How to Undo a Git Stash
- Collect and Save Changes in the Directory in Git
-
Use the
git stash
Command to Save Staged and Unstaged Changes in the Directory in Git -
Use the
git stash pop
Command to Undogit stash
in Git -
Use
git stash apply
andgit stash drop
to Undogit stash
in Git - Conclusion
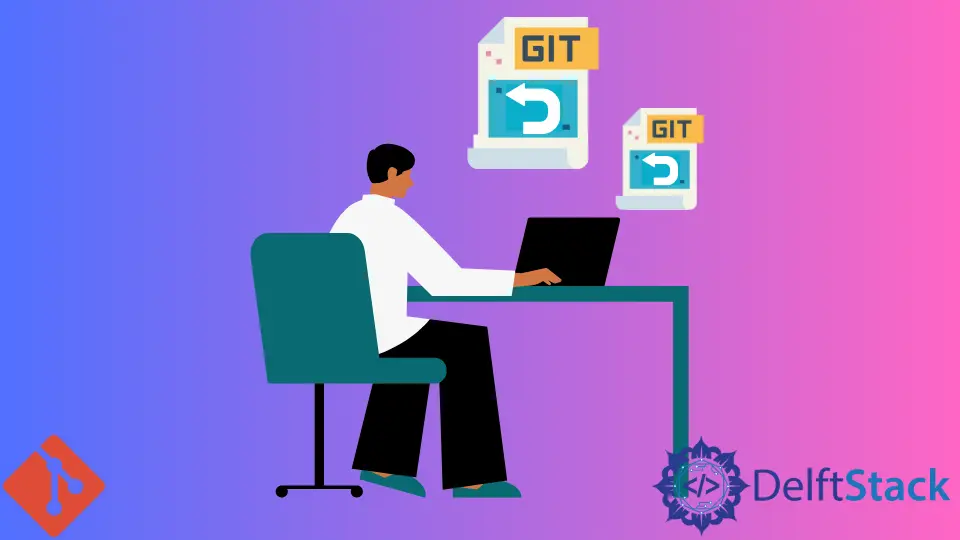
Git stash is a powerful feature that allows you to temporarily save your changes and revert to a clean working directory. However, there may be scenarios where you want to undo a stash.
This article explores different methods to achieve this, providing example code for each one. But before doing that, we must first know how to make and save changes to our repository.
Collect and Save Changes in the Directory in Git
Git allows you to save changes locally and push them to a server when needed. In Git, instead of using the term save
, we use commit
. We use the git add
, git commit
, and git stash
commands to save changes.
Use the git add
Command to Collect Changes in the Directory
The git add
command collects all changes in your project’s directory and brings them to the staging area. Practically, you instruct Git to update your files in the next commit.
You will have to run git commit
to save the updates. This is how you can use the git add
command to stage a file or folder for the next commit.
git add Tutorial.txt
For a directory:
git add -Delft
In our case, we have a Tutorial.txt
file in our working directory. If we made some changes in the file, we would use the git add Tutorial.txt
command to stage the commit’s changes.
Use the git commit
Command to Save Staged Changes in the Directory
We use the git commit
command to save any staged changes in our working directories. We use this command hand in hand with git add
.
These commits are like snapshots of the current state of your project.
Git allows you to gather the changes you feel are collected before pushing them to the central server. This way, only what is working and agreed upon can move to the central server.
Earlier on, we staged a file using this command.
git add Tutorial.txt
We use the git status
command to check the output below.
pc@JOHN MINGW64 ~/Git (main)
$ git status
On branch main
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
new file: Tutorial.txt
To save the changes, run git commit
.
pc@JOHN MINGW64 ~/Git (main)
$ git status
On branch main
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
new file: Tutorial.txt
$git commit
Output:
Summarize the commit on the first line. Leaving it blank will end the commit.
Use the git stash
Command to Save Staged and Unstaged Changes in the Directory in Git
Git stash is a mechanism for temporarily saving changes that are not ready to be committed. It’s particularly useful when you need to switch branches or fix a bug quickly without committing incomplete work.
We use the git stash
command to save staged and unstaged changes. Use the git status
command to check your dirty workspace.
$ git status
On branch main
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: Tutorial.txt
Changes not staged for commit:
(use "git add/rm <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
deleted: text.txt.txt
modified: text.txt.txt.bak
Use the git stash
command to save the above changes working on something else.
pc@JOHN MINGW64 ~/Git (main)
$ git stash
Saved working directory and index state WIP on main: 195e5c3 $git status
Running git status
:
pc@JOHN MINGW64 ~/Git (main)
$ git status
On branch main
nothing to commit, working tree clean
Create a Branch From Stash
If you want to retain the stash for future reference, you can create a branch from it.
git stash branch <branch-name> stash@{n}
The command above creates a new branch named <branch-name>
based on the state of the stash at index n
.
Use the git stash pop
Command to Undo git stash
in Git
git stash pop
is a command that combines two steps: applying the most recent stash and dropping it from the stash stack. This means it restores your changes and simultaneously removes the stash entry.
To undo a git stash
using git stash pop
, follow these steps:
-
Open your terminal or command line and navigate to the root directory of your Git repository.
-
Use the following command to apply the most recent stash:
pc@JOHN MINGW64 ~/Git (main) $ git stash pop Removing text.txt.txt On branch main Changes not staged for commit: (use "git add/rm <file>..." to update what will be committed) (use "git restore <file>..." to discard changes in working directory) modified: Tutorial.txt deleted: text.txt.txt modified: text.txt.txt.bak no changes added to commit (use "git add" and/or "git commit -a") Dropped refs/stash@{0} (e1fdba2aaecc32e7ad546de1586a2381f812a5dd)
It will re-apply your stash to your working copy.
-
After executing the command, Git will apply the changes from the stash to your working directory. You can now review the changes to ensure they were restored correctly.
-
Verify that the changes are as expected. If satisfied, you can commit them to your repository.
Use git stash pop
With Index
You can also use git stash pop
with an index to apply a specific stash.
git stash pop stash@{n}
This command applies the stash at index n
and drops it in one step.
Tips and Considerations
- If you have multiple stashes,
git stash pop
will apply the most recent one by default. If you want to apply a specific stash, you can usegit stash pop stash@{n}
wheren
is the index of the stash you want to apply. - If there are conflicts during the
git stash pop
operation, Git will notify you. You’ll need to resolve the conflicts manually. - After using
git stash pop
, the stash entry is removed from the stash stack. This means you can’t refer to it later. - If you want to keep the stash entry, you can use
git stash apply
followed bygit stash drop
.
Use git stash apply
and git stash drop
to Undo git stash
in Git
The simplest way to undo a Git stash is to apply the stash and then drop it. This restores your changes and removes the stash entry.
The git stash apply
command applies the most recent stash to your working directory. Your changes are restored, but the stash entry is retained.
The git stash drop
command discards the most recent stash. Since we’ve already applied the changes, dropping the stash entry ensures it doesn’t clutter your stash list.
To reverse a stash operation, we’ll employ a two-step process:
- Apply the Stash: This step restores the saved changes from the stash to the working directory.
- Drop the Stash: This step permanently deletes the stash entry from the stack.
Applying the Stash
To apply the most recent stash, execute the following command:
git stash apply
This command instructs Git to apply the latest stash. Your changes will be restored to the working directory, allowing you to review and integrate them.
Dropping the Stash
To remove the stash entry, use the following command:
git stash drop
This command deletes the most recent stash from the stack. The changes you applied in the previous step will remain in your working directory.
Combining git stash apply
and git stash drop
You can perform both operations in a single step using:
git stash pop
This command applies the stash and then drops it. It’s a convenient shortcut for applying and dropping stashes.
Apply a Specific Stash
If you have multiple stashes, you can apply a specific one by referring to its index.
git stash apply stash@{n}
git stash drop stash@{n}
git stash apply stash@{n}
: This applies the stash at indexn
.git stash drop stash@{n}
: This drops the stash at indexn
.
Tips and Considerations
- If you have multiple stashes,
git stash apply
andgit stash drop
will target the most recent stash by default. If you want to apply or drop a specific stash, usegit stash apply stash@{n}
orgit stash drop stash@{n}
, wheren
is the index of the stash. - If conflicts arise during the
git stash apply
operation, Git will alert you. You’ll need to resolve these conflicts manually. - Once a stash is dropped, it cannot be retrieved. Be certain that you won’t need the changes before executing
git stash drop
.
Conclusion
In conclusion, Git stash provides a versatile way to temporarily save changes and return to a clean working directory. However, situations may arise where you need to undo a stash. In this article, we’ve explored various methods to achieve this, providing detailed explanations and example code for each approach.
Whether you choose to use git stash apply
and git stash drop
or leverage the convenience of git stash pop
, each method serves as a valuable tool in managing your version control workflow. Additionally, we’ve covered how to apply specific stashes and even create branches from stashes for future reference.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn