GoLang Mapa de mapas
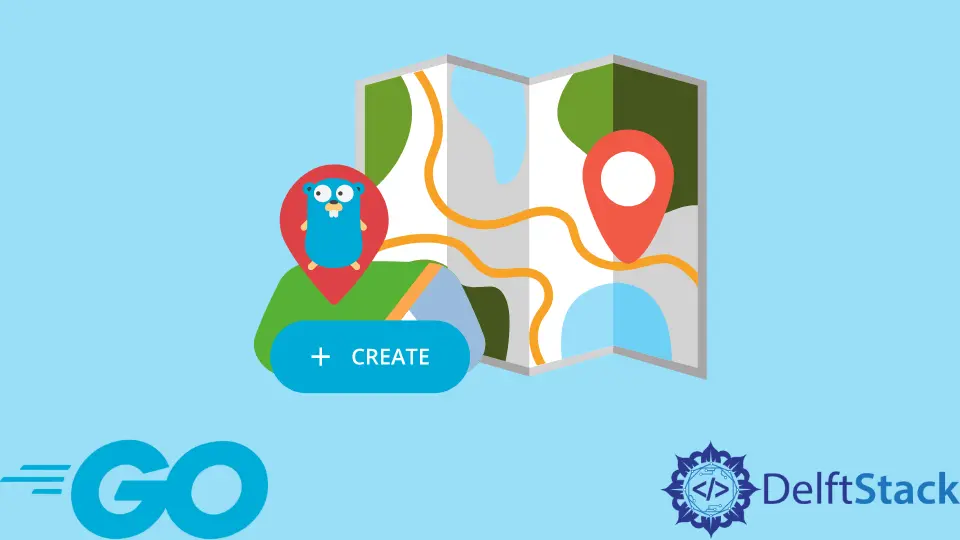
Este tutorial demuestra cómo crear un mapa de mapas en GoLang.
GoLang Mapa de mapas
El mapa de mapas o el mapa anidado son aquellos donde los pares clave-valor los valores también son los mapas. Crear un mapa de mapas es posible en GoLang.
Cuando definimos un mapa, tenemos que definir el tipo de clave y valor. En este caso, el tipo de clave puede ser int
, string
, o cualquier otro tipo, y el tipo de valor volverá a ser map
.
Sintaxis:
var DemoMap = map[string]map[string]string{}
o
var DemoMap = map[int]map[string]string{}
Como podemos ver, el DemoMap
se define con la cadena de clave, el tipo int y el valor como tipo de mapa. Tengamos un ejemplo.
Código:
package main
import "fmt"
func main() {
DemoMap := map[int]map[string]string{
1: {
"one": "Delftstack1",
"two": "Delftstack2",
"three": "Delftstack3",
},
2: {
"four": "Delftstack4",
"five": "Delftstack5",
"Six": "Delftstack6",
},
3: {
"seven": "Delftstack7",
"eight": "Delftstack8",
"nine": "Delftstack9",
},
4: {
"ten": "Delftstack10",
"eleven": "Delftstack11",
"twelve": "Delftstack12",
},
}
fmt.Println(DemoMap)
}
El código anterior crea un mapa de mapas con enteros clave y un mapa de valores y luego imprime el mapa.
Producción :
map[
1:map[one:Delftstack1 three:Delftstack3 two:Delftstack2]
2:map[Six:Delftstack6 five:Delftstack5 four:Delftstack4]
3:map[eight:Delftstack8 nine:Delftstack9 seven:Delftstack7]
4:map[eleven:Delftstack11 ten:Delftstack10 twelve:Delftstack12]]
Eliminar un mapa anidado
Podemos usar el método delete()
de GoLang para eliminar un mapa anidado. Toma dos parámetros, uno es el mapa principal y el otro es la clave del mapa anidado.
Tengamos un ejemplo.
Código:
package main
import "fmt"
func main() {
DemoMap := map[int]map[string]string{
1: {
"one": "Delftstack1",
"two": "Delftstack2",
"three": "Delftstack3",
},
2: {
"four": "Delftstack4",
"five": "Delftstack5",
"Six": "Delftstack6",
},
3: {
"seven": "Delftstack7",
"eight": "Delftstack8",
"nine": "Delftstack9",
},
4: {
"ten": "Delftstack10",
"eleven": "Delftstack11",
"twelve": "Delftstack12",
},
}
delete(DemoMap, 3)
fmt.Println(DemoMap)
}
El código anterior eliminará el mapa anidado en la clave 3 del mapa principal.
Producción :
map[
1:map[one:Delftstack1 three:Delftstack3 two:Delftstack2]
2:map[Six:Delftstack6 five:Delftstack5 four:Delftstack4]
4:map[eleven:Delftstack11 ten:Delftstack10 twelve:Delftstack12]]
Del mismo modo, podemos eliminar un miembro del mapa anidado usando el mismo método delete()
donde el primer parámetro será el mapa anidado y el segundo parámetro será la clave que queremos eliminar.
Código:
package main
import "fmt"
func main() {
DemoMap := map[int]map[string]string{
1: {
"one": "Delftstack1",
"two": "Delftstack2",
"three": "Delftstack3",
},
2: {
"four": "Delftstack4",
"five": "Delftstack5",
"Six": "Delftstack6",
},
3: {
"seven": "Delftstack7",
"eight": "Delftstack8",
"nine": "Delftstack9",
},
4: {
"ten": "Delftstack10",
"eleven": "Delftstack11",
"twelve": "Delftstack12",
},
}
delete(DemoMap[3], "seven")
fmt.Println(DemoMap)
}
El código anterior eliminará un miembro del mapa anidado en la clave 3 con la clave de miembro siete
.
Producción :
map[
1:map[one:Delftstack1 three:Delftstack3 two:Delftstack2]
2:map[Six:Delftstack6 five:Delftstack5 four:Delftstack4]
3:map[eight:Delftstack8 nine:Delftstack9]
4:map[eleven:Delftstack11 ten:Delftstack10 twelve:Delftstack12]]
Iterar sobre un mapa anidado
Para iterar sobre el mapa anidado, tenemos que dar el número de índice del mapa con el bucle for
. Veamos un ejemplo en el que iteramos a través de un mapa anidado.
Código:
package main
import "fmt"
func main() {
DemoMap := map[int]map[string]string{
1: {
"one": "Delftstack1",
"two": "Delftstack2",
"three": "Delftstack3",
},
2: {
"four": "Delftstack4",
"five": "Delftstack5",
"Six": "Delftstack6",
},
3: {
"seven": "Delftstack7",
"eight": "Delftstack8",
"nine": "Delftstack9",
},
4: {
"ten": "Delftstack10",
"eleven": "Delftstack11",
"twelve": "Delftstack12",
},
}
for key, value := range DemoMap[3] {
fmt.Println(key, value)
}
}
El código anterior iterará sobre el mapa en la tercera posición en el mapa principal.
Producción :
seven Delftstack7
eight Delftstack8
nine Delftstack9
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook