How to Draw a Circle in Java
-
Draw a Circle Using the
drawOval()
Function in Java -
Draw a Circle Using the
drawRoundRect()
Function in Java -
Draw a Circle Using
Shape
anddraw()
in Java
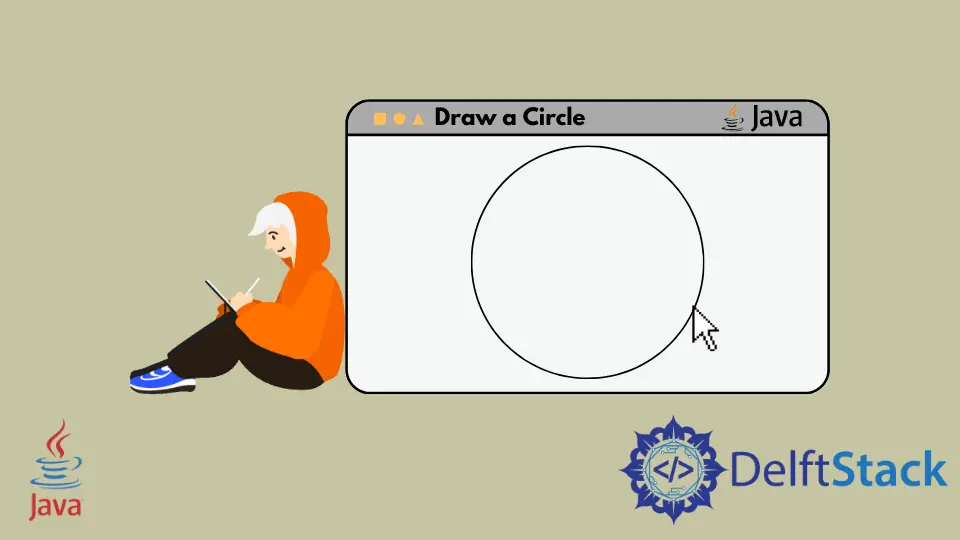
In this article, we will introduce how we can draw a circle in Java using libraries java.awt
and javax.swing
that are used to create GUI components. We will use several functions of the Graphics2d
class that provides more control over the graphical components that we want to draw on the screen.
Draw a Circle Using the drawOval()
Function in Java
In the first example, we use the method drawOval()
of the Graphics2D
class that could create oval shapes, but to create a perfect circle. To draw the circle, we first override the function paint(Graphics g)
that has paints the component using the Graphics
class.
We cast Graphics
to Graphics2D
type to use its methods and properties. Now we call the drawOval()
function and pass four arguments. The first two arguments are the x and y coordinates of the circle, while the last two arguments specify the width and the height of the circle to be drawn.
At last, we extend JFrame
from the javax.swing
package to create a window frame with the specified size and properties.
import java.awt.*;
import javax.swing.*;
public class DrawCircle extends JFrame {
public DrawCircle() {
setTitle("Drawing a Circle");
setSize(400, 400);
setVisible(true);
setDefaultCloseOperation(EXIT_ON_CLOSE);
}
@Override
public void paint(Graphics g) {
Graphics2D g2d = (Graphics2D) g;
g2d.drawOval(150, 150, 100, 100);
}
public static void main(String[] args) {
new DrawCircle();
}
}
Output:
Draw a Circle Using the drawRoundRect()
Function in Java
Another method of the Graphics2D
class called drawRoundRect()
can also be used to draw a circle. As its name suggests, it paints a rounded rectangle. Just like the first example, we override the paint(Graphics g)
function and then call the drawRoundRect()
method of the Graphics2D
class.
drawRoundRect()
accepts six arguments; the first two are x and y coordinates, the next two tell the width and the height, and the last two arguments are the width and height of the arc.
import java.awt.*;
import javax.swing.*;
public class DrawCircle extends JFrame {
public DrawCircle() {
setTitle("Drawing a Circle");
setSize(250, 250);
setVisible(true);
setDefaultCloseOperation(EXIT_ON_CLOSE);
}
@Override
public void paint(Graphics g) {
Graphics2D g2d = (Graphics2D) g;
g2d.drawRoundRect(40, 50, 90, 90, 200, 200);
}
public static void main(String[] args) {
new DrawCircle();
}
}
Output:
Draw a Circle Using Shape
and draw()
in Java
draw()
is another function of the graphics2D
class that takes a Shape
as an argument. Shape
is an interface that provides the properties of a geometrical shape. We want a circle here, so we will use Ellipse2D.Double()
that defines the height and width of the ellipse as well as the x and y coordinates of the framing rectangle.
In the below example, we pass the arguments to Ellipse2D.Double()
, and a created Shape
object to the draw()
method. This method will finally draw a circle in the window that we created using JFrame
.
import java.awt.*;
import java.awt.geom.Ellipse2D;
import javax.swing.*;
public class DrawCircle extends JFrame {
public DrawCircle() {
setTitle("Drawing a Circle");
setSize(250, 250);
setVisible(true);
setDefaultCloseOperation(EXIT_ON_CLOSE);
}
@Override
public void paint(Graphics g) {
Graphics2D g2d = (Graphics2D) g;
Shape circleShape = new Ellipse2D.Double(100, 100, 100, 100);
g2d.draw(circleShape);
}
public static void main(String[] args) {
new DrawCircle();
}
}
Output:
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn