GC Overhead Limit Exceeded Error in Java
- Understanding the GC Overhead Limit Exceeded Error
- Increasing the Heap Size
- Analyzing Memory Usage
- Optimizing Your Code
- Conclusion
- FAQ
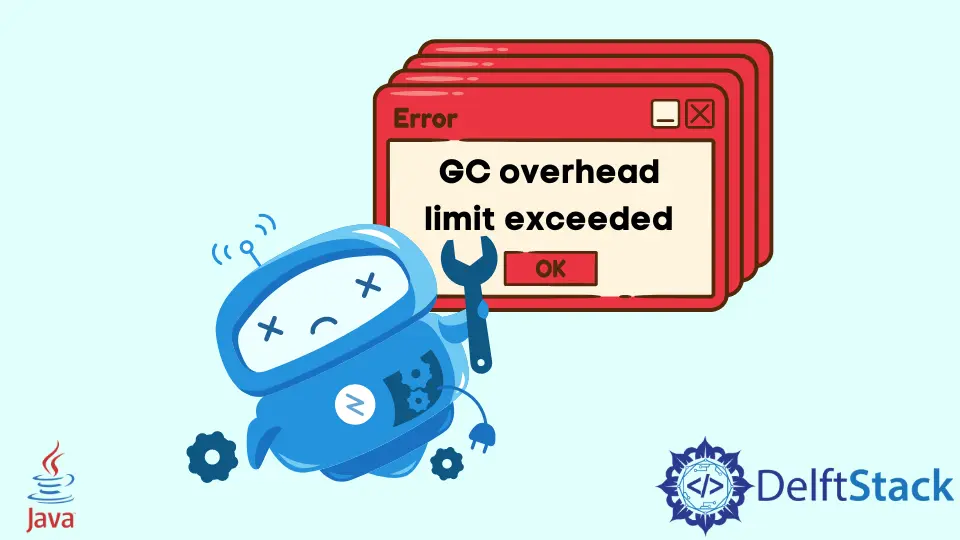
Garbage collection is a crucial part of Java’s memory management system. However, developers often encounter the “GC Overhead Limit Exceeded” error, which can be frustrating and challenging to debug. This error typically indicates that the Java Virtual Machine (JVM) is spending too much time on garbage collection, leaving little time for the application to run. Understanding the underlying causes of this error and knowing how to address it is essential for any Java programmer.
In this article, we’ll dive into what this error means, explore its causes, and discuss effective solutions to resolve it. By the end of this read, you’ll have a clearer understanding of how to tackle the GC Overhead Limit Exceeded error in Java.
Understanding the GC Overhead Limit Exceeded Error
The “GC Overhead Limit Exceeded” error occurs when the JVM is spending excessive time on garbage collection, usually more than 98% of its time, while recovering less than 2% of the heap memory. This situation often arises when your application is running out of memory, leading the garbage collector to work overtime.
When this happens, the JVM triggers this error to prevent further degradation of application performance. It serves as a warning that your application is in trouble. Common causes include memory leaks, inefficient algorithms, or simply not allocating enough heap size for your application. Recognizing these issues early can save you from major headaches down the line.
Increasing the Heap Size
One of the most straightforward solutions to the GC Overhead Limit Exceeded error is to increase the heap size allocated to your Java application. By providing more memory, you can reduce the frequency of garbage collection cycles, allowing your application to perform more efficiently.
To increase the heap size, you can use the -Xmx
and -Xms
flags when starting your Java application. The -Xms
flag sets the initial heap size, while the -Xmx
flag sets the maximum heap size. For example, if you want to set the initial heap size to 512 MB and the maximum heap size to 2 GB, you would run:
java -Xms512m -Xmx2g -jar your-application.jar
Output:
Application started with increased heap size
Increasing the heap size can be particularly effective for applications that require a lot of memory, such as those processing large datasets or handling numerous concurrent users. However, it’s essential to monitor your application’s performance after making this change; simply increasing the heap size may not always be the best long-term solution.
Analyzing Memory Usage
Another effective way to tackle the GC Overhead Limit Exceeded error is to analyze your application’s memory usage. Java provides several tools for this purpose, including VisualVM and JConsole. These tools allow you to monitor memory consumption in real-time, helping you identify memory leaks or objects that are consuming an excessive amount of memory.
Using VisualVM, for instance, you can connect to your running Java application and view various metrics related to memory usage. Look for trends in memory consumption over time and identify any patterns that may suggest a memory leak. If you notice that memory usage continually increases without being released, you may need to investigate further.
Here’s how you can start VisualVM:
jvisualvm
Output:
VisualVM launched successfully
By pinpointing memory issues, you can make informed decisions about optimizing your code or adjusting your memory settings. This proactive approach can significantly reduce the chances of encountering the GC Overhead Limit Exceeded error in the future.
Optimizing Your Code
Sometimes, the root cause of the GC Overhead Limit Exceeded error lies in the code itself. Inefficient algorithms, unnecessary object creation, and poor data structures can lead to excessive memory usage and frequent garbage collection.
To optimize your code, start by reviewing your algorithms and data structures. Are you creating too many temporary objects? Are there opportunities to reuse existing objects instead? Consider using data structures that are more memory-efficient for your use case. For example, if you are frequently adding and removing elements from a list, using a LinkedList instead of an ArrayList might be more efficient.
Here’s a simple example of optimizing object creation:
List<String> myList = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
myList.add("String " + i);
}
Output:
List populated with strings
In this example, instead of creating a new string for each iteration, consider reusing a string buffer or using StringBuilder to reduce memory overhead. These small changes can lead to significant improvements in performance and memory usage, ultimately helping to prevent the GC Overhead Limit Exceeded error.
Conclusion
The GC Overhead Limit Exceeded error in Java can be a significant obstacle for developers, but understanding its causes and solutions can make a world of difference. By increasing the heap size, analyzing memory usage, and optimizing your code, you can effectively mitigate this error and improve your application’s performance. Remember, prevention is key. Regularly monitor your application’s memory consumption and optimize your code to ensure a smoother experience for both you and your users.
FAQ
-
What does the GC Overhead Limit Exceeded error mean?
It indicates that the JVM is spending too much time on garbage collection and recovering very little memory. -
How can I increase the heap size in Java?
You can use the-Xms
and-Xmx
flags when starting your application to set the initial and maximum heap size. -
What tools can I use to analyze memory usage in Java?
Tools like VisualVM and JConsole can help you monitor memory consumption and identify potential memory leaks.
-
How can I optimize my code to prevent this error?
Review your algorithms and data structures, reduce unnecessary object creation, and consider reusing objects where possible. -
Is increasing the heap size always the best solution?
Not necessarily. While it can help, it’s important to analyze memory usage and optimize your code for a more sustainable solution.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack