Multiple Inheritance in Java
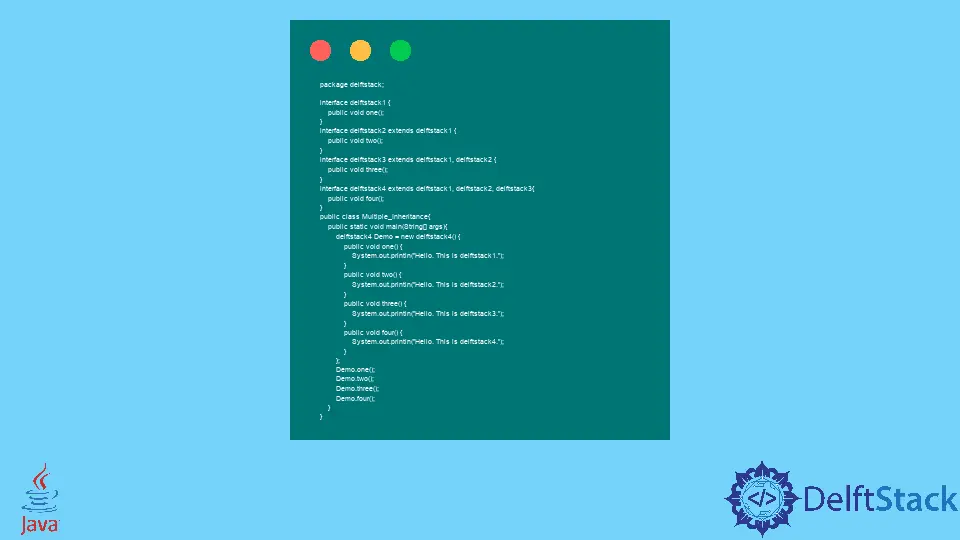
Multiple Inheritance means when a class is a child class to numerous classes, and Java doesn’t allow that. But we can use an interface instead to achieve the same purpose.
This tutorial will demonstrate how to achieve multiple inheritances in Java.
Use Interfaces to Achieve Multiple Inheritance in Java
Java doesn’t support multiple inheritances in classes to avoid ambiguity. But the same purpose can be achieved using interfaces.
package delftstack;
interface delftstack1 {
public void one();
}
interface delftstack2 extends delftstack1 {
public void two();
}
interface delftstack3 extends delftstack1, delftstack2 {
public void three();
}
interface delftstack4 extends delftstack1, delftstack2, delftstack3 {
public void four();
}
public class Multiple_Inheritance {
public static void main(String[] args) {
delftstack4 Demo = new delftstack4() {
public void one() {
System.out.println("Hello. This is delftstack1.");
}
public void two() {
System.out.println("Hello. This is delftstack2.");
}
public void three() {
System.out.println("Hello. This is delftstack3.");
}
public void four() {
System.out.println("Hello. This is delftstack4.");
}
};
Demo.one();
Demo.two();
Demo.three();
Demo.four();
}
}
The code above contains four interfaces from which delftstack2
inherits one interface, delftstack1
, delftstack3
, and delftstack4
inherit two, three interfaces, respectively. These interfaces perform the same functionality of class in multiple inheritances.
Output:
Hello. This is delftstack1.
Hello. This is delftstack2.
Hello. This is delftstack3.
Hello. This is delftstack4.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook