在继承中执行 Java 构造函数
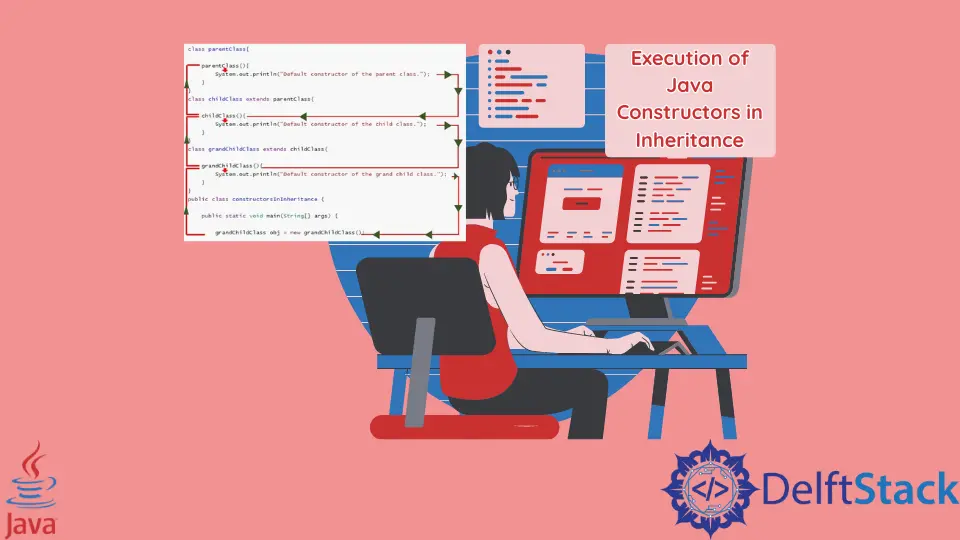
今天,我们将学习继承中 Java 构造函数的执行。我们将在派生类(也称为子类和子类)中看到默认构造函数和参数化构造函数的代码示例。
在继承中执行 Java 构造函数
继续这篇文章需要足够的继承知识。如果你正在阅读本教程,我们假设你对 Java 继承有深入的了解。
让我们在使用 extends
关键字扩展父类(也称为基类或超类)时了解 Java 构造函数的执行过程。
在继承中执行默认 Java 构造函数
示例代码:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass();
}
}
输出:
Default constructor of the parent class.
Default constructor of the child class.
Default constructor of the grand child class.
在这里,我们有一个名为 constructorsInInheritance
的测试类,它创建 grandChildClass
的对象。
我们还有另外三个名为 parentClass
、childClass
、grandChildClass
的类,其中 grandChildClass
继承 childClass
而 childClass
扩展了 parentClass
。
这里,parentClass
默认构造函数由 childClass
构造函数自动调用。每当我们实例化子类时,都会自动执行父类的构造函数,然后是子类的构造函数。
观察上面给出的输出。如果仍然感到困惑,请参阅以下视觉说明。
如果我们在 main
方法中创建 childClass
的对象会怎样?现在将如何执行默认构造函数?
parentClass
的构造函数将首先执行,然后 childClass
的构造函数将产生以下结果。
输出:
Default constructor of the parent class.
Default constructor of the child class.
当父类具有默认和参数化构造函数时在继承中执行 Java 构造函数
示例代码:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass();
}
}
输出:
Default constructor of the parent class.
Default constructor of the child class.
Default constructor of the grand child class.
在这里,我们在 parentClass
中有一个参数化的构造函数。但是,仍然调用默认构造函数,因为我们在 main
方法中调用 grandChildClass()
默认构造函数,进一步调用父类默认构造函数。
我们还要在 childClass
和 grandChildClass
中编写参数化构造函数。然后,在 main
函数中调用 grandChildClass
的参数化构造函数。
观察调用了哪些构造函数,它们是默认的还是参数化的。
示例代码:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
childClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the child class");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
grandChildClass(String name) {
System.out.println(
"Hi " + name + "! It's a parameterized constructor of the grand child class");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass("Mehvish");
}
}
输出:
Default constructor of the parent class.
Default constructor of the child class.
Hi Mehvish! It's a parameterized constructor of the grand child class
上面的代码只调用了 grandChildClass
的参数化构造函数。我们使用 super()
调用 parentClass
、childClass
和 grandChildClass
的参数化构造函数。
请记住,父类构造函数调用必须在子类构造函数的第一行。
使用 super
调用父类和所有子类的参数化构造函数
示例代码:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
childClass(String name) {
super(name);
System.out.println("Hi " + name + "! It's a parameterized constructor of the child class");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
grandChildClass(String name) {
super(name);
System.out.println(
"Hi " + name + "! It's a parameterized constructor of the grand child class");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass("Mehvish");
}
}
输出:
Hi Mehvish! It's a parameterized constructor of the parent class
Hi Mehvish! It's a parameterized constructor of the child class
Hi Mehvish! It's a parameterized constructor of the grand child class
我们使用 super
关键字来调用参数化的父类构造函数。它指的是父类(超类或基类)。
我们用它来访问父类的构造函数并调用父类的方法。
super
对于在父类和子类中具有确切名称的方法非常有用。