How to Compare Java Enum Using == or equals() Method in Java
-
Compare Enum Using the
==
Operator in Java -
Compare Enum Using the
equals()
Method in Java -
Compare Enum Values and Handle
null
Safety - Compare Two Different Enum Values in Java
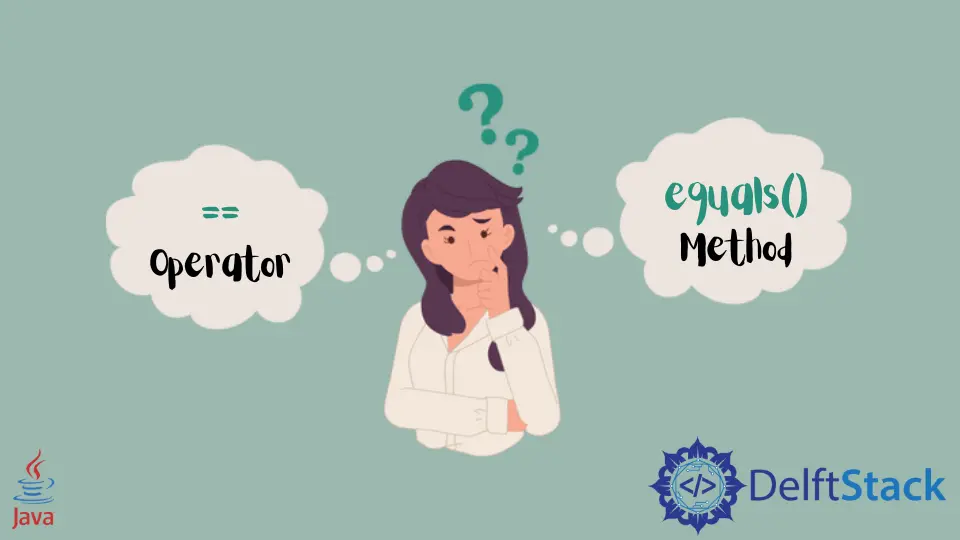
This tutorial introduces how to compare Java enum using the ==
operator or equals()
method in Java.
Enum is a set of constants used to collect data sets such as day, month, color, etc. In Java, to create an enum, we use the enum keyword and then provide values for the type.
This article will demonstrate how to compare the enum values and objects. Let’s understand with some examples.
Compare Enum Using the ==
Operator in Java
The ==
(equal) operator is a binary operator that requires two operands. It compares the operands and returns either true
or false
.
We can use this to compare enum values. See the example below.
enum Color {
red,
green,
yello,
white,
black,
purple,
blue;
}
public class SimpleTesting {
public static void main(String[] args) {
boolean result = isGreen(Color.blue);
System.out.println(result);
result = isGreen(Color.green);
System.out.println(result);
}
public static boolean isGreen(Color color) {
if (color == Color.green) {
return true;
}
return false;
}
}
Output:
false
true
Compare Enum Using the equals()
Method in Java
Java equals()
method compares two values and returns a boolean value, either true
or false
. We can use this method to compare enum values.
Here, we used the Color
enum to compare its values. The first value returns false
, but it returns true
for the second. See the example below.
enum Color {
red,
green,
yello,
white,
black,
purple,
blue;
}
public class SimpleTesting {
public static void main(String[] args) {
boolean result = isGreen(Color.blue);
System.out.println(result);
result = isGreen(Color.green);
System.out.println(result);
}
public static boolean isGreen(Color color) {
if (color.equals(Color.green)) {
return true;
}
return false;
}
}
Output:
false
true
Compare Enum Values and Handle null
Safety
In Java, the most problematic issue is handling null
values. It also applies to the enum comparison; if we use the equals()
method to compare enum values and the enum object is null
, it throws nullpointerexception
. See the example below.
enum Color {
red,
green,
yello,
white,
black,
purple,
blue;
}
public class SimpleTesting {
public static void main(String[] args) {
Color color = null;
boolean result = isGreen(color);
System.out.println(result);
result = isGreen(Color.green);
System.out.println(result);
}
public static boolean isGreen(Color color) {
if (color.equals(Color.green)) {
return true;
}
return false;
}
}
Output:
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "javaexample.Color.equals(Object)" because "color" is null
However, if we work with the ==
(equals) operator and compare enum values/objects, it will not throw the nullpointerexception
. That means this operator is null
safe and better to use than the equals()
method. See the example below.
enum Color {
red,
green,
yello,
white,
black,
purple,
blue;
}
public class SimpleTesting {
public static void main(String[] args) {
Color color = null;
boolean result = isGreen(color);
System.out.println(result);
result = isGreen(Color.green);
System.out.println(result);
}
public static boolean isGreen(Color color) {
if (color == Color.green) {
return true;
}
return false;
}
}
Output:
false
true
Compare Two Different Enum Values in Java
We can also compare two enum objects using the equals()
method. Since both the objects are different, it returns false
in both cases. See the example below.
enum Color {
red,
green,
yello,
white,
black,
purple,
blue;
}
enum MyColors {
green,
red,
blue;
}
public class SimpleTesting {
public static void main(String[] args) {
boolean result = isGreen(Color.red);
System.out.println(result);
result = isGreen(Color.green);
System.out.println(result);
}
public static boolean isGreen(Color color) {
if (color.equals(MyColors.red)) {
return true;
}
return false;
}
}
Output:
false
false