How to Get Today's Date in HTML Ultimate Guide
-
Use the JavaScript
Date()
Function to Get the Current Date in HTML -
Get the Current Date in the
y/m/d
Format in HTML -
Get the Current Date Using the JavaScript Method
toLocaleDateString()
in HTML - Formatting the Current Date
-
Get Current Today by Leveraging
Moment.js
-
Get the Current Date Using the JavaScript Method
toUTCString
in HTML - Conclusion
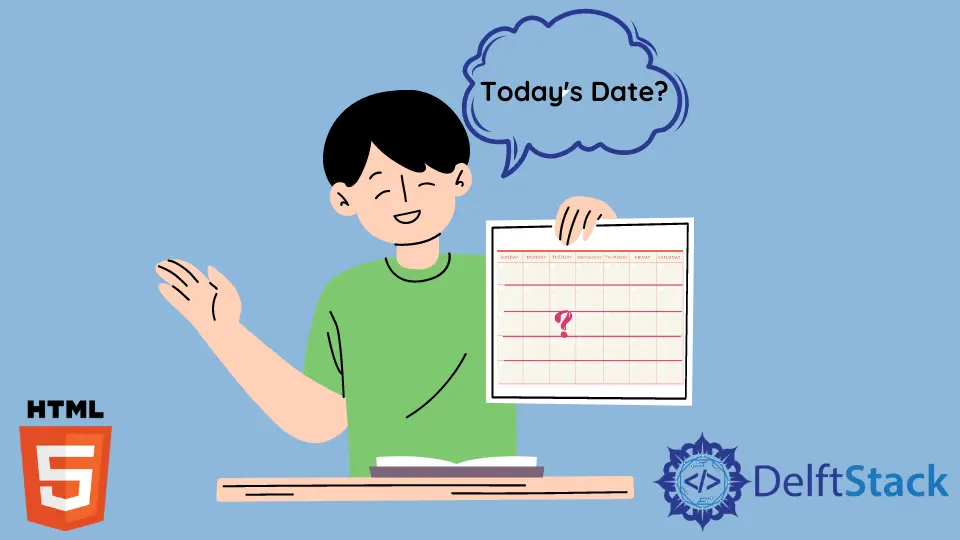
In this article, we will introduce a few methods to get the current date in HTML.
In today’s fast-paced digital world, where information is constantly being updated, displaying the current date on your website can provide visitors with valuable context. Whether you’re running a blog, news site, or an event platform, the current date helps users understand when the information was last updated or published.
Furthermore, the current date can contribute to a seamless user experience by adding a sense of real-time interaction.
Imagine a scenario where you’re organizing an international online conference with attendees from different time zones. Displaying the conference schedule with the current date and time in each attendee’s local time can ensure everyone knows when the sessions will take place, regardless of their location.
Use the JavaScript Date()
Function to Get the Current Date in HTML
We can use the JavaScript Date()
function to get the current date. The function returns the current date and time.
We can create an HTML container and display the current date in it using JavaScript. We will use the innerHTML
property to write the date in HTML.
Example Code:
<div id="current_date">
<script>
document.getElementById("current_date").innerHTML = Date();
</script>
</div>
In our HTML structure, we employ the <div> tag with id="current_date"
as a placeholder for displaying the date.
Within the accompanying <script>
tag, JavaScript dynamically sets the content of the <div>
using the document.getElementById("current_date")
function to access the specified <div>
element.
Leveraging the innerHTML
property facilitates the injection of content into the selected HTML element.
The Date()
method in JavaScript fetches the current date and time, which is then assigned to the innerHTML
of the <div>
, ensuring the visibility of the current date and time on the webpage.
Output:
Get the Current Date in the y/m/d
Format in HTML
We can also find the current date in y/m/d
format using JavaScript Date()
methods and embed it in an HTML file. We can get the current year from the getFullYear()
method, the current month from the getMonth()
method, and the current day from the getDate()
method.
In this method, we will use the Date()
object to access these various functions. We can format the date in any way we want and display it on an HTML page using the innerHTML
property.
Example Code:
<div id="current_date">
<script>
date = new Date();
year = date.getFullYear();
month = date.getMonth() + 1;
day = date.getDate();
document.getElementById("current_date").innerHTML = month + "/" + day + "/" + year;
</script>
</div>
In our process of date display, we first create a new Date
object representing the current date and time using var date = new Date();
.
To extract the year, month, and day, we employ getFullYear()
, getMonth() + 1
, and getDate()
respectively.
Since getMonth()
returns a zero-based index, we add 1 to align it with conventional month numbering (1-12).
Finally, we dynamically display the formatted date string by injecting it into the HTML element with the id current_date
, using document.getElementById("current_date").innerHTML
.
Output:
Get the Current Date Using the JavaScript Method toLocaleDateString()
in HTML
We can use the JavaScript function toLocaleDateString()
to find the current date. The toLocaleDateString()
function returns the current date according to the language provided in the function.
There are various language-specific conventions, and we can define the language with the toLocaleDateString()
function. The function takes two parameters, which are locales
and options
.
We can specify the language of the output by the locales
option. For example, we can use en-US
for US English and en-GB
for British English.
Example Code:
<div>
<script>
date = new Date().toLocaleDateString();
document.write(date);
</script>
</div>
In our JavaScript code, we initiate the creation of a Date
object using new Date()
, capturing the current date and time.
For localization, we employ toLocaleDateString()
on the Date
object, automatically adapting to the browser’s locale settings when specific parameters are not provided.
Subsequently, we utilize document.write(date)
to seamlessly incorporate the localized date string into the HTML document, precisely within the designated <div>
element.
This approach ensures the accurate display of the localized date on our webpage.
Output:
Formatting the Current Date
While we’ve delved into the y/m/d
format and the toLocaleDateString()
method, let’s expand our horizons and explore a range of formatting possibilities. This will empower readers with a diverse array of choices for tailoring date displays:
Displaying the Day Name
To augment the user’s comprehension of the displayed date, consider incorporating the day name. JavaScript’s getDay()
method supplies the day of the week as a numerical value (0 for Sunday, 1 for Monday, and so forth).
By mapping these numbers to their respective day names, you can present a more informative result.
<div id="current_date">
<script>
const daysOfWeek = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
const date = new Date();
const dayName = daysOfWeek[date.getDay()];
document.getElementById("current_date").innerHTML = `${dayName}, ${date.toLocaleDateString()}`;
</script>
</div>
In our approach to displaying dates, we start by defining daysOfWeek
, an array that contains the names of the week’s days.
We then create a new Date
object with const date = new Date();
, representing the current date and time.
To fetch the day’s name, we use date.getDay()
, which returns a numeric value corresponding to the current day of the week, and this value is then used to retrieve the appropriate day name from daysOfWeek
.
Finally, we concatenate this day name with the localized date string and set this combination as the inner HTML of the div
with the id current_date
, ensuring our webpage displays both the day and date in a user-friendly format.
Output:
Human-Readable Format
For a natural, human-friendly date presentation, we can employ the toLocaleDateString()
method with supplementary options to render the month and day in a descriptive format.
<div id="current_date">
<script>
const options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' };
document.getElementById("current_date").innerHTML = new Date().toLocaleDateString('en-US', options);
</script>
</div>
In our process of date formatting, we begin by defining format options within the options object, specifying the desired display elements such as the full name of the weekday, the numeric form of the year, and the long form of the month and day.
We then utilize the toLocaleDateString()
method on a new Date
object, specifying the en-US
locale and incorporating the predefined options to format the date accordingly.
Finally, we update the HTML content by setting the innerHTML
property of the current_date
div to the formatted date string, ensuring the display of the date in the specified format on our webpage.
Output:
Get Current Today by Leveraging Moment.js
Moment.js
, a widely embraced JavaScript library tailored for date and time manipulation, extends an extensive spectrum of formatting options. This flexibility facilitates tailoring the date display to align seamlessly with your website’s design.
In addition to the previously mentioned example, let’s explore a few more formatting options that Moment.js
provides:
Custom Date Format
Moment.js
empowers you to craft a customized date format by arranging different placeholders. For instance, consider the following code that displays the date as YYYY-MM-DD
:
<div id="current_date">
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.1/moment.min.js"></script>
<script>
const formattedDate = moment().format('YYYY-MM-DD');
document.getElementById("current_date").innerHTML = formattedDate;
</script>
</div>
In our script, we first incorporate the Moment.js
library through a CDN link, availing its range of functions for our use.
We then create a Moment.js
object representing the current date and time by invoking moment()
.
This object is formatted into a year-month-day format using the format('YYYY-MM-DD')
method.
Finally, we display this formatted date string by assigning it to the inner HTML of the div
with the ID current_date
, ensuring that the current date is presented in a clear, standardized format on our webpage.
Output:
Relative Time
Moment.js enables you to present dates in a human-readable, relative format, expressing time intervals in terms like a few seconds ago
, in 5 minutes
, or 2 days ago
. This approach adds a dynamic dimension to your date presentation, enhancing the user’s sense of immediacy.
<div id="current_date">
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.1/moment.min.js"></script>
<script>
const formattedDate = moment().fromNow();
document.getElementById("current_date").innerHTML = `Last updated: ${formattedDate}`;
</script>
</div>
In our script implementation, we integrate the Moment.js
library by including it through a CDN, thereby making its functionalities accessible for use.
To generate relative time, we employ the moment().fromNow()
method, which calculates the time difference from the current moment.
The resulting relative time string is then incorporated into a message, Last updated:
, and set as the inner HTML content of the div
with the ID current_date
.
This process ensures that our webpage displays the last update time in a human-readable format, providing users with real-time information on when the content was last modified.
Output:
Localized Formats
Moment.js
boasts comprehensive localization capabilities, accommodating various languages and cultural preferences. By specifying the desired locale, you can ensure that the date display adheres to the conventions of different regions.
<div id="current_date">
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.1/moment-with-locales.min.js"></script>
<script>
// Set the desired locale
moment.locale('fr'); // French
const formattedDate = moment().format('LL'); // Display date in the localized long format
document.getElementById("current_date").innerHTML = formattedDate;
</script>
</div>
In our approach to date display, we first include Moment.js with its locale data in the script tag, enabling us to format dates in a localized manner.
We set the locale to French using moment.locale('fr')
, influencing the formatting style of the date.
Utilizing moment().format('LL')
, we format the current date in a long format that aligns with the French locale settings.
Finally, we ensure this formatted date is displayed in the HTML element identified by the ID current_date
, providing our webpage viewers with a localized and easily understandable date presentation.
Output:
Calendar Time
Moment.js
offers the ability to represent dates as calendar time, providing a clear context of the day, month, and year.
<div id="current_date">
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.1/moment.min.js"></script>
<script>
const formattedDate = moment().calendar();
document.getElementById("current_date").innerHTML = `Today's date: ${formattedDate}`;
</script>
</div>
We enhance date and time manipulation by incorporating Moment.js
through a script tag import, leveraging this robust library’s capabilities.
To present dates in a user-friendly, relative format, we use moment().calendar()
, which formats the current date in a style that reflects its proximity to the present moment.
The resulting formatted date is then displayed within the HTML element identified by the ID current_date
, ensuring that visitors to our webpage have a clear and contextually relevant understanding of time-related information.
Output:
By harnessing the capabilities of Moment.js
, you can not only format the date in diverse ways but also imbue your date display with a dynamic, language-specific, and culturally relevant character.
This library enriches your toolkit by offering an array of functionalities that cater to the nuances of presenting dates in a web context.
Get the Current Date Using the JavaScript Method toUTCString
in HTML
The toUTCString
method serves the purpose of converting a Date
object to a string, representing the date and time in UTC. UTC is a time standard that serves as the basis for timekeeping worldwide.
This method is particularly useful when a standardized, global representation of time is required, regardless of the user’s local time zone.
<div id="current_date">
<script>
const currentDate = new Date();
const formattedDate = currentDate.toUTCString();
document.getElementById("current_date").innerHTML = `Today's date in UTC: ${formattedDate}`;
</script>
</div>
In our implementation, we begin by creating a new Date
object with const currentDate = new Date();
, which captures the current date and time.
We then utilize the currentDate.toUTCString()
method to convert this Date
object into a string representation of the date and time in UTC format.
The final step involves displaying this formatted date string within the HTML element identified by the ID current_date
, ensuring that the current UTC date and time are clearly presented on our web page.
Output:
Conclusion
This comprehensive guide on how to display the current date in HTML offers a variety of methods to suit different requirements and preferences. Starting with the basic JavaScript Date()
function, the article demonstrates how to display the current date in HTML form using simple JavaScript embedding.
It further explores formatting the date in the y/m/d
format and using the toLocaleDateString()
method for language-specific date representation. The guide then delves into more advanced formatting options, such as displaying the day name and a human-readable format, leveraging the extensive capabilities of the Moment.js library.
Moment.js
enables custom date formats, relative time presentations, localized formats, and calendar time representations, offering a versatile tool for date manipulation and display. Lastly, the guide discusses the use of the JavaScript toUTCString
method for displaying the date and time in the standardized UTC format.
Overall, this article serves as an ultimate guide, offering a range of solutions and ensuring that readers can choose the most suitable method for incorporating the current date into their HTML content.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn