How to Get Value From JTextField
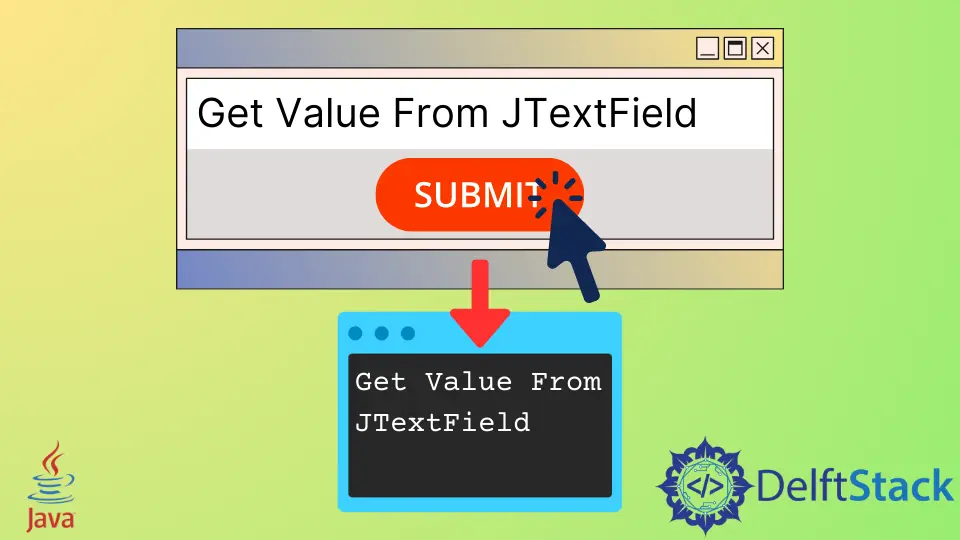
The Java programming provides utilities and functions to draw various real-time images and components for the visual representation. The classes provide various methods for setting the layout, size, color that encapsulated in various classes. The functions become the base of graphics programming. Some packages hold the graphics utility classes. The java.awt
javax.swing
package provides the graph and applet functioning.
Below is the code block to demonstrate the same.
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class ValueFromJTextField extends JFrame implements ActionListener {
JTextField textField;
JButton submitButton = new JButton("Submit");
public ValueFromJTextField() {
JPanel myPanel = new JPanel();
add(myPanel);
myPanel.setLayout(new GridLayout(3, 2));
textField = new JTextField();
myPanel.add(textField);
myPanel.add(submitButton);
submitButton.addActionListener(this);
}
public void actionPerformed(ActionEvent actionEvent) {
if (actionEvent.getSource() == submitButton) {
String data = textField.getText();
System.out.println(data);
}
}
public static void main(String args[]) {
ValueFromJTextField g = new ValueFromJTextField();
g.setLocation(10, 10);
g.setSize(300, 300);
g.setVisible(true);
}
}
In the above code block, the actual execution starts from the main
method.
First, an instance of the ValueFromJTextField
class gets instantiated using a new keyword. It internally calls the public constructor of the class. Into the constructor, some variables get initialized. An instance of JPanel
gets instantiated that creates a new panel with the double buffer initially. The class is present in the javax.swing
package and has enough features to represent Graphics in Java. The add
method is present in the Container
class, present in the java.awt
package. The add
method takes a Component
instance as a parameter then appends the passed instance to the end of the Container
component. The function throws NullPointerException
when the element passed is a null value.
After the panel is added to the Container, the size can be set using the setLayout
function. The instance of Layout
gets passed to the function and does not return anything. A GridLayout
constructor sets the values of rows and columns. Into the grid, a text field gets created and inserted into it using the add method. Next to the text field, a JBotton
instance gets created and inserted in panel. As the last statement, an action listener gets added to submit button instance.
Below the constructor, an action listener method gets implemented. The function takes an instance of the ActionEvent
class. The class depicts a sort of action or event that gets performed over the component. Inside the code block, the source event gets checked using the getSource
method. So the action event instance has a predefined function to check the source of the event from where it seems to get generated. So, conditionally source gets checked to originate from the button instance.
The textField
instance gets used to calling the getText
method. This function returns the text present in the text field component and throws NullPointerException
when the document is a null value. Finally, the text string gets printed in the console output using the println
function.
The console output of the above code block gets shown below.
Hi, This is Jack Daniel
The layout of the Container gets shown below.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn