Llame a la API REST en Java
- Descripción general de la API REST en Java
-
Use las solicitudes
GET
yPOST
para llamar a la API REST en Java
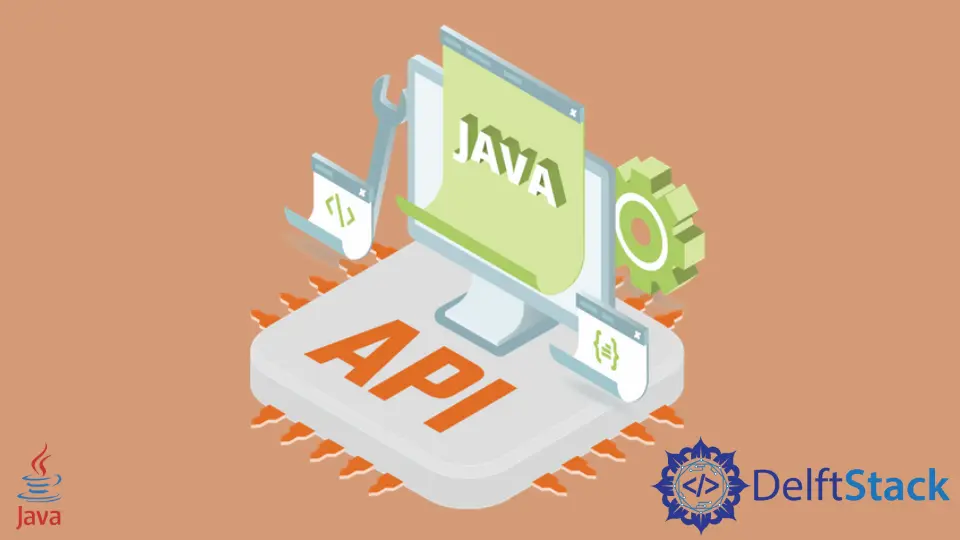
Si está trabajando en un programa que le permite conectarse con un servidor, es posible que esté familiarizado con la API REST. A través de esta API, puede conectarse con un servidor específico.
En este artículo, aprenderemos sobre la API REST, su funcionamiento y características clave, y cómo podemos usar las solicitudes GET
y POST
para llamar a la API REST en Java.
Descripción general de la API REST en Java
La palabra REST
se define como Transferencia de Estado Representacional
. ¿Como funciona? En este sistema, su programa es un cliente que puede enviar una solicitud al servidor para obtener algunos datos.
Por otro lado, el servidor aquí recibe esa llamada y responde al cliente. Este par de solicitud y respuesta sigue un protocolo conocido como HTTP
o Protocolo de transferencia de hipertexto
.
Características clave de la API REST
Las siguientes son las características clave de la API REST:
- El diseño de la API REST no tiene estado, lo que significa que cuando un cliente y un servidor desean conectarse, necesitarán información adicional para completar la solicitud.
- La API REST también puede almacenar recursos en caché para un mejor rendimiento.
- La API REST utiliza una interfaz uniforme que permite al cliente hablar con el servidor en un idioma específico.
Use las solicitudes GET
y POST
para llamar a la API REST en Java
El GET
recibe una representación de un recurso específico, mientras que POST
se utiliza para escribir datos que se procesarán en un recurso identificado. Aprendamos cómo podemos usarlos para llamar a la API REST a continuación.
Utilice la solicitud GET
Código de ejemplo:
// Importing all the necessary packages
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HTTPGet {
public static void main(String args[]) throws Exception {
// Providing the website URL
URL url = new URL("http://example.com");
// Creating an HTTP connection
HttpURLConnection MyConn = (HttpURLConnection) url.openConnection();
// Set the request method to "GET"
MyConn.setRequestMethod("GET");
// Collect the response code
int responseCode = MyConn.getResponseCode();
System.out.println("GET Response Code :: " + responseCode);
if (responseCode == MyConn.HTTP_OK) {
// Create a reader with the input stream reader.
BufferedReader in = new BufferedReader(new InputStreamReader(MyConn.getInputStream()));
String inputLine;
// Create a string buffer
StringBuffer response = new StringBuffer();
// Write each of the input line
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Show the output
System.out.println(response.toString());
} else {
System.out.println("Error found !!!");
}
}
}
En el ejemplo anterior, ilustramos el método de solicitud más utilizado en HTTP
, conocido como GET
. Ya escribimos el propósito de cada línea en el código de ejemplo.
En este ejemplo, creamos una conexión HTTP
basada en la URL proporcionada y luego definimos la propiedad solicitada. Después de eso, establecemos el método de solicitud en GET
.
Luego creamos un BufferedReader
con InputStreamReader
para leer y guardar cada línea del documento. Por último, imprimimos el sitio web como salida.
Producción :
GET Response Code :: 200
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8">
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
Utilice la solicitud POST
Código de ejemplo:
// Importing all the necessary packages
import java.io.*;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
public class HTTPPost {
public static void main(String[] args) throws MalformedURLException, IOException {
// Providing the website URL
URL myUrl = new URL("https://example.com");
// Creating an HTTP connection
HttpURLConnection MyConn = (HttpURLConnection) myUrl.openConnection();
// Set the request method to "POST"
MyConn.setRequestMethod("POST");
MyConn.setDoOutput(true);
// Creating an output stream
OutputStream out = MyConn.getOutputStream();
// Defining the sting to post
String postedString = "This is the posted text !!!!!!";
out.write(postedString.getBytes());
// Collect the response code
int responseCode = MyConn.getResponseCode();
System.out.print("Value of http created is:" + MyConn.HTTP_CREATED + "\n");
if (responseCode == MyConn.HTTP_CREATED) {
System.out.print("This is the response Code: " + responseCode + "\n");
System.out.print(
"This is the response Message fromserver: " + MyConn.getResponseMessage() + "\n");
} else {
System.out.print("GO HOME, EVERYBODY :( ");
}
// Creating an input stream reader
InputStreamReader in = new InputStreamReader(MyConn.getInputStream());
// Creating a buffered reader
BufferedReader buffer = new BufferedReader(in);
// Creating a string buffer
StringBuffer fromServer = new StringBuffer();
String eachLine = null;
// Writing each line of the document
while ((eachLine = buffer.readLine()) != null) {
fromServer.append(eachLine);
fromServer.append(System.lineSeparator());
}
buffer.close();
// Printing the html document
System.out.print("Here is our webpage:\n" + fromServer);
}
}
Producción :
Value of http created is:201
GO HOME, EVERYBODY :( Here is our webpage:
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8">
<meta http-equiv="Content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif;
}
div {
width: 600px;
margin: 5em auto;
padding: 2em;
background-color: #fdfdff;
border-radius: 0.5em;
box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02);
}
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
En el ejemplo anterior, ilustramos otro método de solicitud más utilizado en HTTP
, conocido como POST
. En el ejemplo, creamos una conexión HTTP
basada en la URL proporcionada y luego definimos la propiedad solicitada.
Después de eso, configuramos el método de solicitud en POST
. Luego, configuramos un OutputStream
para publicar la cadena; después de eso, trabajamos con el código de respuesta
.
A continuación, creamos un BufferedReader
con el InputStreamReader
. Luego creamos un StringBuffer
para contener todos los documentos. Con suerte, ha aprendido cómo llamar a la API REST usando solicitudes GET
/POST
en Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn