How to Fix the Error: Else Without if in Java
-
the
error: 'else' without 'if'
in Java -
Reasons for the
error: 'else' without 'if'
in Java -
Fix the
error: 'else' without 'if'
in Java - Conclusion
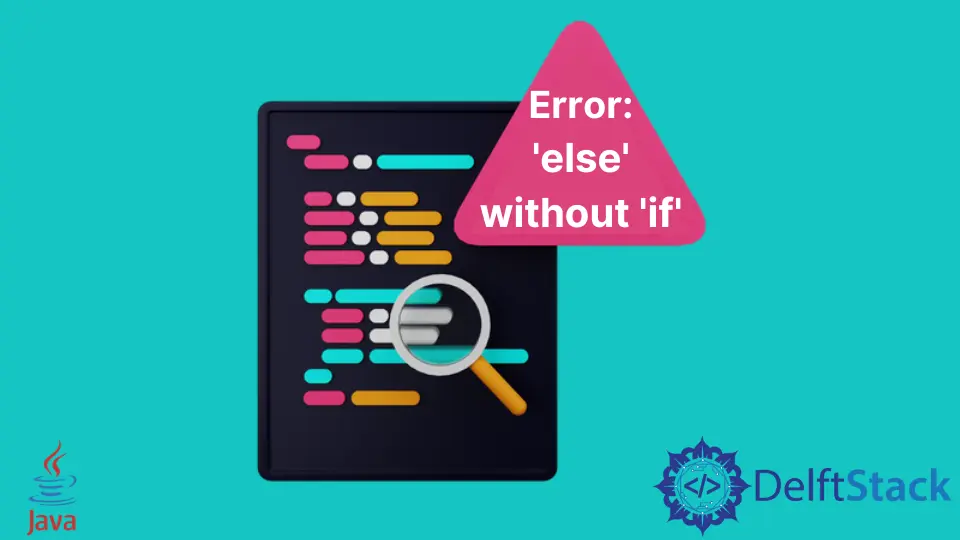
Today, we will learn about an error saying 'else' without 'if'
while writing code in Java. We will also figure out the possible reasons causing this error and find its solution.
the error: 'else' without 'if'
in Java
Usually, the error: 'else' without 'if' in Java
is faced by newbies in Java programming. Before moving toward the causes and solution for this error, let’s write a program that produces this error and understand it.
So, assuming that we are Python experts and beginners in Java. So, we will write the Java program containing if-else
as follows.
Example Code:
import java.util.Scanner;
class Test {
public static void main(String[] args) {
int temp;
Scanner scan = new Scanner(System.in);
System.out.println("What's the current temperature?");
temp = scan.nextInt();
if (temp > 95 || temp < 20)
;
System.out.println("Visit our shops");
else if (temp <= 95) if (temp >= 80) System.out.println("Swimming");
else if (temp >= 60) if (temp <= 80) System.out.println("Tennis");
else if (temp >= 40) if (temp < 60) System.out.println("Golf");
else if (temp < 40) if (temp >= 20) System.out.println("Skiing");
}
}
Output:
In this program, we get the current temperature from the user and decide our future activity based on the current temperature. The image above shows that we are getting a logical error about which NetBeans IDE informs at compile time.
So, we cannot even execute the code until we resolve the error. For that, we will have to know the possible reasons below.
Reasons for the error: 'else' without 'if'
in Java
The error itself is self-explanatory, which says that a Java compiler cannot find an if
statement associated with the else
statement. Remember that the else
statement does not execute unless they’re associated with an if
statement.
So, the possible reasons are listed below.
-
The first reason is that we forgot to write the
if
block before theelse
block. -
The closing bracket of the
if
condition is missing. -
We end the
if
statement by using a semi-colon.
How to solve this error? Let’s have a look at the following section.
Fix the error: 'else' without 'if'
in Java
Example Code:
import java.util.Scanner;
class Test {
public static void main(String[] args) {
int temp;
Scanner scan = new Scanner(System.in);
System.out.println("What's the current temperature?");
temp = scan.nextInt();
if (temp > 95 || temp < 20) {
System.out.println("Visit our shops");
} else if (temp <= 95) {
if (temp >= 80) {
System.out.println("Swimming");
} else if (temp >= 60) {
if (temp <= 80) {
System.out.println("Tennis");
} else if (temp >= 40) {
if (temp < 60) {
System.out.println("Golf");
} else if (temp < 40) {
if (temp >= 20) {
System.out.println("Sking");
}
}
}
}
}
}
}
In this Java code, we begin by obtaining the current temperature from the user through the Scanner
class. The program then evaluates the temperature using a series of nested if-else
statements to determine suitable activities based on the temperature range.
If the temperature (temp
) is either above 95
or below 20
, the program advises the user to "Visit our shops"
; if the temperature is less than or equal to 95
, the program further checks specific temperature ranges.
If the temperature is 80
or above, it suggests "Swimming"
. For temperatures between 60
and 80
, it recommends "Tennis"
.
In the range of 40
to 60
, it suggests "Golf"
. Finally, if the temperature is below 40
but equal to or above 20
, the program suggests "Skiing"
.
The code structure ensures that each temperature range is considered, and the appropriate activity is recommended based on the given temperature input.
Output:
What's the current temperature?
96
Visit our shops
To fix the code, we removed the semi-colon (;
) from the end of the if
statement and placed the {}
for each block to fix an error saying 'else' without 'if'
.
It is better to use curly brackets {}
until we are expert enough and know where we can omit them (we can omit them when we have a single statement in the block).
Conclusion
In conclusion, the article addresses the common Java error 'else' without 'if'
often encountered by beginners. The provided code example demonstrates the error, indicating a logical issue that prevents successful compilation and execution.
The article outlines the reasons behind the error, emphasizing common mistakes such as forgetting the if
block, missing the closing bracket (}
) of the if
condition, or erroneously ending the if
statement with a semi-colon (;
). In order to resolve the error, the article presents a corrected version of the code, emphasizing the importance of using curly braces {}
to properly structure if-else
statements, especially for beginners.
The revised code ensures that each temperature range is considered, and the appropriate activity is recommended based on the given temperature input. Overall, the article provides valuable insights for newcomers to Java programming, helping them understand, identify, and fix the 'else' without 'if'
error in their code.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack