Inheritance on an Enum in Java
-
enums
in Java -
Challenges in
enum
Inheritance in Java -
Methods to Achieve
Enum
Inheritance in Java - Conclusion
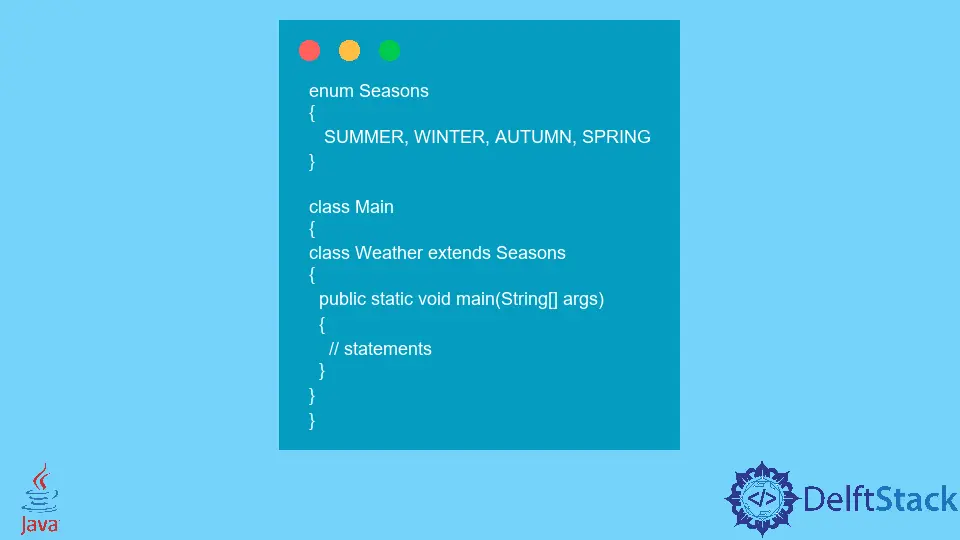
Explore the challenges and methods associated with implementing enum
inheritance in Java, understanding how to overcome limitations and employ effective techniques for enhanced code organization and maintainability.
It is a common practice to represent enum
values in uppercase. In Java, all the enum
classes are final
by default.
So, we cannot inherit or derive different classes from it.
enums
in Java
In Java, an enum
, short for enumeration, is a special data type that represents a fixed set of constants. It provides a way to define a collection of related values, often used to represent categories, options, or states.
They bring clarity to the code by encapsulating related constants within a named group, improving code maintainability, and promoting a more structured and expressive coding style. Overall, enums
in Java simplify the process of working with a predefined set of values and contribute to the creation of robust and understandable code.
Challenges in enum
Inheritance in Java
Enum
inheritance in Java is challenging as enums
cannot directly extend classes, limiting traditional inheritance. While enums
implicitly extend java.lang.Enum
, they cannot extend other classes.
This constraint restricts the ability to share behavior among enums
, requiring alternative approaches like interfaces or composition to emulate inheritance. Thus, the absence of direct class inheritance complicates the organization and reuse of code in enum
structures.
Methods to Achieve Enum
Inheritance in Java
Inheritance on Enums
Using Interface in Java
By using interfaces in conjunction with enums
, we can emulate inheritance, providing a means to share common functionality across enum
constants. Using interfaces in implementing inheritance on enums
in Java provides a clean and flexible approach.
Interfaces allow enums
to share common behavior without the constraints of class inheritance. This method promotes code organization, enhances readability, and accommodates diverse behaviors across enum
constants, offering a more versatile and maintainable solution compared to other methods.
In the realm of Java programming, enums
stand as powerful tools for representing a fixed set of constants. However, when it comes to extending or inheriting behavior among enums
, Java doesn’t directly support class inheritance.
Code Example:
public interface Animal {
void makeSound();
}
enum Dog implements Animal {
BARK;
@Override
public void makeSound() {
System.out.println("Woof!");
}
}
enum Cat implements Animal {
MEOW;
@Override
public void makeSound() {
System.out.println("Meow!");
}
}
public class EnumInheritanceExample {
public static void main(String[] args) {
Dog.BARK.makeSound(); // Outputs: Woof!
Cat.MEOW.makeSound(); // Outputs: Meow!
}
}
In this code snippet, we’re using interfaces to simulate inheritance on enums
. First, we define an interface called Animal
with a method makeSound()
, which our enum
constants will implement for shared behavior.
Next, we declare two enums
, Dog
and Cat
, both implementing the Animal
interface. Each enum
constant provides a unique implementation of the makeSound()
method, representing the distinctive sound of the associated animal.
In the EnumInheritanceExample
class, we showcase invoking the makeSound()
method on different enum
constants, demonstrating the ability to consistently use the shared interface despite the enums
having different implementations.
When we run the main method, the output will be:
This output showcases the successful emulation of inheritance on enums
using interfaces. Despite the limitations of direct enum
inheritance in Java, this approach provides a flexible and clean way to share behavior among enum
constants.
Employing interfaces with enums
allows us to overcome the absence of direct inheritance for enum
constants in Java. This technique enhances code organization, promotes consistency, and enables the creation of more expressive and maintainable codebases.
Inheritance on Enums
Using EnumMap
in Java
EnumMap
is crucial for implementing inheritance on enums
in Java as it efficiently associates specific data with enum
constants. This method enhances code organization, ensures type safety, and provides a streamlined way to manage constant-specific information.
Compared to other methods, EnumMap
offers a clean and organized approach, making it a powerful tool for implementing inheritance on enums
in Java.
Code Example
import java.util.EnumMap;
enum AnimalType { DOG, CAT }
class Animal {
String sound;
public Animal(String sound) {
this.sound = sound;
}
public String getSound() {
return sound;
}
}
public class EnumMapInheritanceExample {
public static void main(String[] args) {
EnumMap<AnimalType, Animal> animalMap = new EnumMap<>(AnimalType.class);
animalMap.put(AnimalType.DOG, new Animal("Woof!"));
animalMap.put(AnimalType.CAT, new Animal("Meow!"));
Animal dog = animalMap.get(AnimalType.DOG);
Animal cat = animalMap.get(AnimalType.CAT);
System.out.println("Dog says " + dog.getSound()); // Outputs: Dog says: Woof!
System.out.println("Cat says " + cat.getSound()); // Outputs: Cat says: Meow!
}
}
In the given example, we employ the EnumMap
to link distinct data with each enum
constant in an orderly fashion. To begin, we define an enum
called AnimalType
to represent various types of animals.
Following that, we introduce a straightforward Animal
class designed to store data unique to each animal type. In the EnumMapInheritanceExample
class, we utilize an EnumMap
named animalMap
to connect each AnimalType
with a corresponding instance of the Animal
class, encapsulating specific data for each enum
constant.
When we run the main method, the output will be:
The EnumMap
in Java proves to be a valuable tool for implementing inheritance-like behavior on enums
. By associating enum
constants with specific data, this approach enhances code organization and maintainability.
The example demonstrates a structured way to handle constant-specific information, allowing for clean and efficient management of data associated with each enum
constant.
Inheritance on Enums
Using Enum
With Fields and Methods in Java
Fields and methods in enums
provide a structured way to implement inheritance in Java, enabling constants to encapsulate behavior and data. This approach enhances code readability, promotes maintainability, and supports a versatile representation of constant-specific functionalities.
Compared to other methods, using fields and methods in enums
ensures a cohesive and expressive design, making it a powerful technique for implementing inheritance in Java enums
.
Code Example
enum MathOperation {
ADD {
@Override
public int apply(int x, int y) {
return x + y;
}
},
SUBTRACT {
@Override
public int apply(int x, int y) {
return x - y;
}
};
public abstract int apply(int x, int y);
}
public class EnumWithMethodsExample {
public static void main(String[] args) {
int resultAdd = MathOperation.ADD.apply(5, 3);
int resultSubtract = MathOperation.SUBTRACT.apply(8, 3);
System.out.println(
"Result of ADD operation= " + resultAdd); // Outputs: Result of ADD operation: 8
System.out.println("Result of SUBTRACT operation= "
+ resultSubtract); // Outputs: Result of SUBTRACT operation: 5
}
}
In this illustration, we delve into the concept of enums
enriched with fields and methods. Our enum
, named MathOperation
, introduces two constants—ADD
and SUBTRACT
.
Each constant features an abstract method named apply(int x, int y)
, serving as a foundational template for operations. In the EnumWithMethodsExample
class, we exhibit the invocation of the apply
method on both enum
constants (ADD
and SUBTRACT
).
This exemplifies how enums
can encapsulate behavior, transforming them into functional units that can be seamlessly utilized.
When we run the main method, the output will be:
This output illustrates the effectiveness of using enums
with fields and methods. Each enum
constant becomes a self-contained unit with specific behavior, providing a clean and expressive way to represent and utilize constant-specific functionalities.
Conclusion
Exploring enum
inheritance in Java reveals both challenges and effective methods. While direct class inheritance is limited, interfaces, abstract classes, EnumMap
, and fields/methods in enums offer versatile solutions.
Interfaces provide flexibility, EnumMap
ensures efficient data association and fields/methods allow encapsulation of behavior. These methods enhance code organization, readability, and maintainability.
Choosing the right approach depends on the specific requirements, emphasizing the importance of understanding and leveraging these techniques to achieve effective enum
inheritance in Java.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn