Java で REST API を呼び出す
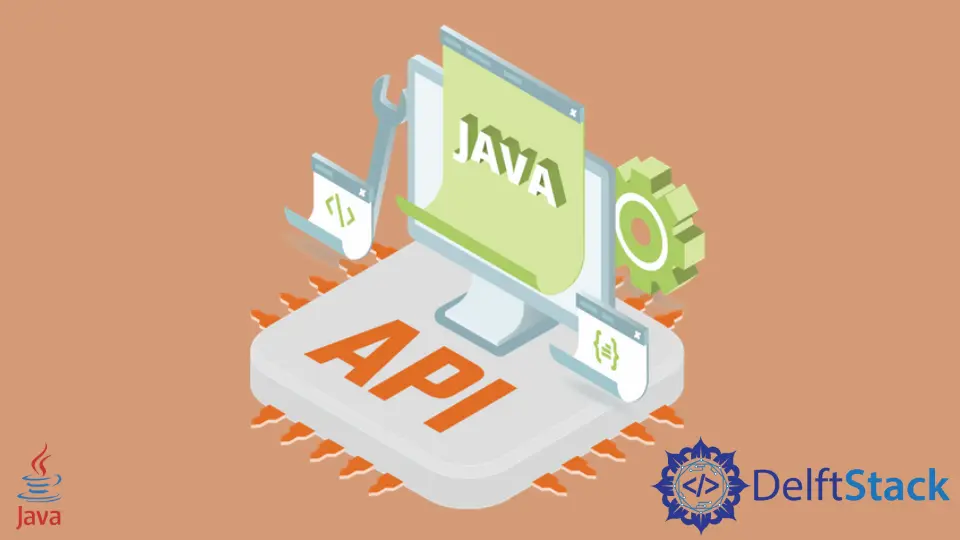
サーバーに接続できるプログラムに取り組んでいる場合は、REST API に精通している可能性があります。 この API を介して、特定のサーバーに接続できます。
この記事では、REST API、その動作と重要な機能、および GET
および POST
リクエストを使用して Java で REST API を呼び出す方法について学びます。
Java での REST API の概要
REST
という言葉は、Representational State Transfer
として定義されています。 それはどのように機能しますか? このシステムでは、プログラムはサーバーにリクエストを送信してデータを取得できるクライアントです。
一方、ここではサーバーがその呼び出しを受け取り、クライアントに応答します。 この要求と応答のペアは、HTTP
または Hyper Text Transfer Protocol
として知られるプロトコルに従います。
REST API の主な機能
REST API の主な機能は次のとおりです。
- REST API の設計はステートレスです。つまり、クライアントとサーバーが接続する場合、要求を完了するために追加の情報が必要になります。
- REST API は、パフォーマンスを向上させるためにリソースをキャッシュすることもできます。
- REST API は、クライアントが特定の言語でサーバーと通信できるようにする統一インターフェースを使用します。
GET
& POST
リクエストを使用して Java で REST API を呼び出す
GET
は指定されたリソースの表現を受け取り、POST
は処理対象のデータを識別されたリソースに書き込むために使用されます。 それらを使用して以下の REST API を呼び出す方法を学びましょう。
GET
リクエストを使用する
コード例:
// Importing all the necessary packages
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HTTPGet {
public static void main(String args[]) throws Exception {
// Providing the website URL
URL url = new URL("http://example.com");
// Creating an HTTP connection
HttpURLConnection MyConn = (HttpURLConnection) url.openConnection();
// Set the request method to "GET"
MyConn.setRequestMethod("GET");
// Collect the response code
int responseCode = MyConn.getResponseCode();
System.out.println("GET Response Code :: " + responseCode);
if (responseCode == MyConn.HTTP_OK) {
// Create a reader with the input stream reader.
BufferedReader in = new BufferedReader(new InputStreamReader(MyConn.getInputStream()));
String inputLine;
// Create a string buffer
StringBuffer response = new StringBuffer();
// Write each of the input line
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Show the output
System.out.println(response.toString());
} else {
System.out.println("Error found !!!");
}
}
}
上記の例では、GET
として知られる HTTP
で最も使用されるリクエスト メソッドを示しました。 サンプル コードの各行の目的は既に記述しています。
この例では、提供された URL に基づいて HTTP
接続を作成し、要求されたプロパティを定義しました。 その後、リクエストメソッドをGET
に設定します。
次に、InputStreamReader
を使用して BufferedReader
を作成し、各ドキュメント行を読み取って保存します。 最後に、Web サイトを出力として印刷します。
出力:
GET Response Code :: 200
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8">
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
POST
リクエストを使用する
コード例:
// Importing all the necessary packages
import java.io.*;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
public class HTTPPost {
public static void main(String[] args) throws MalformedURLException, IOException {
// Providing the website URL
URL myUrl = new URL("https://example.com");
// Creating an HTTP connection
HttpURLConnection MyConn = (HttpURLConnection) myUrl.openConnection();
// Set the request method to "POST"
MyConn.setRequestMethod("POST");
MyConn.setDoOutput(true);
// Creating an output stream
OutputStream out = MyConn.getOutputStream();
// Defining the sting to post
String postedString = "This is the posted text !!!!!!";
out.write(postedString.getBytes());
// Collect the response code
int responseCode = MyConn.getResponseCode();
System.out.print("Value of http created is:" + MyConn.HTTP_CREATED + "\n");
if (responseCode == MyConn.HTTP_CREATED) {
System.out.print("This is the response Code: " + responseCode + "\n");
System.out.print(
"This is the response Message fromserver: " + MyConn.getResponseMessage() + "\n");
} else {
System.out.print("GO HOME, EVERYBODY :( ");
}
// Creating an input stream reader
InputStreamReader in = new InputStreamReader(MyConn.getInputStream());
// Creating a buffered reader
BufferedReader buffer = new BufferedReader(in);
// Creating a string buffer
StringBuffer fromServer = new StringBuffer();
String eachLine = null;
// Writing each line of the document
while ((eachLine = buffer.readLine()) != null) {
fromServer.append(eachLine);
fromServer.append(System.lineSeparator());
}
buffer.close();
// Printing the html document
System.out.print("Here is our webpage:\n" + fromServer);
}
}
出力:
Value of http created is:201
GO HOME, EVERYBODY :( Here is our webpage:
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8">
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
上記の例では、POST
として知られる、HTTP
で最もよく使用される別のリクエスト メソッドを示しました。 この例では、提供された URL に基づいて HTTP
接続を作成し、要求されたプロパティを定義しました。
その後、リクエストメソッドを POST
に設定します。 次に、OutputStream
を設定して文字列を送信します。 その後、応答コード
を処理しました。
次に、InputStreamReader
で BufferedReader
を作成しました。 次に、すべてのドキュメントを保持する StringBuffer
を作成しました。 Java で GET
/POST
リクエストを使用して REST API を呼び出す方法を学んだことを願っています。
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn