How to Iterate Over an Entire Map in Go
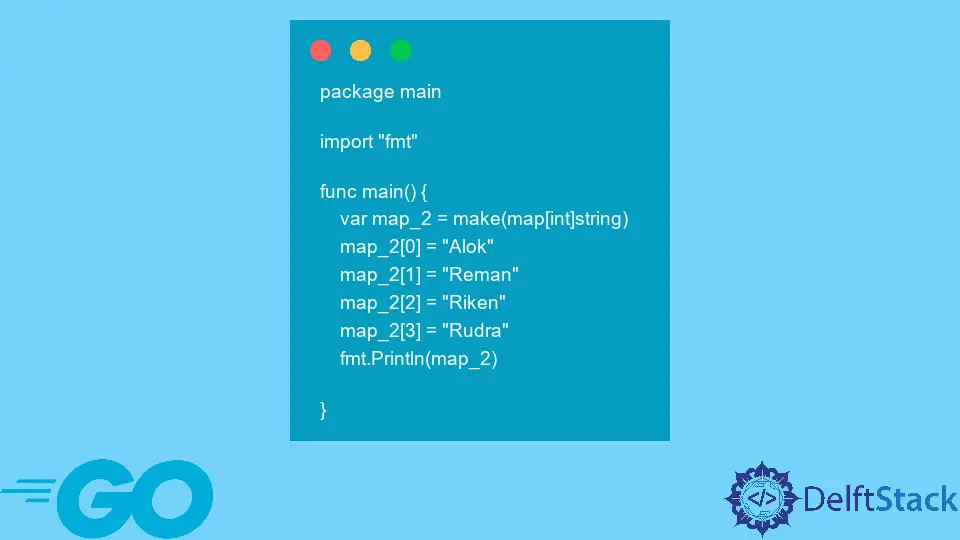
Hash tables are one of the most powerful and versatile data structures in Computer Science. There are varieties of hash table implementations for different programming languages. In Go, hash tables are implemented as built-in map
data types. In short, a map
is a collection of key-value
pairs. We use for...range
statement in Go to loop over the entire keys
of a map
by retrieving one key
at a time.
Declare and Initialize Maps
A map
can be declared using the following syntax:
var map_name map[KeyType]ValueType
Where KeyType
is any comparable datatype
and ValueType
is also any data-type including map
itself too.
package main
import "fmt"
func main() {
map_1 := map[int]string{
0: "Alok",
1: "Reman",
2: "Riken",
3: "Rudra",
}
fmt.Println(map_1)
}
Output:
map[0:Alok 1:Reman 2:Riken 3:Rudra]
Maps
can also be created using the inbuilt make()
function.
package main
import "fmt"
func main() {
var map_2 = make(map[int]string)
map_2[0] = "Alok"
map_2[1] = "Reman"
map_2[2] = "Riken"
map_2[3] = "Rudra"
fmt.Println(map_2)
}
Output:
map[0:Alok 1:Reman 2:Riken 3:Rudra]
Iterate Over a Map in Go
We can iterate over the entire map
using for...range
statements. However, iteration order over a map
is not fixed as the map
is an unordered collection.
Iterate Over All Keys and Values of a map
in Go
package main
import "fmt"
func main() {
map_1 := map[int]string{
0: "Alok",
1: "Reman",
2: "Riken",
3: "Rudra",
}
for k, v := range map_1 {
fmt.Printf("Key:%v Value: %s\n", k, v)
}
}
Output:
Key:0 Value: Alok
Key:1 Value: Reman
Key:2 Value: Riken
Key:3 Value: Rudra
However, if you are using different data-type for keys
or values
of the map
, then you must specify the format for that particular data-type while printing keys
or values
. An example of a map
with string
data-type as keys
is shown below:
package main
import "fmt"
func main() {
map_1 := map[string]string{
"Giri ": "Alok",
"Nembang ": "Reman",
"Maharjan": "Riken",
"Jha ": "Rudra",
}
for k, v := range map_1 {
fmt.Printf("Key:%v Value: %s\n", k, v)
}
}
Output:
Key:Giri Value: Alok
Key:Nembang Value: Reman
Key:Maharjan Value: Riken
Key:Jha Value: Rudra
Iterate Over Keys Only in Go map
package main
import "fmt"
func main() {
map_1 := map[int]string{
0: "Alok",
1: "Reman",
2: "Riken",
3: "Rudra",
}
for k := range map_1 {
fmt.Printf("Key:%v\n", k)
}
}
Output:
Key:0
Key:1
Key:2
Key:3
Iterate Over Values Only in Go map
package main
import "fmt"
func main() {
map_1 := map[int]string{
0: "Alok",
1: "Reman",
2: "Riken",
3: "Rudra",
}
for _, v := range map_1 {
fmt.Printf("Value:%s\n", v)
}
}
Output:
Value:Riken
Value:Rudra
Value:Alok
Value:Reman
The order in which the key
and value
pairs in the output above and order in which key-value
pairs are printed on your side may differ because the map
is an unordered collection. So, you need not worry about that.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn