How to Delete Key From Map in Go
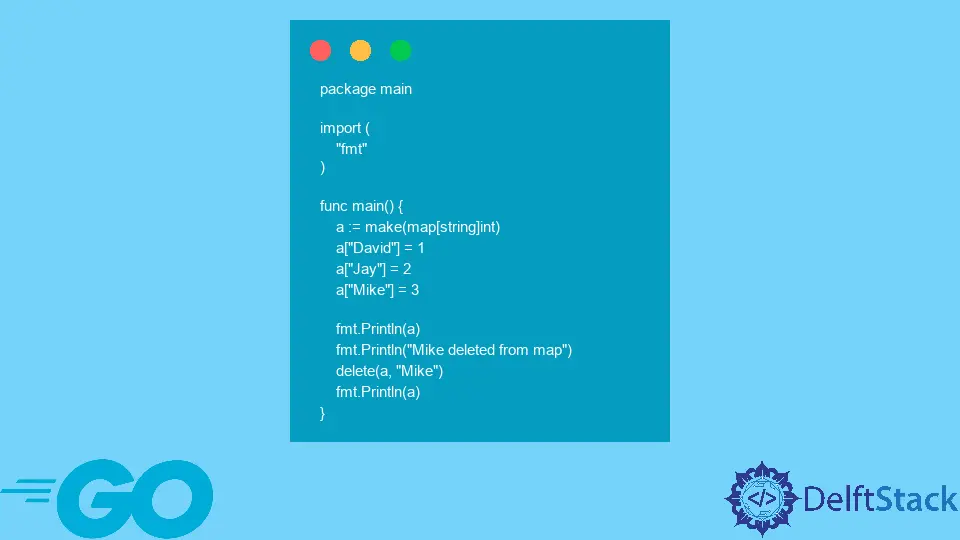
When working with maps in Go, managing key-value pairs efficiently is crucial. One common operation developers often need to perform is deleting a key from a map. This operation can help in managing memory and ensuring that your program operates smoothly.
In this tutorial, we will explore how to delete a key from a map in Go, providing you with clear code examples and explanations. Whether you are a beginner or an experienced developer, this guide will equip you with the knowledge to handle maps effectively in your Go projects.
Understanding Maps in Go
Before we dive into the specifics of deleting a key from a map, let’s take a moment to understand what maps are in Go. A map is a built-in data type that associates keys with values. They are similar to dictionaries in Python or hash tables in other programming languages. Maps in Go are unordered collections, meaning that the order of elements is not guaranteed.
To declare a map in Go, you can use the following syntax:
m := make(map[string]int)
Here, m
is a map that associates string keys with integer values. You can add elements to the map and retrieve them using their keys. However, as your application evolves, there might be instances where you need to remove certain keys from the map. This is where the delete
function comes into play.
Deleting a Key from a Map
The process of deleting a key from a map in Go is straightforward. You can use the built-in delete
function. The syntax is simple:
delete(map, key)
In this case, map
is the name of your map, and key
is the key you want to remove. Let’s see this in action with a practical example.
Example Code for Deleting a Key
Here’s a simple example that demonstrates how to delete a key from a map in Go:
package main
import "fmt"
func main() {
m := make(map[string]int)
m["apple"] = 1
m["banana"] = 2
m["cherry"] = 3
fmt.Println("Original map:", m)
delete(m, "banana")
fmt.Println("Map after deleting 'banana':", m)
}
Output:
Original map: map[apple:1 banana:2 cherry:3]
Map after deleting 'banana': map[apple:1 cherry:3]
In this example, we first create a map called m
and populate it with three key-value pairs. We then print the original map, delete the key "banana"
using the delete
function, and print the updated map. As you can see, the key-value pair for "banana"
has been successfully removed.
The delete
function is a powerful tool that allows you to manage your maps dynamically. It’s important to note that if you attempt to delete a key that doesn’t exist in the map, Go will not throw an error; it simply does nothing.
Conclusion
In this tutorial, we explored how to delete a key from a map in Go using the delete
function. We provided clear examples to illustrate the process, ensuring that you can easily understand and implement this operation in your own projects. Managing maps effectively is essential for writing efficient Go code, and knowing how to delete keys is a fundamental skill every Go developer should master.
With this knowledge in hand, you can now manipulate maps more effectively, enhancing the performance and functionality of your applications.
FAQ
-
How do I check if a key exists in a Go map?
You can check if a key exists in a Go map by using the comma-ok idiom. For example,value, exists := m[key]
will setexists
to true if the key is present. -
Can I delete multiple keys from a Go map at once?
No, you cannot delete multiple keys in a singledelete
call. You will need to calldelete
for each key you want to remove. -
What happens if I delete a key that does not exist in the map?
If you delete a key that does not exist, Go will not throw an error; it will simply ignore the operation. -
Are maps in Go thread-safe?
No, maps in Go are not thread-safe. If you need to access a map from multiple goroutines, you should use synchronization mechanisms like mutexes. -
Can I use any data type as a key in a Go map?
You can use any data type that is comparable as a key in a Go map. This includes basic types like strings and integers, but not slices or maps.