How to Fix Java Unresolved Compilation Error
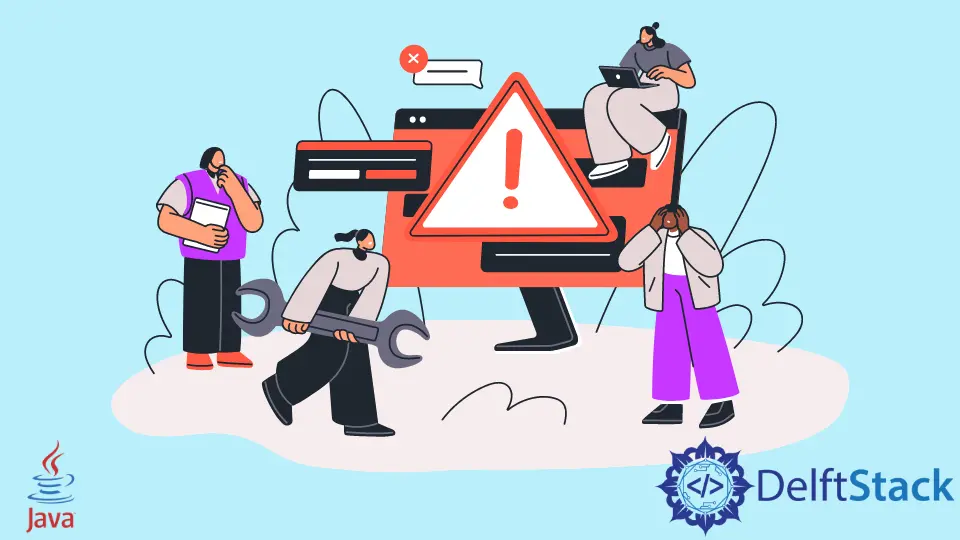
Java works over the three components: JVM, JRE, and JDK.
JVM (Java Virtual Machine) does not physically exist and provides an environment to run the byte code. This bytecode can also get used to running on another machine as they are platform-independent.
Similarly, JRE is the runtime environment that holds JVM and supporting libraries and jars to load the programs in the system. And JDK is the Java Development Kit needed to build and develop enterprise-level solutions.
All the components together constitute the application to build and run properly. If any function breaks, it leads to compile time or run time issues.
The Java language works first to convert source code to the class file. And the runtime environment uses the class file or the bytecode to run the actual program.
The conversion process of source code to class file or machine-understandable byte code is called a compilation. The compilation is the crucial part of the application run process that needs to get passed before the application runs.
The compilation process deals with solving the syntactical and semantics issues, optimization issues, and making correct use of features, which is the actual work of the compiler. The JVM runs the application by first loading the code, verifying the loaded changes and executing the application, and lastly make a runtime environment to run the code.
Below is the code block to show compilation issues in Java.
public class CompilationIssues {
public static void main(String[] args) {
MyClass myClass = new MyClass();
System.out.println("The instance of MyClass is : " + myClass);
}
}
The CompilationIssues
class holds the main
method in the above code block.
The method has an instance of MyClass
that gets created using a new keyword. The object gets appended with the print-stream function to print the object of the newly created object.
The observations related to the program given above are:
If an IDE like Intellij, Eclipse, and Netbeans gets used, it will populate an error in red color saying the MyClass
class is not available.
The editor provides various suggestions to import the class if present in the scope. If the class name gets not found, it suggests creating another new Class in the package with the same name.
The screenshot of options from the IDE is as below.
If the file is a simple Java file that gets written in notepad or no special Java editor, then saving and compilation is the solution.
That way, it will give the error in the below-given format. Additionally, it will provide a suggestion of what line actual issue exists. One can eradicate or resolve the problem with the error definition and stack trace only.
Below is the output for the above compilation issue error.
C :\Users\IdeaProjects\Test\src\main\java\CompilationIssues.java : 3 : 9 java
: cannot find symbol symbol : class MyClass location : class CompilationIssues
The resolution of such a problem can be having a keen eye over the stack trace.
One can observe and understand the issue clearly and provide a solution. The above issue can get resolved by creating the class if not present.
And if the class showing error gets already existing, import the package in the class that holds the main
method, and where the issue gets populated, using an import statement import org.test.MyClass
statement. This import statement will resolve the compilation issues.
The compilation can also occur when the rules defined in Java do not meet its condition. Like exception handling, unreachable code, scope or variable, public-private access modifiers usage, initializing the variables are some of compilation issues examples.
The worst-case is, there can be incompatible versions of Java. Like Java 11 features and setting the environment Java variable as Java 8.
One should keep a proper note and observe the error message that populates when the program compiles. It will help in resolving the issue quickly and appropriately.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack