How to Call a Method in Another Class in Java
- Call a Method in Another Class in Java
-
Call a
static
Method in Another Class in Java -
Call a
protected
Method in Another Class in Java -
Call a
public
Method in Another Class in Java
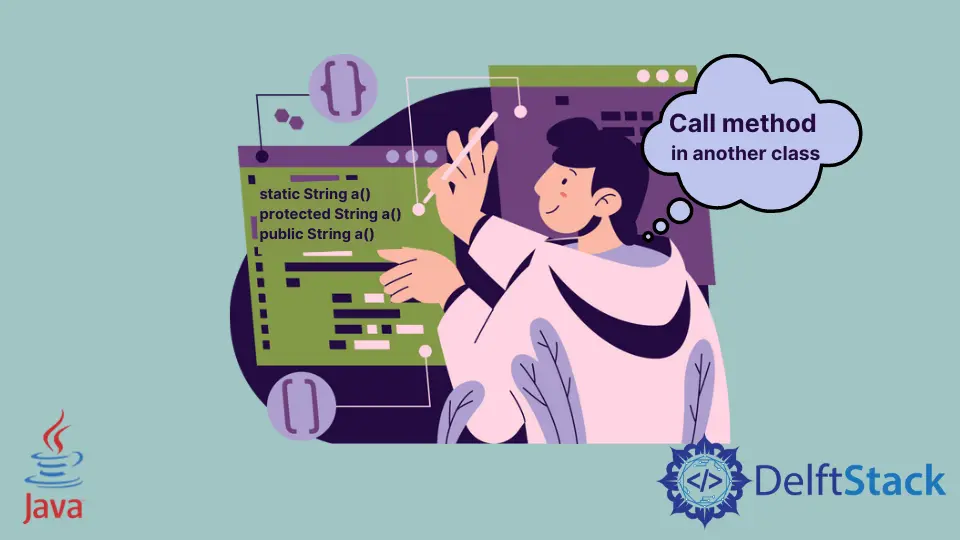
This tutorial introduces how to call a method of another class in Java.
In Java, a class can have many methods, and while creating applications, we can call these methods into the same class and another class. There can be several scenarios where a method can be called in another class. So, let’s start with examples.
Call a Method in Another Class in Java
To class a method of another class, we need to have the object of that class. Here, we have a class Student
that has a method getName()
. We access this method from the second class SimpleTesting
by using the object of the Student
class. See the example below.
class Student {
String name;
Student(String name) {
this.name = name;
}
public String getName() {
return this.name;
}
}
public class SimpleTesting {
public static void main(String[] args) {
Student student = new Student("John");
String name = student.getName();
System.out.println("Student name is : " + name);
}
}
Output:
Student name is : John
Call a static
Method in Another Class in Java
It is another scenario where we are calling a static method of another class. In the case of a static method, we don’t need to create an object to call the method. We can call the static
method by using the class name as we did in this example to call the getName()
static method. See the example below.
class Student {
static String name;
static String getName() {
return name;
}
}
public class SimpleTesting {
public static void main(String[] args) {
Student.name = "John";
String name = Student.getName();
System.out.println("Student name is : " + name);
}
}
Output:
Student name is : John
Call a protected
Method in Another Class in Java
If the instance method of a class is declared as protected
, it can be called only inside the subclass. Here, we extend the Student
class into the SimpleTesting
class and call the getName()
method using the object of SimpleTesting
class. See the example below.
class Student {
protected String name;
protected String getName() {
return this.name;
}
}
public class SimpleTesting extends Student {
public static void main(String[] args) {
SimpleTesting st = new SimpleTesting();
st.name = "John";
String name = st.getName();
System.out.println("Student name is : " + name);
}
}
Output:
Student name is : John
private
methods of any class into another class since private methods are only limited to the same class.Call a public
Method in Another Class in Java
A method declared as the public
is available for outside access and can be called into another class. Here, we called a public method getName()
into another class by using the object of Student
class. See the example below.
class Student {
public String name;
public String getName() {
return this.name;
}
}
public class SimpleTesting {
public static void main(String[] args) {
Student st = new Student();
st.name = "John";
String name = st.getName();
System.out.println("Student name is : " + name);
}
}
Output:
Student name is : John