Java에서 REST API 호출
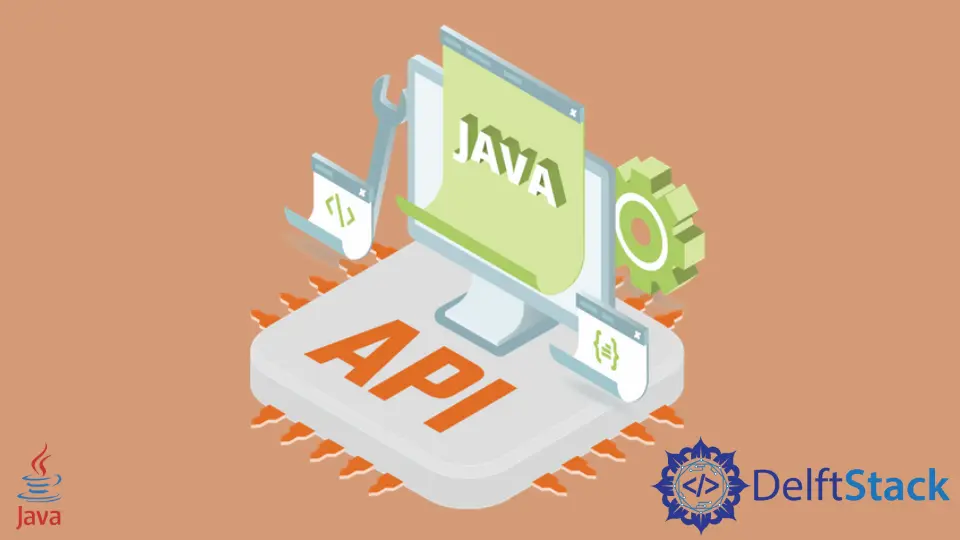
서버에 연결할 수 있는 프로그램을 작업 중이라면 REST API에 익숙할 것입니다. 이 API를 통해 특정 서버에 연결할 수 있습니다.
이 기사에서는 REST API, 작동 및 주요 기능, GET
및 POST
요청을 사용하여 Java에서 REST API를 호출하는 방법에 대해 알아봅니다.
Java의 REST API 개요
REST
라는 단어는 Representational State Transfer
로 정의됩니다. 어떻게 작동합니까? 이 시스템에서 프로그램은 일부 데이터를 얻기 위해 서버에 요청을 보낼 수 있는 클라이언트입니다.
반면 여기서 서버는 해당 호출을 수신하고 클라이언트에 응답합니다. 이 요청 및 응답 쌍은 HTTP
또는 Hyper Text Transfer Protocol
로 알려진 프로토콜을 따릅니다.
REST API의 주요 기능
다음은 REST API의 주요 기능입니다.
- REST API의 디자인은 상태 비저장입니다. 즉, 클라이언트와 서버가 연결을 원할 때 요청을 완료하기 위해 추가 정보가 필요합니다.
- REST API는 성능 향상을 위해 리소스를 캐시할 수도 있습니다.
- REST API는 클라이언트가 특정 언어로 서버와 대화할 수 있도록 하는 균일한 인터페이스를 사용합니다.
GET
및 POST
요청을 사용하여 Java에서 REST API 호출
GET
은 지정된 리소스의 표현을 받는 반면 POST
는 식별된 리소스에 처리할 데이터를 쓰는 데 사용됩니다. 아래에서 REST API를 호출하기 위해 어떻게 사용할 수 있는지 알아봅시다.
GET
요청 사용
예제 코드:
// Importing all the necessary packages
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HTTPGet {
public static void main(String args[]) throws Exception {
// Providing the website URL
URL url = new URL("http://example.com");
// Creating an HTTP connection
HttpURLConnection MyConn = (HttpURLConnection) url.openConnection();
// Set the request method to "GET"
MyConn.setRequestMethod("GET");
// Collect the response code
int responseCode = MyConn.getResponseCode();
System.out.println("GET Response Code :: " + responseCode);
if (responseCode == MyConn.HTTP_OK) {
// Create a reader with the input stream reader.
BufferedReader in = new BufferedReader(new InputStreamReader(MyConn.getInputStream()));
String inputLine;
// Create a string buffer
StringBuffer response = new StringBuffer();
// Write each of the input line
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Show the output
System.out.println(response.toString());
} else {
System.out.println("Error found !!!");
}
}
}
위의 예에서는 GET
으로 알려진 HTTP
에서 가장 많이 사용되는 요청 방법을 설명했습니다. 우리는 이미 예제 코드에서 각 줄의 목적을 작성했습니다.
이 예에서는 제공된 URL을 기반으로 HTTP
연결을 만든 다음 요청된 속성을 정의했습니다. 그런 다음 요청 방법을 GET
으로 설정합니다.
그런 다음 InputStreamReader
를 사용하여 BufferedReader
를 생성하여 각 문서 라인을 읽고 저장했습니다. 마지막으로 웹 사이트를 출력으로 인쇄합니다.
출력:
GET Response Code :: 200
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8">
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
POST
요청 사용
예제 코드:
// Importing all the necessary packages
import java.io.*;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
public class HTTPPost {
public static void main(String[] args) throws MalformedURLException, IOException {
// Providing the website URL
URL myUrl = new URL("https://example.com");
// Creating an HTTP connection
HttpURLConnection MyConn = (HttpURLConnection) myUrl.openConnection();
// Set the request method to "POST"
MyConn.setRequestMethod("POST");
MyConn.setDoOutput(true);
// Creating an output stream
OutputStream out = MyConn.getOutputStream();
// Defining the sting to post
String postedString = "This is the posted text !!!!!!";
out.write(postedString.getBytes());
// Collect the response code
int responseCode = MyConn.getResponseCode();
System.out.print("Value of http created is:" + MyConn.HTTP_CREATED + "\n");
if (responseCode == MyConn.HTTP_CREATED) {
System.out.print("This is the response Code: " + responseCode + "\n");
System.out.print(
"This is the response Message fromserver: " + MyConn.getResponseMessage() + "\n");
} else {
System.out.print("GO HOME, EVERYBODY :( ");
}
// Creating an input stream reader
InputStreamReader in = new InputStreamReader(MyConn.getInputStream());
// Creating a buffered reader
BufferedReader buffer = new BufferedReader(in);
// Creating a string buffer
StringBuffer fromServer = new StringBuffer();
String eachLine = null;
// Writing each line of the document
while ((eachLine = buffer.readLine()) != null) {
fromServer.append(eachLine);
fromServer.append(System.lineSeparator());
}
buffer.close();
// Printing the html document
System.out.print("Here is our webpage:\n" + fromServer);
}
}
출력:
Value of http created is:201
GO HOME, EVERYBODY :( Here is our webpage:
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8">
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
위의 예에서는 POST
로 알려진 HTTP
에서 가장 많이 사용되는 또 다른 요청 방법을 설명했습니다. 예제에서는 제공된 URL을 기반으로 HTTP
연결을 만든 다음 요청된 속성을 정의했습니다.
그런 다음 요청 방법을 POST
로 설정합니다. 그런 다음 OutputStream
을 설정하여 문자열을 게시합니다. 그 후 응답 코드
로 작업했습니다.
다음으로 InputStreamReader
를 사용하여 BufferedReader
를 만들었습니다. 그런 다음 모든 문서를 보관할 StringBuffer
를 만들었습니다. Java에서 GET
/POST
요청을 사용하여 REST API를 호출하는 방법을 배웠기를 바랍니다.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn