods exist, subList()
allows for a clean and readable solution, creating a sublist that contains only the last element.
This method is valued for its simplicity and directness, offering an efficient way to handle array lists when the focus is on obtaining the last element.
Let’s consider a scenario where we have an ArrayList
of integers named numbers
, and we want to retrieve the last element using the subList()
method. Below is a Java code example demonstrating this technique:
import java.util.ArrayList;
import java.util.List;
public class LastElementRetrieval {
public static void main(String[] args) {
// Creating an ArrayList of integers
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(15);
numbers.add(25);
numbers.add(35);
numbers.add(45);
// Retrieving the last element using the subList() method
List<Integer> lastElementList = numbers.subList(numbers.size() - 1, numbers.size());
int lastElement = lastElementList.get(0);
// Displaying the result
System.out.println("Original ArrayList: " + numbers);
System.out.println("Last Element: " + lastElement);
}
}
The process starts with initializing an ArrayList
named numbers
designated for storing integer values. Subsequently, sample integer values are added to the ArrayList
for illustrative purposes.
Retrieving the last element using the subList()
method involves calculating the index of the last element with numbers.size() - 1
. Then, a sublist
containing only the last element is created using numbers.subList(lastIndex, numbers.size())
.
Finally, the element is retrieved from the sublist
with lastElementList.get(0)
. The outcome, consisting of the original ArrayList
and the last element, is printed to the console.
Upon running the code, the output would be:
Original ArrayList: [15, 25, 35, 45]
Last Element: 45
The subList()
method provides an elegant solution for extracting specific portions of an ArrayList
, including the last element. By creating a sublist, we can seamlessly navigate and manipulate portions of the list without modifying the original structure.
This approach enhances code readability and maintains the integrity of the original ArrayList
. Understanding and utilizing methods like subList()
adds versatility to our Java programming toolkit and contributes to writing more expressive and efficient code.
Converting ArrayList
to ArrayDeque
in Java
Converting an ArrayList
to an ArrayDeque
for retrieving the last element offers efficiency and flexibility. ArrayDeque's
getLast()
method directly retrieves the last element, providing a concise solution.
This approach is favored for its streamlined code and the versatility of ArrayDeque
, especially when considering scenarios where frequent access to both ends of the collection is beneficial. It’s a pragmatic choice for developers looking to optimize last element retrieval in Java applications.
Consider a scenario where we have an ArrayList
of strings named fruits
, and we want to retrieve the last element using the conversion to ArrayDeque
. Below is a Java code example illustrating this technique:
import java.util.ArrayDeque;
import java.util.ArrayList;
public class LastElementRetrieval {
public static void main(String[] args) {
// Creating an ArrayList of strings
ArrayList<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
fruits.add("Grapes");
// Converting ArrayList to ArrayDeque
ArrayDeque<String> dequeFruits = new ArrayDeque<>(fruits);
// Retrieving the last element using ArrayDeque
String lastElement = dequeFruits.getLast();
// Displaying the result
System.out.println("Original ArrayList: " + fruits);
System.out.println("Last Element: " + lastElement);
}
}
The process commences with the initialization of an ArrayList
named fruits
, intended for storing string values. Subsequently, string elements are added to the ArrayList
for illustrative purposes.
To retrieve the last element efficiently, the ArrayList
fruits
is then transformed into an ArrayDeque
named dequeFruits
. The last element is obtained using the getLast()
method of the ArrayDeque
.
The result, which includes the original ArrayList
and the last element, is printed to the console.
Upon running the code, the output would be:
Original ArrayList: [Apple, Banana, Orange, Grapes]
Last Element: Grapes
The conversion of an ArrayList
to an ArrayDeque
introduces a fluid approach to last element retrieval. ArrayDeque's
ability to efficiently access both ends aligns with the need to fetch the last element effortlessly.
This technique not only simplifies the code but also provides an alternative perspective on managing collections in Java. Understanding the versatility of data structures, such as ArrayDeque
, contributes to a developer’s toolkit for creating robust and efficient Java applications.
Converting ArrayList
to LinkedList
in Java
Converting an ArrayList
to a LinkedList
for retrieving the last element offers a straightforward solution. LinkedList
’s getLast()
method efficiently accesses the last element.
This approach is valued for its simplicity and the inherent strengths of LinkedList
in managing elements at both ends. It’s an effective choice, especially when quick access to the last element is a priority, making it suitable for certain use cases in Java programming.
Consider a scenario where we have an ArrayList
of integers named numbers
, and we want to retrieve the last element using the conversion to a LinkedList
. Below is a Java code example illustrating this technique:
import java.util.ArrayList;
import java.util.LinkedList;
public class LastElementRetrieval {
public static void main(String[] args) {
// Creating an ArrayList of integers
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(15);
numbers.add(25);
numbers.add(35);
numbers.add(45);
// Converting ArrayList to LinkedList
LinkedList<Integer> linkedNumbers = new LinkedList<>(numbers);
// Retrieving the last element using LinkedList
int lastElement = linkedNumbers.getLast();
// Displaying the result
System.out.println("Original ArrayList: " + numbers);
System.out.println("Last Element: " + lastElement);
}
}
The process starts with the creation of an ArrayList
named numbers
designed for storing integer values. Subsequently, integer values are added to the ArrayList
for illustrative purposes.
To streamline the process of fetching the last element, the ArrayList
numbers
is then transformed into a LinkedList
named linkedNumbers
. Retrieving the last element is achieved using the getLast()
method of the LinkedList
.
The result, comprising the original ArrayList
and the last element, is printed to the console.
Upon running the code, the output would be:
Original ArrayList: [15, 25, 35, 45]
Last Element: 45
Converting an ArrayList
to a LinkedList
offers a seamless approach to last element retrieval. LinkedList
’s efficient handling of elements at both ends aligns with the need to fetch the last element effortlessly.
This technique not only simplifies the code but also provides an alternative perspective on managing collections in Java. Understanding the strengths and use cases of different data structures enhances a developer’s ability to make informed choices, contributing to the creation of efficient and scalable Java applications.
Best Practices for Last Element Retrieval in ArrayList
Direct Index Access
When retrieving the last element from an ArrayList
, favor using the direct index approach (size() - 1
) combined with the get()
method. This provides a straightforward and readable solution.
Consideration for Empty ArrayList
Always handle scenarios where the ArrayList
might be empty. Implement checks to avoid exceptions, ensuring a graceful response when attempting to retrieve the last element from an empty list.
Streamlined Code
Prioritize code readability and simplicity. Choose methods that make the code concise and easy to understand, enhancing maintainability and collaboration with other developers.
Exception Handling
Implement robust error handling to manage potential exceptions, such as IndexOutOfBoundsException
. This ensures that your application remains stable even in unexpected situations.
Testing
Include comprehensive testing to validate the functionality of your last element retrieval implementation. This ensures the reliability of your code under various scenarios and edge cases.
Choosing the Right Method
Consider the specific needs of your application. While various methods exist, select the one that aligns with your code structure, performance requirements, and overall design principles.
Code Documentation
Provide clear comments and documentation for your last element retrieval implementation. This aids in understanding the purpose and functionality of the code for both current and future developers.
Performance Optimization
Be mindful of performance considerations, especially in situations where last element retrieval is a frequent operation. Evaluate and choose methods that align with the efficiency requirements of your application.
Conclusion
Mastering the art of retrieving the last element from an ArrayList
is pivotal for efficient Java programming. Understanding the importance of this operation in real-time scenarios sets the foundation for streamlined applications.
Embracing effective methods, such as direct index access and leveraging Java features like the Stream API, empowers developers to write concise and readable code.
Best practices, including exception handling and performance considerations, ensure robust implementations. By incorporating these practices, developers can navigate ArrayLists
with precision, enhancing code reliability and maintainability.