How to Call REST API in Java
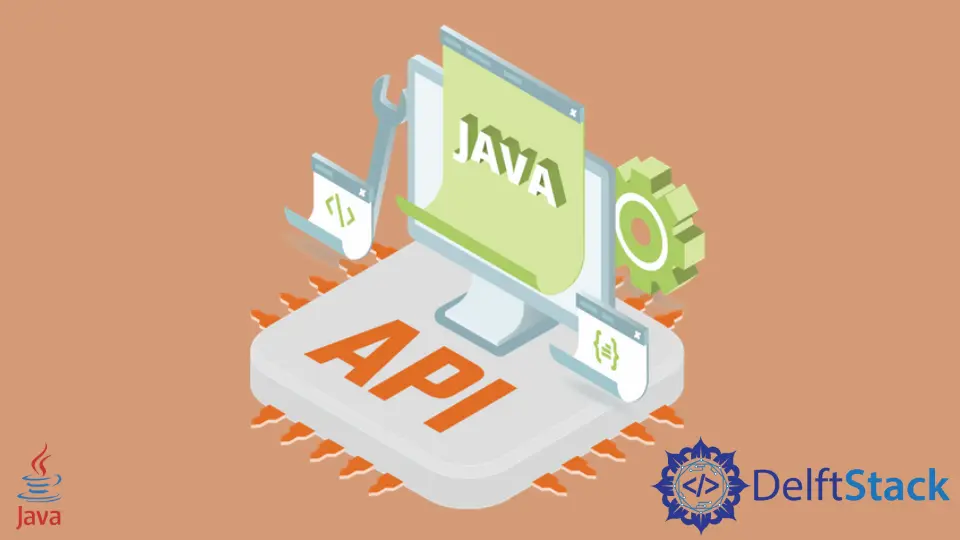
If you are working on a program that allows you to connect with a server, you may be familiar with the REST API. Through this API, you can able to connect with a specific server.
In this article, we will learn about REST API, it’s working & key features, and how we can use GET
and POST
requests to call REST API in Java.
Overview of REST API in Java
The word REST
is defined as Representational State Transfer
. How does it work? In this system, your program is a client that can send a request to the server to get some data.
On the other hand, the server here receives that call and responds to the client. This request and response pair follows a protocol known as the HTTP
or Hyper Text Transfer Protocol
.
Key Features of REST API
Following are the key features of the REST API:
- The design of the REST API is stateless, which means when a client and a server want to connect, they will need a piece of extra information to complete the request.
- The REST API also can cache resources for better performance.
- The REST API uses a uniform interface that enables the client to speak with the server in a specific language.
Use GET
& POST
Requests to Call REST API in Java
The GET
receives a representation of a specified resource while POST
is used for writing data to be processed to an identified resource. Let’s learn how we can use them to call REST API below.
Use GET
Request
Example Code:
// Importing all the necessary packages
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HTTPGet {
public static void main(String args[]) throws Exception {
// Providing the website URL
URL url = new URL("http://example.com");
// Creating an HTTP connection
HttpURLConnection MyConn = (HttpURLConnection) url.openConnection();
// Set the request method to "GET"
MyConn.setRequestMethod("GET");
// Collect the response code
int responseCode = MyConn.getResponseCode();
System.out.println("GET Response Code :: " + responseCode);
if (responseCode == MyConn.HTTP_OK) {
// Create a reader with the input stream reader.
BufferedReader in = new BufferedReader(new InputStreamReader(MyConn.getInputStream()));
String inputLine;
// Create a string buffer
StringBuffer response = new StringBuffer();
// Write each of the input line
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Show the output
System.out.println(response.toString());
} else {
System.out.println("Error found !!!");
}
}
}
In the example above, we illustrated the most used request method in HTTP
, known as GET
. We already wrote the purpose of each line in the example code.
In this example, we created an HTTP
connection based on the provided URL and then defined the requested property. After that, we set the request method to GET
.
Then we created a BufferedReader
with InputStreamReader
to read and save each document line. Lastly, we print the website as an output.
Output:
GET Response Code :: 200
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8">
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
Use POST
Request
Example Code:
// Importing all the necessary packages
import java.io.*;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
public class HTTPPost {
public static void main(String[] args) throws MalformedURLException, IOException {
// Providing the website URL
URL myUrl = new URL("https://example.com");
// Creating an HTTP connection
HttpURLConnection MyConn = (HttpURLConnection) myUrl.openConnection();
// Set the request method to "POST"
MyConn.setRequestMethod("POST");
MyConn.setDoOutput(true);
// Creating an output stream
OutputStream out = MyConn.getOutputStream();
// Defining the sting to post
String postedString = "This is the posted text !!!!!!";
out.write(postedString.getBytes());
// Collect the response code
int responseCode = MyConn.getResponseCode();
System.out.print("Value of http created is:" + MyConn.HTTP_CREATED + "\n");
if (responseCode == MyConn.HTTP_CREATED) {
System.out.print("This is the response Code: " + responseCode + "\n");
System.out.print(
"This is the response Message fromserver: " + MyConn.getResponseMessage() + "\n");
} else {
System.out.print("GO HOME, EVERYBODY :( ");
}
// Creating an input stream reader
InputStreamReader in = new InputStreamReader(MyConn.getInputStream());
// Creating a buffered reader
BufferedReader buffer = new BufferedReader(in);
// Creating a string buffer
StringBuffer fromServer = new StringBuffer();
String eachLine = null;
// Writing each line of the document
while ((eachLine = buffer.readLine()) != null) {
fromServer.append(eachLine);
fromServer.append(System.lineSeparator());
}
buffer.close();
// Printing the html document
System.out.print("Here is our webpage:\n" + fromServer);
}
}
Output:
Value of http created is:201
GO HOME, EVERYBODY :( Here is our webpage:
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8">
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
In the example above, we illustrated another most used request method in HTTP
, known as POST
. In the example, we created an HTTP
connection based on the provided URL and then defined the requested property.
After that, we set the request method to POST
. Then, we set an OutputStream
to post the string; after that, we worked with the response code
.
Next, we created a BufferedReader
with the InputStreamReader
. Then we created a StringBuffer
to hold all the documents. Hopefully, you have learned how to call REST API using GET
/POST
requests in Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn