How to Declare a Constant Map in Golang
- Understanding Constants in Go
- Maps in Go
- Challenges in Declaring Constant Maps in Go
-
Declare a Constant Map Using
map[int]string
in Go -
Declare a Constant Map Using
map[string]int
in Go - Use a Function to Return Constant-Like Maps in Go
-
Use
make
andconst
in a Unique Way (Pre-Go 1.18) - Considerations
- Conclusion
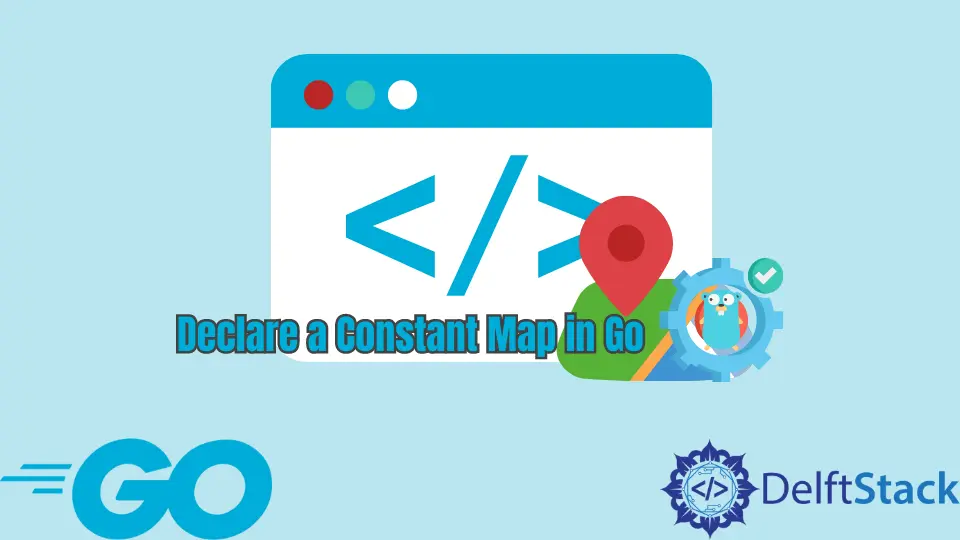
In Go, constants must be determinable at compile-time, and maps, being reference types that require allocation, don’t fit this requirement. Hence, you can’t directly declare a map as a constant.
However, you can create a variable and initialize it once with values that you treat as constant throughout the program’s execution. Although the map variable itself can be modified, its values can remain unchanged, effectively creating a similar effect to a constant map.
Understanding Constants in Go
In Go, constants are values that are known at compile time and cannot be changed during the execution of a program.
They are immutable and must be initialized with constant expressions. This means their values must be determinable at compile-time and cannot be altered after that.
Maps in Go
Maps in Go are a collection of unordered key-value pairs. They provide an efficient way to store and retrieve data based on unique keys.
Maps are reference types and are initialized using the make()
function or a shorthand declaration with map[keyType]valueType{}
syntax.
Challenges in Declaring Constant Maps in Go
Creating a true constant map in Go is challenging because maps, being reference types that require allocation, don’t fit the criteria for constants. Go’s constant declarations must be resolvable at compile-time, whereas maps need runtime allocation.
Declare a Constant Map Using map[int]string
in Go
Although direct constant maps are not feasible in Go due to map properties requiring runtime allocation, you can create a variable initialized once and treat it as a constant map throughout the program.
In Go, the syntax for a map with int
keys and string
values is defined as follows:
var myMap map[int]string
This line declares a variable named myMap
that is a map with int
keys and string
values. However, this declaration only creates the map variable; it doesn’t initialize it.
To initialize the map, you need to use make()
or initialize it directly with values.
Example:
package main
import (
"fmt"
)
var constMap = map[int]string{
1: "Jay",
2: "Adam",
3: "Mike",
4: "Wiz",
5: "Lucas",
}
func main() {
fmt.Println(constMap[1])
fmt.Println(constMap[5])
}
Output:
Jay
Lucas
This code snippet initializes a global variable constMap
as a map with integer keys and string values. The map is populated with names associated with specific integer keys.
Inside the main()
function, it demonstrates how to access and print values from this map based on the keys provided. The fmt.Println()
statements within main()
retrieve and print the values associated with the keys 1
and 5
from constMap
.
The output of this code would be the names "Jay"
(associated with key 1
) and "Lucas"
(associated with key 5
), showcasing how to retrieve specific values stored in the map using integer keys.
Declare a Constant Map Using map[string]int
in Go
In Go, the syntax for a map with string
keys and int
values is defined as follows:
var myMap map[string]int
This line declares a variable named myMap
that is a map with string
keys and int
values.
However, similar to other maps in Go, this declaration only creates the map variable; it doesn’t initialize it. To initialize the map, you can use make()
or initialize it directly with values.
Here’s an example of how to initialize and use a map[string]int
:
package main
import (
"fmt"
)
var constMap = map[string]int{
"Jay": 1,
"Adam": 2,
"Mike": 3,
"Wiz": 4,
"Lucas": 5,
}
func main() {
fmt.Println(constMap["Jay"])
fmt.Println(constMap["Lucas"])
}
Output:
1
5
This Go code initializes a global variable constMap
as a map with string keys and integer values. The map is populated with names as keys and specific integers as their associated values.
Within the main()
function, fmt.Println()
statements showcase how to access and print values from this map using specific string keys ("Jay"
and "Lucas"
).
fmt.Println(constMap["Jay"])
retrieves and prints the value associated with the key "Jay"
, which is 1
, and fmt.Println(constMap["Lucas"])
retrieves and prints the value associated with the key "Lucas"
, which is 5
.
The code demonstrates how to access and retrieve values from the constMap
using string keys, displaying the associated integers for the respective names.
Use a Function to Return Constant-Like Maps in Go
In Go, constant maps—where both the map structure and its contents are immutable—aren’t directly supported due to limitations with constants and map types. However, using functions to return maps initialized with constant values provides a way to achieve constant-like behavior.
The approach involves creating a function that initializes and returns a map with predefined constant values. This function acts as a factory method, producing the constant-like map whenever invoked.
By design, the map returned from the function remains unchanged throughout the program’s execution, akin to a constant.
Let’s create a function getConstantMap()
that generates a map containing predefined constant values:
package main
import (
"fmt"
)
func getConstantMap() map[int]string {
return map[int]string{
1: "Apple",
2: "Banana",
3: "Orange",
}
}
func main() {
// Using the function to get the constant-like map
constantMap := getConstantMap()
// Accessing and printing values from the constant-like map
fmt.Println("Value for key 1:", constantMap[1])
fmt.Println("Value for key 2:", constantMap[2])
fmt.Println("Value for key 3:", constantMap[3])
}
Output:
Value for key 1: Apple
Value for key 2: Banana
Value for key 3: Orange
In this example, the getConstantMap()
is a function that returns a map with int
keys and string
values. The map is initialized with predefined constant values, representing fruits associated with integer keys.
In main()
, the getConstantMap()
function is called, and the returned map is assigned to the constantMap
variable. Subsequently, specific values are accessed and printed from constantMap
using integer keys.
Use make
and const
in a Unique Way (Pre-Go 1.18)
In earlier versions of Go (pre-Go 1.18), direct initialization of maps as constants wasn’t possible due to language limitations. However, a unique workaround involving the utilization of make
and const
keywords was employed to create constant-like maps.
This approach provided a means to initialize a map once and treat it as immutable throughout program execution.
The technique involves defining an immediately invoked function expression (IIFE) that utilizes make
to create a map, initializes it with desired constant values, and returns the resulting map. This IIFE is invoked immediately, generating a map that acts as a constant-like structure by not allowing the reassignment of the variable to a different map.
Let’s create an example demonstrating the use of make
and const
in a unique way to generate a constant-like map:
package main
import "fmt"
func main() {
// Using a unique method for a constant-like map pre-Go 1.18
var colors = func() map[string]string {
m := make(map[string]string)
m["red"] = "#ff0000"
m["green"] = "#00ff00"
m["blue"] = "#0000ff"
return m
}()
// Accessing and printing values from the constant-like map
fmt.Println("Red color code:", colors["red"])
fmt.Println("Green color code:", colors["green"])
fmt.Println("Blue color code:", colors["blue"])
}
Output:
Red color code: #ff0000
Green color code: #00ff00
Blue color code: #0000ff
The code demonstrates a unique technique used in earlier versions of Go (pre-1.18) to create a constant-like map. It utilizes an immediately invoked function expression (IIFE) to initialize and assign a map containing color names as keys and their respective hexadecimal codes as values to a variable named colors
.
This method, though not enforcing true immutability, achieves a constant-like behavior by initializing the map once upon declaration and prevents its reassignment throughout the program.
The code showcases accessing and printing specific values from this map, demonstrating the constant-like behavior created by this approach.
Considerations
- In the first three methods of this article, the map itself is immutable (constant), but the values within the map can still be changed. To ensure immutability, consider using frozen structures or libraries providing immutability.
- Starting from Go 1.18, maps can be initialized directly as constants, simplifying the process.
Remember, while these methods create constant-like maps in Go, they might not provide complete immutability for the underlying values within the map. Always ensure the intended immutability based on your application’s requirements.
Conclusion
In Go, directly creating constant maps isn’t feasible due to language restrictions. However, workarounds exist to achieve constant-like maps.
You can declare a variable and initialize it once, treating it as constant throughout program execution. Although the map variable itself remains mutable, its values remain unchanged, emulating a constant map.
The article explores different techniques, including initializing maps with specific keys and values, employing functions to return constant-like maps, and leveraging make
and const
before Go 1.18 to create maps that act as constants.
While these methods offer constant-like behavior for maps, the underlying values can still be altered. Understanding these workarounds helps manage maps effectively in Go applications, catering to various immutability needs.
Additionally, from Go 1.18 onwards, initializing maps directly as constants simplifies this process, offering a more straightforward approach for constant map creation.