Java Default Parameters
- Default Parameters in Java
- Set Default Parameters using var-args in Java
- Set Default Parameters as Empty String in Java
- Set Default Parameters using var-args with any number of arguments in Java
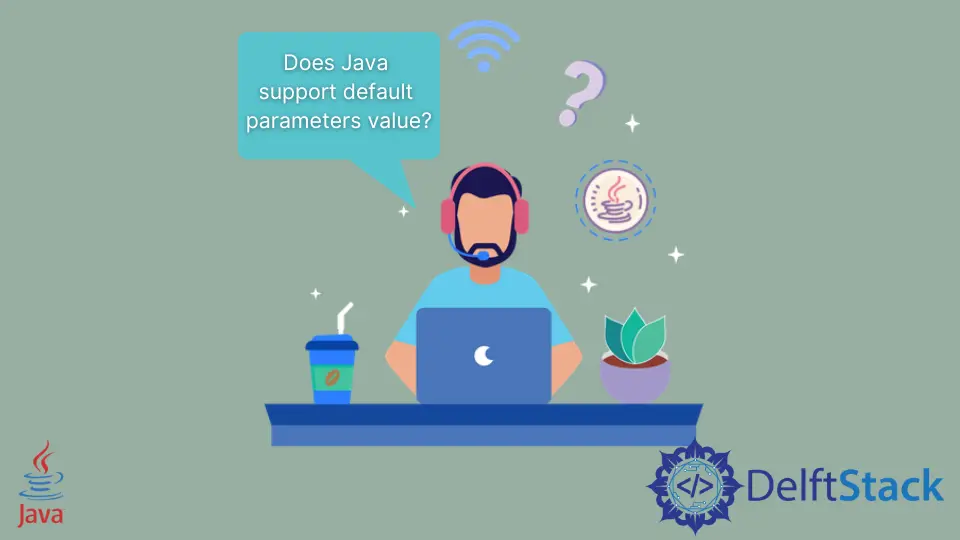
This tutorial introduces how to implement default parameters in Java.
A default parameter is used when no value is passed. It is helpful when we want to pass limited arguments while the method accepts multiple arguments. For example, a method accepts three arguments, but if we wish to pass only two arguments during the method call, then the Java compiler uses the default value of the third argument to avoid any compilation error.
Java does not support default parameters value, but we can achieve it using some built-in solutions such as var-args or method overloading. Let’s see some examples.
Default Parameters in Java
In this example, we use the method overloading approach to set the default parameter value. However, this is not a fine solution but can be used as an alternative. Notice, we pass 0 as the default value while calling the add()
method.
This approach doesn’t work if we have two optional parameters of the same type, and any of them can be omitted.
public class SimpleTesting {
int add(int a, int b) {
return a + b;
}
int add(int a, int b, int c) {
return a + b + c;
}
public static void main(String[] args) {
SimpleTesting test = new SimpleTesting();
int val1 = 10;
int val2 = 20;
int result1 = test.add(val1, 0);
int result2 = test.add(val1, 0, val2);
System.out.println("resutl1 : " + result1);
System.out.println("resutl2 : " + result2);
}
}
Output:
resutl1 : 10
resutl2 : 30
Set Default Parameters using var-args in Java
This is another approach where we use the variable args feature to set default parameters. The var-args
allows passing a variable length of arguments of the same type. See the example below.
public class SimpleTesting {
int add(int a, int... b) {
int b2 = b.length > 0 ? b[0] : 0;
return a + b2;
}
int add(int a, int b, int c) {
return a + b + c;
}
public static void main(String[] args) {
SimpleTesting test = new SimpleTesting();
int val1 = 10;
int val2 = 20;
int result1 = test.add(val1);
int result2 = test.add(val1, 0, val2);
System.out.println("resutl1 : " + result1);
System.out.println("resutl2 : " + result2);
}
}
Output:
resutl1 : 10
resutl2 : 30
Set Default Parameters as Empty String in Java
In the case of string parameters, we can set an empty string to the parameters; however, this string holds null as the default value. See the example below.
public class SimpleTesting {
String defaulPara(int a, String str1, String str2) {
return str1 + str2 + a;
}
public static void main(String[] args) {
SimpleTesting test = new SimpleTesting();
int val1 = 10;
String result1 = test.defaulPara(val1, "", "second");
String result2 = test.defaulPara(val1, "first", "");
System.out.println("resutl1 : " + result1);
System.out.println("resutl2 : " + result2);
}
}
Output:
resutl1 : second10
resutl2 : first10
Set Default Parameters using var-args with any number of arguments in Java
In the case of var-args, we are free to provide any number of arguments while calling the method. So, if you want to provide only limited arguments, then it will work fine. See the example below.
public class SimpleTesting {
int defaulPara(int... a) {
int sum = 0;
for (int i : a) {
sum += i;
}
return sum;
}
public static void main(String[] args) {
SimpleTesting test = new SimpleTesting();
int val1 = 10;
int val2 = 20;
int result1 = test.defaulPara();
int result2 = test.defaulPara(val1);
int result3 = test.defaulPara(val1, val2);
System.out.println("resutl1 : " + result1);
System.out.println("resutl2 : " + result2);
System.out.println("resutl3 : " + result3);
}
}
Output:
resutl1 : 0
resutl2 : 10
resutl3 : 30