How to Fix Execution of Java Constructors in Inheritance
- Execution of Java Constructors in Inheritance
- Execution of Default Java Constructor in Inheritance
- Execution of Java Constructor in Inheritance When Parent Class Has Default & Parameterized Constructor
-
Use
super
to Call the Parameterized Constructor of Parent Class and All Child Classes
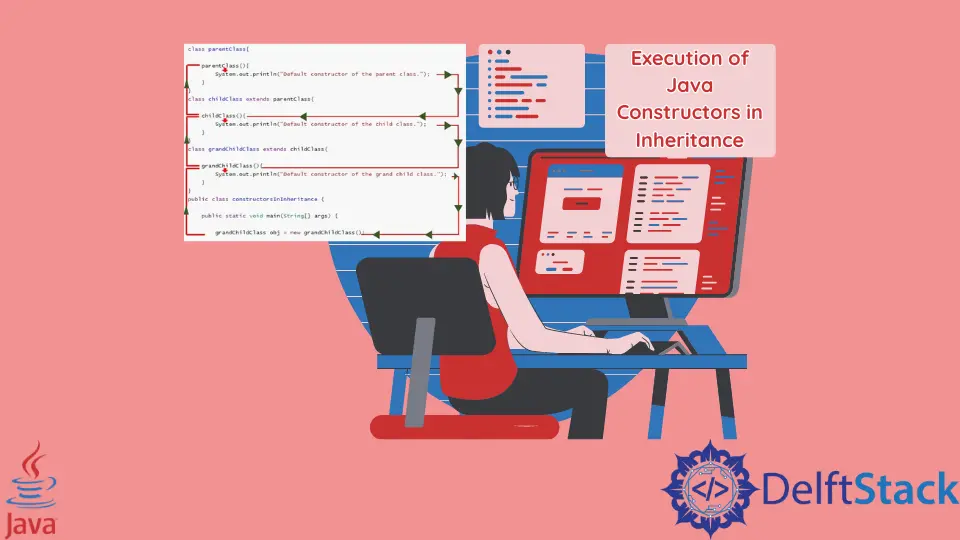
Today, we will learn about the execution of Java constructors in inheritance. We will see code examples of the default and parameterized constructors in the derived classes (also called the child classes and sub-classes).
Execution of Java Constructors in Inheritance
Enough knowledge on inheritance is necessary to continue with this article. If you are reading this tutorial, we assume that you have a solid understanding of Java inheritance.
Let’s learn about the execution process of Java constructors while we are extending the parent class (also known as base class or superclass) using the extends
keyword.
Execution of Default Java Constructor in Inheritance
Example code:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass();
}
}
Output:
Default constructor of the parent class.
Default constructor of the child class.
Default constructor of the grand child class.
Here, we have one test class named constructorsInInheritance
, which creates the object of grandChildClass
.
We have three other classes named parentClass
, childClass
, grandChildClass
, where the grandChildClass
inherits the childClass
and childClass
extends the parentClass
.
Here, the parentClass
default constructor is automatically called by a childClass
constructor. Whenever we instantiate the child class, the parent class’ constructor gets executed automatically, followed by the child class’ constructor.
Observe the output given above. If still confused, see the following visual explanation.
What if we create an object of the childClass
in the main
method? How will the default constructors get executed now?
The constructor of the parentClass
will be executed first, and then the constructor of the childClass
will produce the following result.
Output:
Default constructor of the parent class.
Default constructor of the child class.
Execution of Java Constructor in Inheritance When Parent Class Has Default & Parameterized Constructor
Example code:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass();
}
}
Output:
Default constructor of the parent class.
Default constructor of the child class.
Default constructor of the grand child class.
Here, we have a parameterized constructor in the parentClass
. However, the default constructors are still called because we invoke the grandChildClass()
default constructor in the main
method, further calling the parent class default constructor.
Let’s also write the parameterized constructors in the childClass
and the grandChildClass
. Then, call the parameterized constructor of the grandChildClass
in the main
function.
Observe which constructors are called, whether they are default or parameterized.
Example code:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
childClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the child class");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
grandChildClass(String name) {
System.out.println(
"Hi " + name + "! It's a parameterized constructor of the grand child class");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass("Mehvish");
}
}
Output:
Default constructor of the parent class.
Default constructor of the child class.
Hi Mehvish! It's a parameterized constructor of the grand child class
The code above only calls the parameterized constructor of the grandChildClass
. We use the super()
to call the parameterized constructor of the parentClass
, childClass
, and the grandChildClass
.
Remember, the parent class constructor call must be on the first line in the child class’s constructor.
Use super
to Call the Parameterized Constructor of Parent Class and All Child Classes
Example code:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
childClass(String name) {
super(name);
System.out.println("Hi " + name + "! It's a parameterized constructor of the child class");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
grandChildClass(String name) {
super(name);
System.out.println(
"Hi " + name + "! It's a parameterized constructor of the grand child class");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass("Mehvish");
}
}
Output:
Hi Mehvish! It's a parameterized constructor of the parent class
Hi Mehvish! It's a parameterized constructor of the child class
Hi Mehvish! It's a parameterized constructor of the grand child class
We used the super
keyword to call the parameterized parent class constructor. It refers to the parent class (superclass or base class).
We used it to access the parent class constructor and called the parent class methods.
super
is very useful for methods with the exact names in parent and child classes.