The valueOf Method of Enum Class in Java
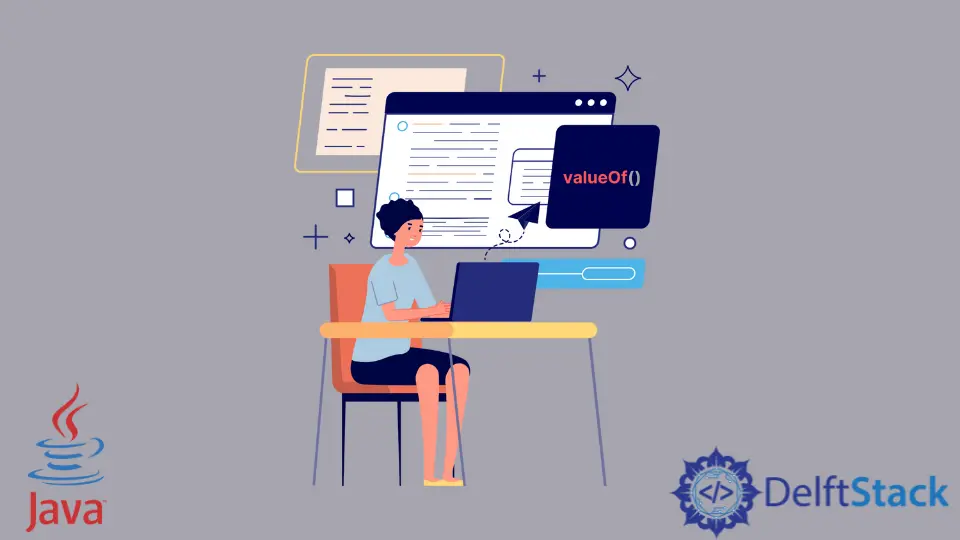
In Java, Enum
is a special data type that enables a variable to be a set of predefined constants.
Common examples include days of the week, directions, colors, etc. As Enums are constants, they are all defined in uppercase letters.
the valueOf Method of Enum Class in Java
In Java, we use the enum
keyword to define an Enum
type to create our data type classes.
Enum
class has the valueOf()
method, which returns the enum constant of the specified enum type with the specified name. The name of the enum type must match the identifier used to declare the enum constant.
The valueOf()
method is case-sensitive, and it will throw an IlegalArgumentException
with an invalid string.
Here we have used Colors
enum. An Enum
class automatically gets a static valueOf
method at the compile time.
The color
variable of the type Colors
is the enum type defined in this program given below. The color
variable can take one of the color enum constants (RED, GREEN, BLUE, WHITE, PINK, YELLOW). In our case, color is set to Colors.BLUE
.
Using the valueOf()
, we have an instance of Enum
class Colors
for a given string value. The colorRed
variable will point to the Colors.RED
. We can see that in the print statement below in output.
public class EnumTest {
enum Colors { RED, GREEN, BLUE, WHITE, PINK, YELLOW }
public static void main(String[] arg) {
Colors color = Colors.BLUE;
Colors colorRed = Colors.valueOf("RED");
System.out.println("color : " + color);
System.out.println("Colors.valueOf(RED) : " + colorRed);
}
}
Output:
color : BLUE
Colors.valueOf(RED) : RED
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn