How to Create a Bitmap Image in Java
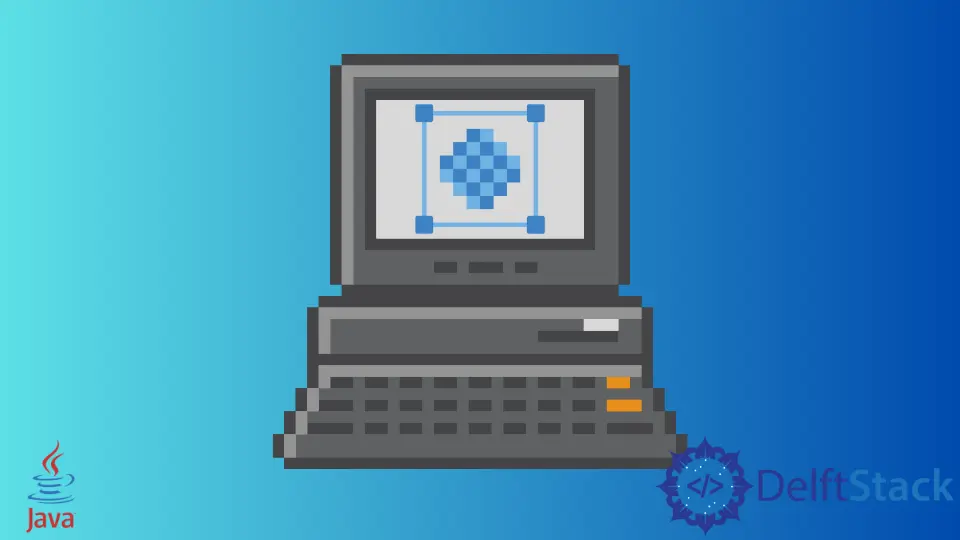
The bitmap is an image file format that stores images as an array of bits organized in a particular fashion to produce the image.
This article discusses how we can create a bitmap image in Java.
Bitmap Image in Java
Java does not have a specific Bitmap class to represent bitmap images.
Instead, it has a BufferedImage
class. This class is a subclass of the Image
class and includes a color model and raster of image data.
You can use the BufferedImage
class to store the image data as pixels. You can invoke the class’s get
and set
methods to manipulate the pixel values to get the desired image.
Types of Bitmap Image in Java
The Java bitmap image or the Java BufferedImage
has several different types. You can set the flag variable representing the type of the image in the constructor itself.
The types differ in the coloring schemes, and you can even produce a grayscale picture. To know more about the flags and methods of the BufferedImage
class, you can visit here.
Use the BufferedImage
Class to Create a Bitmap Image in Java
You can create a bitmap image in Java using the BufferedImage
class and the setRGB()
method.
It provides us with a data buffer and various methods that we can use to manipulate the image data. To create a BufferedImage
, we can use the BufferedImage()
constructor.
The BufferedImage()
constructor takes the image width as its first input argument, the height of the image as its second input argument, and an integer constant representing image type as its third input argument.
The setRGB()
method, when invoked on a BufferedImage
, sets a pixel in the BufferedImage
with a specific RGB value.
The setRGB()
method takes the x-coordinate of the pixel to be manipulated as its first input argument, the y-coordinate of the pixel as its second input argument, and an integer RGB value as its third input argument. We used the red RGB value as the third input argument in our example below.
Let us see the code that produces a bitmap image of size 10x10 and colors it with red.
import java.awt.Color;
import java.awt.image.BufferedImage;
public class ImageExample {
public static void main(String[] args) {
BufferedImage img = new BufferedImage(10, 10, BufferedImage.TYPE_4BYTE_ABGR);
img.setRGB(1, 1, Color.RED.getRGB());
System.out.println(img);
}
}
Output:
BufferedImage@50040f0c: type = 6 ColorModel: #pixelBits = 32 numComponents = 4 color space = java.awt.color.ICC_ColorSpace@7a4f0f29 transparency = 3 has alpha = true isAlphaPre = false ByteInterleavedRaster: width = 10 height = 10 #numDataElements 4 dataOff[0] = 3
We created a bitmap image in Java stored in the img
variable in the above example. You can color each pixel by looping through the image.
And the output shown on the console is the metadata of the image.
If you want to see the image, you must save the image in an image format such as png
, jpg
, etc. You can also set the color of your choice to the image by passing the RGB values to the setRGB()
method.