How to Fix the Could Not Find or Load Main Class Error in Java
- Could Not Find Error Due to Passing the Wrong Name in Java
- Could Not Find Error Due to Wrong Package Name in Java
- Could Not Find Error Due to Wrong CLASSPATH in Java
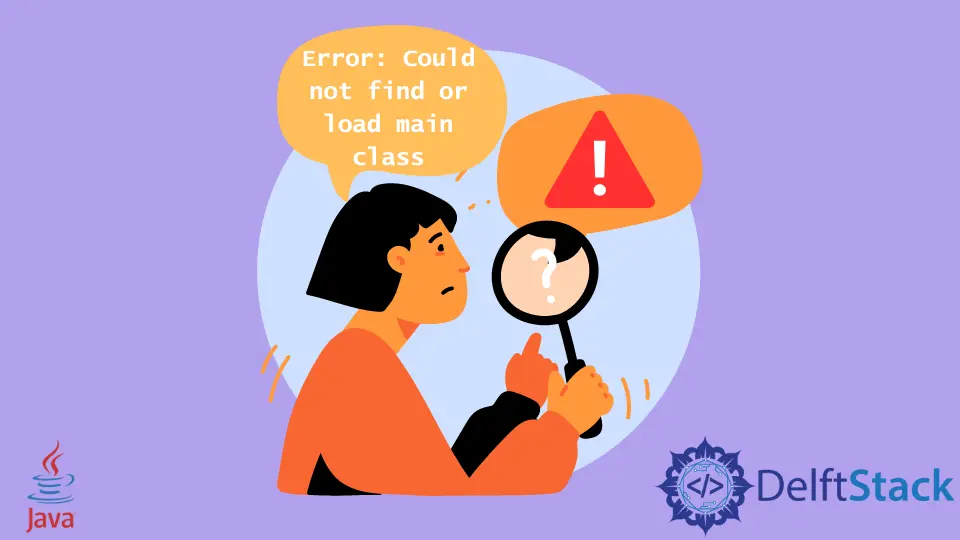
This tutorial introduces the could not find or load main class errors in Java.
Suppose we have written a code and compiled it. Till now, everything is working fine, but when we finally ran it, an error showed up.
could not find or load main class
This tutorial will discuss why this error occurs and how to resolve it. Let us first recap how we run a java program using the command prompt.
First, we compile the code using the javac command like below:
javac<.java file name>
After executing the above command, A file with the .class
extension gets created into the current folder.
The .class
file will have the same class as the .java
program. We then run the .class
file using the following command to execute the Java code:
java<classname>
We may get the could not find or load main class
error. This error is a runtime error and occurs when the Java Virtual machine cannot locate the main class (class containing the main method) we are trying to run.
This error most commonly occurs when running our Java programs using the command prompt. Before discussing the causes of this error, let us first understand CLASSPATH.
CLASSPATH in Java
This is the executable.class
and other resource files.
The JVM uses it to locate the files. The default CLASSPATH is the current directory unless we explicitly set the CLASSPATH in the system variables.
To run a program, we need to pass the class name. We take the following example to illustrate the point:
public class DelftStack {
public static void main(String args[]) {
System.out.println("Hello from DelftStack");
}
}
Let’s first compile it using the javac command:
C :\Users\User\Documents\DelftStack\java
> javac DelftStack.java C :\Users\USer\Documents\DelftStack\java
>
After the above command execution, a DelftStack.class
file gets created in our current directory. Let’s run that file by using the java command.
C :\Users\User\Documents\DelftStack\java
> java DelftStack.class Error : Could not find or load main class DelftStack.
class Caused by : java.lang.ClassNotFoundException : DelftStack.class
Here, we are getting an error because we are trying to run the .class
file. Instead, we just need to pass the class name.
Look below:
C :\Users\User\Documents\DelftStack\java > java DelftStack Hello from DelftStack
Could Not Find Error Due to Passing the Wrong Name in Java
The could not find or load the main class
can also occur when we pass the wrong class name. By continuing the previous example, if we try to run the program with the wrong name as follows:
C :\Users\User\Documents\DelftStack\java > java DelftStac Error
: Could not find or load main class DelftStac Caused by : java.lang.ClassNotFoundException
: DelftStac
We get the error above because we have misspelled the class name. Here, the JVM is trying to run a class named DelftStac
, which doesn’t exist.
We can resolve this issue by correctly spelling out the class name as follows:
C :\Users\User\Documents\DelftStack\java > java DelftStack Hello from DelftStack
We should also note here that the class name is case-sensitive. If we run the class Delftstack
, we will get an error.
Look below:
C :\Users\User\Documents\DelftStack\java > java Delftstack Error
: Could not find or load main class Delftstack Caused by : java.lang.NoClassDefFoundError
: Delftstack(wrong name
: Delftstack)
We should use the correct spelling and the correct cases to run a file successfully.
Could Not Find Error Due to Wrong Package Name in Java
Let’s move our DelftStack
class into the com.DelftStack
package. A package is used to keep similar classes together.
Look at the following code:
package com.DelftStack;
public class DelftStack {
public static void main(String args[]) {
System.out.println("Hello from DelftStack");
}
}
To compile a package in Java, we use the following command:
javac - d.<.java file name>
The -d
flag switch is used to tell where to keep the generated class file. The .
means the current directory.
We compile the above code as follows:
C :\Users\User\Documents\DelftStack\java > javac - d.DelftStack.java
After executing the above command, the following folder structure gets created in our current directory.
com\DelftStack\DelftStack.class
As we can see, our class file is two folders deep from our current directory. So if we try to run our class file like we were doing in previous cases, we get an error.
C :\Users\User\Documents\DelftStack\java > java DelftStack Error
: Could not find or load main class DelftStack Caused by : java.lang.ClassNotFoundException
: DelftStack
The reason for this error is that no DelftStack
class exists in our current folder. To run the class present in a package, we need to pass its fully qualified name (com.DelftStack.DelftStack
in this case).
C :\Users\User\Documents\DelftStack\java > java com.DelftStack.DelftStack Hello from DelftStack
This tells Java to look for the class inside the com\DelftStack
folder.
Could Not Find Error Due to Wrong CLASSPATH in Java
The CLASSPATH tells the JVM where the .class
files are present.
Suppose we are currently in a different folder, and we want to run a Java program whose class file exists in a different folder. In this case, we can pass the location of the class file using the -classpath
option.
For example:
java - classpath XYZ / ABC < class name
>
The above command tells Java to look for the .class
file inside the ZYX/ABC
folder.
In the previous case, we created a package.
Suppose we want to run the file inside the com/DelftStack
folder. Using the following command, we can do so:
> java - classpath../../ com.DelftStack.DelftStack Hello from DelftStack
The ../
means the parent directory. So ../../
means to lookup two directory levels.
Let us take another example, suppose we are at the desktop (folder) location, and we want to run a class file somewhere else on the computer. We can do so by below.
> java - cp C :\Users\User\Documents\DelftStack\java com.DelftStack.DelftStack Hello from DelftStack
The -cp
flag is the shorthand for -classpath
. Here, we passed the full location of the folder where the .class
file is present.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack