How to Copy ArrayList in Java
- Copy ArrayList to Another by Passing It to Another ArrayList’s Constructor
-
Copy ArrayList to Another Using the
addAll()
Fuction -
Copy ArrayList Using Java 8
Stream
-
Copy ArrayList to Another Using the
clone()
Method
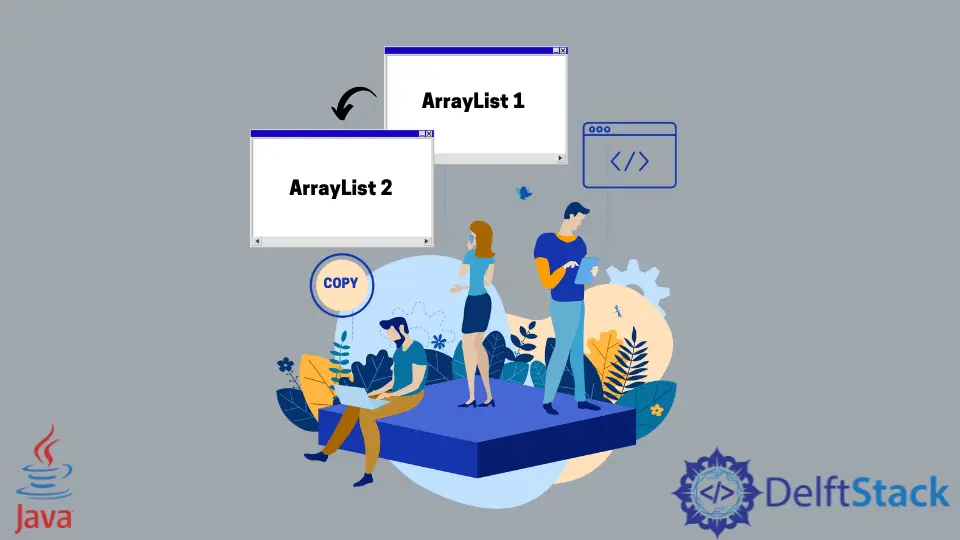
After we have introduced how to copy array in Java in another article, we will introduce four methods to copy an ArrayList to another ArrayList in Java in this article. We will use the same elements in every example to copy an ArrayList using different methods.
Copy ArrayList to Another by Passing It to Another ArrayList’s Constructor
An ArrayList in Java can have three types of constructor. We can create an ArrayList object with an empty constructor, with initial size, or a collection in which the ArrayList is created using the collection’s elements.
We will use the third type of constructor; we first create an ArrayList names1
with an empty constructor and then add some random names. We create a new ArrayList names2
to copy the elements of names1
to it and pass names1
to the constructor new ArrayList<>(names1)
.
At last, we print the whole names2
ArrayList using forEach
that prints each element.
import java.util.ArrayList;
public class CopyArrayList {
public static void main(String[] args) {
ArrayList<String> names1 = new ArrayList<>();
names1.add("Alan");
names1.add("Alex");
names1.add("Bob");
names1.add("Bryan");
names1.add("Cathy");
names1.add("Drake");
ArrayList<String> names2 = new ArrayList<>(names1);
names2.forEach(System.out::println);
}
}
Output:
Alan
Alex
Bob
Bryan
Cathy
Drake
Copy ArrayList to Another Using the addAll()
Fuction
ArrayList comes with a function addAll()
that takes a Collection
as an argument and adds or appends the given collection’s elements at the end of the ArrayList if there are existing elements. ArrayList implements Collection
, which allows us to use the ArrayList names1
as an argument of the addAll()
method.
names1
contains a few elements that should be copied to the newly created empty Arraylist names2
. And it is done by names2.addAll(names1)
. The output shows the copied elements of names2
.
import java.util.ArrayList;
public class CopyArrayList {
public static void main(String[] args) {
ArrayList<String> names1 = new ArrayList<>();
names1.add("Alan");
names1.add("Alex");
names1.add("Bob");
names1.add("Bryan");
names1.add("Cathy");
names1.add("Drake");
ArrayList<String> names2 = new ArrayList<>();
names2.addAll(names1);
names2.forEach(System.out::println);
}
}
Output:
Alan
Alex
Bob
Bryan
Cathy
Drake
Copy ArrayList Using Java 8 Stream
In this example, we use the new Stream API
introduced in Java 8. We create an ArrayList with elements, then call the stream()
method with names1
to use the stream methods like the collect()
method that collects the stream and folds it into a list using Collectors.toList()
.
This stream returns a List, which needs to be cast to an ArrayList.
import java.util.ArrayList;
import java.util.stream.Collectors;
public class CopyArrayList {
public static void main(String[] args) {
ArrayList<String> names1 = new ArrayList<>();
names1.add("Alan");
names1.add("Alex");
names1.add("Bob");
names1.add("Bryan");
names1.add("Cathy");
names1.add("Drake");
ArrayList<String> names2 = (ArrayList<String>) names1.stream().collect(Collectors.toList());
names2.forEach(System.out::println);
}
}
Output:
Alan
Alex
Bob
Bryan
Cathy
Drake
Copy ArrayList to Another Using the clone()
Method
The last method is the clone()
method that is a native ArrayList
method. It copies the elements and returns a new List, similar to the previous solution. We create an ArrayList with elements and call the clone()
method. At last, we cast the returned results to ArrayList<String>
to get our desired result.
import java.util.ArrayList;
public class CopyArrayList {
public static void main(String[] args) {
ArrayList<String> names1 = new ArrayList<>();
names1.add("Alan");
names1.add("Alex");
names1.add("Bob");
names1.add("Bryan");
names1.add("Cathy");
names1.add("Drake");
ArrayList<String> names2 = (ArrayList<String>) names1.clone();
names2.forEach(System.out::println);
}
}
Output:
Alan
Alex
Bob
Bryan
Cathy
Drake
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn