How to Create a 2D ArrayList in Java
- Create 2d ArrayList in Java Using Fixed-Size Array
- Create a 2D ArrayList in Java by Creating ArrayList of ArrayList
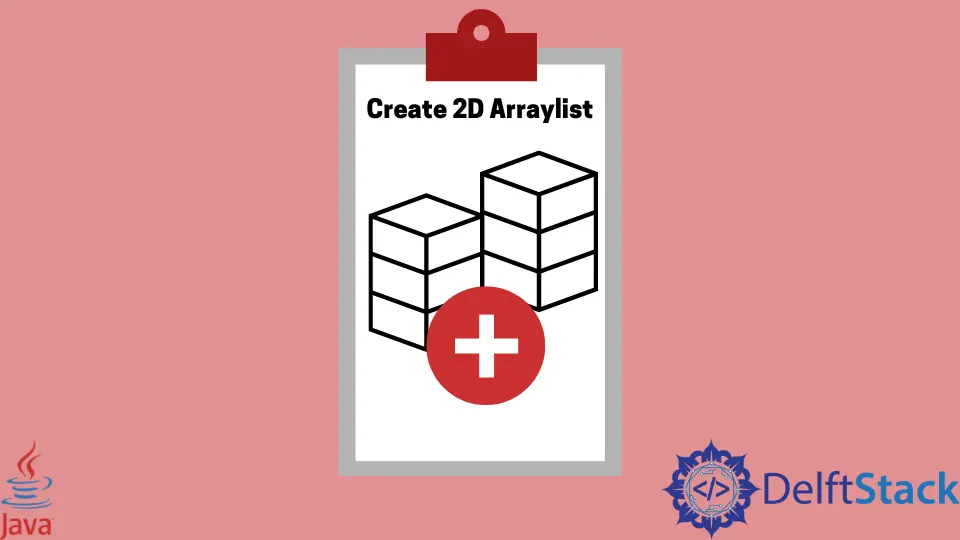
An ArrayList is a dynamic array whose size can be modified, unlike an array with a fixed size. Its flexibility is appreciated the most, but is it flexible enough to create a two-dimensional ArrayList just like a two-dimensional array? Let us find out.
In this tutorial, we will introduce two methods of how you can create a 2D ArrayList Java.
Create 2d ArrayList in Java Using Fixed-Size Array
This first method will create an ArrayList named arraylist1
with a size of three rows and three columns. We want to insert an ArrayList of Strings in arraylist1
; to do this, we will create an ArrayList object in each row and column and add data to it.
The example below shows that arraylist[0][0]
is filled first, which is the first row, and the first column of arraylist1
; this goes on until the ArrayList is completely filled. We are only adding data to the first row here, and the next two rows are null, making the output show null.
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
ArrayList<String>[][] arraylist1 = new ArrayList[3][3];
arraylist1[0][0] = new ArrayList<String>();
arraylist1[0][0].add("String One");
arraylist1[0][0].add("String Two");
arraylist1[0][0].add("String Three");
arraylist1[0][1] = new ArrayList<String>();
arraylist1[0][1].add("String One");
arraylist1[0][1].add("String Two");
arraylist1[0][1].add("String Three");
arraylist1[0][2] = new ArrayList<String>();
arraylist1[0][2].add("String One");
arraylist1[0][2].add("String Two");
arraylist1[0][2].add("String Three");
System.out.println(Arrays.deepToString(arraylist1));
}
}
Output:
[[[String One, String Two, String Three], [String One, String Two, String Three], [String One, String Two, String Three]],
[null, null, null],
[null, null, null]]
Create a 2D ArrayList in Java by Creating ArrayList of ArrayList
The next method to produce a 2D list in Java is to create an ArrayList of ArrayLists; it will serve our purpose as it will be two-dimensional. To insert an innerArraylist
function inside outerArrayList1
, we can initialize the 2D ArrayList Java object to outerArrayList1
.
The next and the last step is adding our data to the innerArraylist
function and then adding it to the outerArrayList
command. Note that we can add more than one ArrayLists into the outerArrayList
command.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
ArrayList<String> innerArraylist;
innerArraylist = new ArrayList<String>();
List<ArrayList<String>> outerArrayList = new ArrayList<>();
innerArraylist.add("String One");
innerArraylist.add("String Two");
innerArraylist.add("String Three");
outerArrayList.add(innerArraylist);
System.out.println(outerArrayList.toString());
}
}
Output:
[[String One, String Two, String Three]]
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn