GoLang Map of Maps
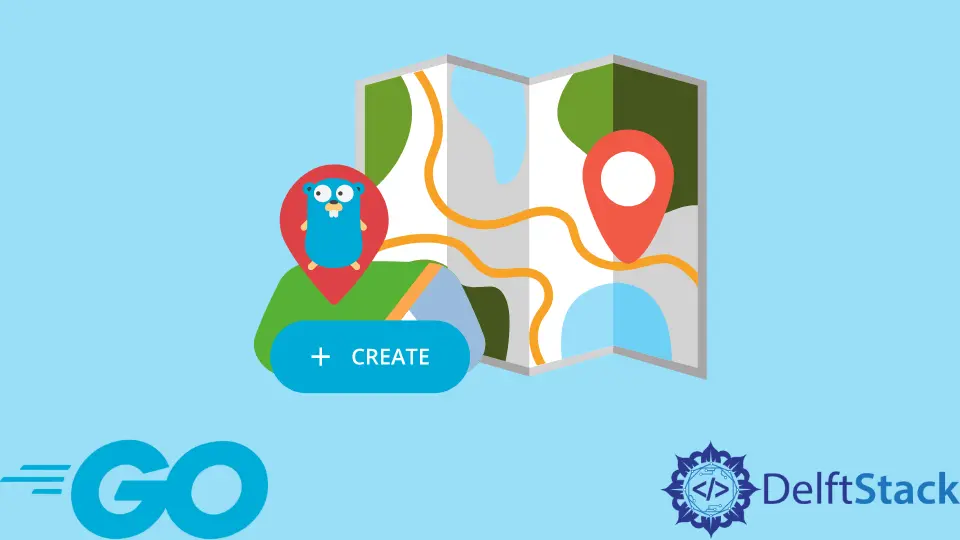
This tutorial demonstrates how to create a map of maps in GoLang.
GoLang Map of Maps
The map of maps or the nested map is those where the key-value pairs the values are also the maps. Creating a map of maps is possible in GoLang.
When we define a map, we have to define the type of key and value. In this case, the type of key can be int
, string
, or any other type, and the type of value will be map
again.
Syntax:
var DemoMap = map[string]map[string]string{}
or
var DemoMap = map[int]map[string]string{}
As we can see, the DemoMap
is defined with the key string, int type, and value as the map type. Let’s have an example.
Code:
package main
import "fmt"
func main() {
DemoMap := map[int]map[string]string{
1: {
"one": "Delftstack1",
"two": "Delftstack2",
"three": "Delftstack3",
},
2: {
"four": "Delftstack4",
"five": "Delftstack5",
"Six": "Delftstack6",
},
3: {
"seven": "Delftstack7",
"eight": "Delftstack8",
"nine": "Delftstack9",
},
4: {
"ten": "Delftstack10",
"eleven": "Delftstack11",
"twelve": "Delftstack12",
},
}
fmt.Println(DemoMap)
}
The code above creates a map of maps with key integer and value map and then prints the map.
Output:
map[
1:map[one:Delftstack1 three:Delftstack3 two:Delftstack2]
2:map[Six:Delftstack6 five:Delftstack5 four:Delftstack4]
3:map[eight:Delftstack8 nine:Delftstack9 seven:Delftstack7]
4:map[eleven:Delftstack11 ten:Delftstack10 twelve:Delftstack12]]
Delete a Nested Map
We can use the delete()
method of GoLang to delete a nested map. It takes two parameters, one is the parent map, and the other is the nested map key.
Let’s have an example.
Code:
package main
import "fmt"
func main() {
DemoMap := map[int]map[string]string{
1: {
"one": "Delftstack1",
"two": "Delftstack2",
"three": "Delftstack3",
},
2: {
"four": "Delftstack4",
"five": "Delftstack5",
"Six": "Delftstack6",
},
3: {
"seven": "Delftstack7",
"eight": "Delftstack8",
"nine": "Delftstack9",
},
4: {
"ten": "Delftstack10",
"eleven": "Delftstack11",
"twelve": "Delftstack12",
},
}
delete(DemoMap, 3)
fmt.Println(DemoMap)
}
The code above will delete the nested map at key 3 from the parent map.
Output:
map[
1:map[one:Delftstack1 three:Delftstack3 two:Delftstack2]
2:map[Six:Delftstack6 five:Delftstack5 four:Delftstack4]
4:map[eleven:Delftstack11 ten:Delftstack10 twelve:Delftstack12]]
Similarly, we can delete a member of the nested map using the same delete()
method where the first parameter will be the nested map and the second parameter will be the key we want to delete.
Code:
package main
import "fmt"
func main() {
DemoMap := map[int]map[string]string{
1: {
"one": "Delftstack1",
"two": "Delftstack2",
"three": "Delftstack3",
},
2: {
"four": "Delftstack4",
"five": "Delftstack5",
"Six": "Delftstack6",
},
3: {
"seven": "Delftstack7",
"eight": "Delftstack8",
"nine": "Delftstack9",
},
4: {
"ten": "Delftstack10",
"eleven": "Delftstack11",
"twelve": "Delftstack12",
},
}
delete(DemoMap[3], "seven")
fmt.Println(DemoMap)
}
The code above will delete a member of the nested map at key 3 with the member key seven
.
Output:
map[
1:map[one:Delftstack1 three:Delftstack3 two:Delftstack2]
2:map[Six:Delftstack6 five:Delftstack5 four:Delftstack4]
3:map[eight:Delftstack8 nine:Delftstack9]
4:map[eleven:Delftstack11 ten:Delftstack10 twelve:Delftstack12]]
Iterate Over a Nested Map
To iterate over the nested map, we have to give the index number of the map with the for
loop. Let’s have an example where we iterate through a nested map.
Code:
package main
import "fmt"
func main() {
DemoMap := map[int]map[string]string{
1: {
"one": "Delftstack1",
"two": "Delftstack2",
"three": "Delftstack3",
},
2: {
"four": "Delftstack4",
"five": "Delftstack5",
"Six": "Delftstack6",
},
3: {
"seven": "Delftstack7",
"eight": "Delftstack8",
"nine": "Delftstack9",
},
4: {
"ten": "Delftstack10",
"eleven": "Delftstack11",
"twelve": "Delftstack12",
},
}
for key, value := range DemoMap[3] {
fmt.Println(key, value)
}
}
The above code will iterate over the map at the third position in the parent map.
Output:
seven Delftstack7
eight Delftstack8
nine Delftstack9
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook