Class Expected Error in Java
-
Introduction to
.class expected
Error in Java -
Why Solve
.class expected
Error? -
Common Errors Leading to the
.class expected
Error -
Best Practices for Avoiding the
.class expected
Error in Java - Conclusion
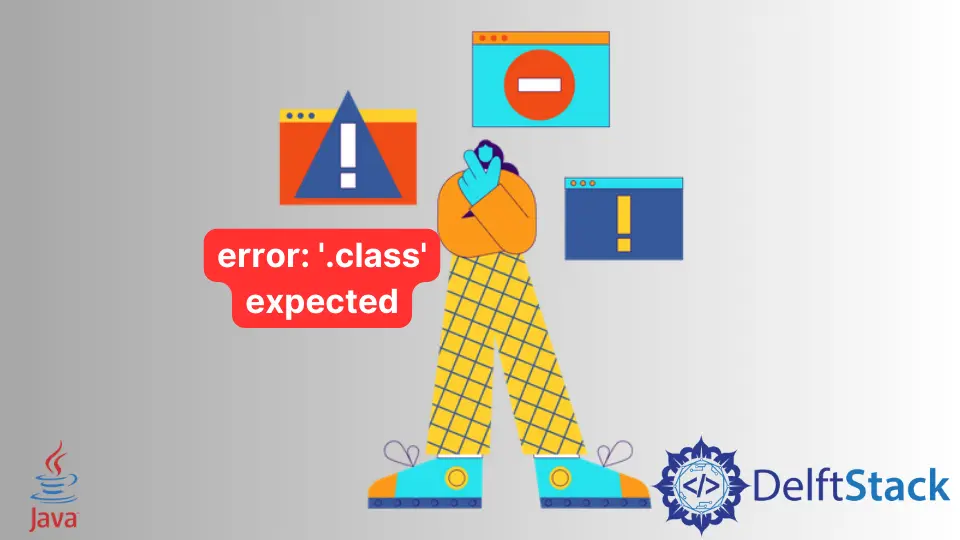
In this guide, we will look into what the .class expected
error in Java.
Navigating the intricate realm of Java programming, the .class expected
error surfaces due to reasons like misplaced brackets or variable type declarations, two common pitfalls made by developers, and can be mitigated through best practices in code organization and syntax adherence.
Introduction to .class expected
Error in Java
The .class expected
error in Java is a compilation error that occurs when the Java compiler encounters a situation where it expects a class-related entity but doesn’t find it. This error usually points to issues such as a missing class declaration, incorrect package declaration, misplaced code outside of a class or method, syntax errors, or incorrect use of keywords.
Essentially, the compiler is signaling that the code structure does not conform to the expected format for a Java program.
This tabular format provides a clear overview of each issue along with example code and a brief description of the problem and solution.
Issue | Example Code | Description |
---|---|---|
Missing Class Declaration | java public class MyClass { // class content } |
This error may occur if you forget to declare a class where it is expected. Every Java program must have at least one class, and the file name should match the class name. |
Incorrect Package Declaration | java package com.example; public class MyClass { // class content } |
If you are using packages, ensure that the package declaration at the beginning of the file matches the directory structure and is correctly formatted. |
Misplaced Code or Statements | java public class MyClass { public static void main(String[] args) { // code here } } |
Placing code outside of a class or method can result in a class expected error. All executable code should be inside a method or a block. |
Incorrect Syntax | java public class MyClass { public static void main(String[] args) { // code here } // Missing semicolon or brace } |
Syntax errors, such as missing semicolons or braces, can lead to a class expected error. |
Incomplete or Incorrect Import Statements | java import java.util.ArrayList; public class MyClass { // class content } |
If you are using external classes, make sure your import statements are correct and complete. |
Incorrect Use of Keywords | java public class MyClass { int class = 10; // "class" is a reserved keyword } |
Using Java keywords incorrectly or inappropriately can lead to compilation errors. |
Incorrect File Name | java // File: MyClass.java public class MyClass { // class content } |
Ensure that the file name matches the name of the public class in the file. Java is case-sensitive, so the names must match exactly. |
Incorrectly Closed Comments or Strings | java public class MyClass { /* Multiline comment without closing } |
Unclosed comments or strings may cause parsing errors. |
In short, a .class
error is an error that occurs if we make a Syntax Error.
Why Solve .class expected
Error?
Solving the .class expected
error in Java is crucial because it directly impacts the compilation and execution of your Java program. In Java, every program must contain at least one class, and this class serves as the entry point for the program’s execution.
If left unresolved, this error prevents the successful compilation of your Java program, meaning it cannot be translated into bytecode that the Java Virtual Machine (JVM) can execute. Consequently, the program won’t run, and any intended functionality or logic within it will remain inaccessible.
Therefore, addressing the .class expected
error is essential to ensure the proper functioning of your Java application.
Common Errors Leading to the .class expected
Error
Extra or Misplaced Curly Braces
The .class expected
error typically surfaces when the Java compiler encounters an unexpected token or construct, signaling that it is expecting a class-related entity. One common scenario leading to this error is the misplacement or addition of curly brackets in the code.
Let’s take a look at a simple example to illustrate this issue.
Consider the following Java code snippet:
public class MyClass {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
} // Extra curly bracket causing ".class expected" error
In this example, an extra closing curly bracket has been added at the end of the class. This seemingly innocent mistake can result in a .class expected
error during compilation.
Upon dissecting the code, we identify the root cause of the .class expected
error: an additional curly bracket (}
) that disrupts the proper structure of the Java code. This surplus bracket interferes with the expected syntax, triggering a compilation error.
To remedy this issue, simply remove the extraneous curly bracket, ensuring the correct alignment and structure of your Java code.
This adjustment brings the code back to its intended form, resolving the .class expected
error and allowing for smooth compilation and execution.
Fixing the Issue
To resolve the .class expected
error, remove the extra curly bracket. The corrected code should look like this:
public class MyClass {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Understanding and resolving the .class expected
error in Java involves meticulous code inspection, particularly for misplaced or extra curly brackets. By adhering to the proper structure and syntax, developers can ensure smooth compilation and execution of their Java programs.
Output:
The ability to diagnose and fix such errors is an essential skill for any Java programmer, contributing to the creation of robust and error-free code.
Function Outside Class
When functions are placed outside of a class in Java, it leads to the .class expected
error. This occurs because Java expects all executable code, including functions, to be encapsulated within a class.
Placing functions outside of a class disrupts the expected structure of Java code, triggering a compilation error. To resolve this issue, it’s essential to ensure that functions are correctly nested within their respective classes, adhering to Java’s syntax and organization principles.
Let’s take a look at a simple example to illustrate this issue.
Consider the following Java code snippet:
public class MyClass {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
// Function misplaced outside the class
public void someFunction() {
System.out.println("This function is outside the class!");
}
In this example, the function someFunction()
is unintentionally placed outside the class, inviting the .class expected
error into our codebase.
Upon analyzing the code, we discern why the .class expected
error emerges when functions find themselves outside a class.
The issue originates from the misplaced function declaration, as exemplified by public void someFunction() {
. This function is incorrectly positioned outside the class to which it should belong.
Consequently, the subsequent line, System.out.println("This function is outside the class!");
encapsulates the body of the function, contributing to a structural anomaly. In Java, functions should be properly nested within their respective classes to adhere to the language’s syntax rules.
Fixing the Issue
To rectify this issue and bid farewell to the .class expected
error, we need to bring the stray function back inside the class where it belongs:
public class MyClass {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
// Function properly placed within the class
public void someFunction() {
System.out.println("This function is now inside the class!");
}
}
Understanding the .class expected
error in the context of misplaced functions outside a class is crucial for Java developers. By ensuring that functions are correctly nested within their respective classes, we maintain the integrity of our code structure and eliminate the possibility of encountering this enigmatic error.
Output:
The ability to diagnose and rectify such issues contributes to the creation of robust and error-free Java programs, ensuring a smooth and error-free development experience.
Best Practices for Avoiding the .class expected
Error in Java
Class Declaration
Always include a valid class declaration in your Java program. Ensure that the class name matches the filename and follows proper Java naming conventions.
Package Declaration
If you are using packages, declare them correctly at the beginning of the file. Verify that the package structure aligns with your directory structure.
Code Placement
Place executable code inside methods or blocks within a class. Avoid placing code directly outside a class or method to prevent compilation errors.
Syntax Accuracy
Double-check for correct syntax, including proper usage of semicolons and braces. Syntax errors can lead to the .class expected
error.
Import Statements
Ensure accurate and complete import statements for external classes. Only import the classes you need to avoid unnecessary clutter and potential conflicts.
Keyword Usage
Use Java keywords appropriately. Avoid using reserved keywords as identifiers to prevent conflicts and compilation errors.
File Naming
Ensure that the filename matches the name of the public class in the file, adhering to Java’s case-sensitive naming convention.
Comment and String Closure
Properly close comments and strings to prevent parsing errors. Unclosed comments or strings can disrupt the compilation process.
By adhering to these best practices, you can minimize the likelihood of encountering the .class expected
error in your Java code. These practices contribute to a clean, well-structured, and error-free codebase, making the development and maintenance processes smoother.
Conclusion
Understanding and addressing the .class expected
error in Java are crucial for maintaining a smooth development process. This article delved into the common reasons behind this error, highlighting two frequent mistakes made by developers: misplaced or extra curly brackets and variable type declarations inside return statements or method calls.
To prevent such errors, it is imperative to adhere to best practices, including proper code organization, syntax adherence, and meticulous attention to class structure. By adopting these practices, developers can fortify their code against the .class expected
error, fostering a more robust and error-free Java programming experience.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack