How to Position Text Over an Image With CSS
- Method 1: Using Absolute Positioning
- Method 2: Using Flexbox
- Method 3: Using CSS Grid
- Conclusion
- FAQ
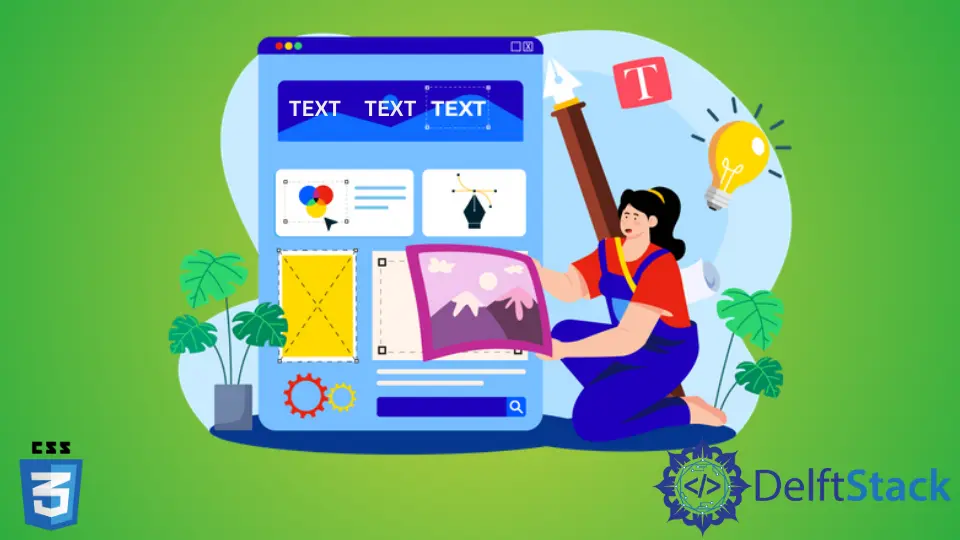
Positioning text over an image can enhance the visual appeal of your web pages, making them more engaging for users. Whether you’re designing a blog post, a portfolio, or a landing page, having well-placed text can significantly improve user experience.
In this tutorial, we will explore various CSS techniques to effectively position text over an image. We will cover methods using absolute positioning, flexbox, and grid layouts. By the end of this article, you’ll have a solid understanding of how to achieve this effect, making your designs more attractive and functional. Let’s dive in!
Method 1: Using Absolute Positioning
One of the most common ways to position text over an image is by using absolute positioning. This method allows you to place text in a specific location on the image, giving you precise control over its placement. Here’s how you can do it:
<div class="image-container">
<img src="your-image.jpg" alt="Example Image">
<div class="overlay-text">Your Text Here</div>
</div>
.image-container {
position: relative;
display: inline-block;
}
.image-container img {
width: 100%;
height: auto;
}
.overlay-text {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
color: white;
font-size: 24px;
text-shadow: 2px 2px 4px rgba(0, 0, 0, 0.7);
}
Output:
Text positioned over the center of the image
In this example, we first create a container for the image and the text. The position: relative;
style on the .image-container
allows us to position the text absolutely within it. The .overlay-text
class uses position: absolute;
, which means it will be placed relative to the nearest positioned ancestor, which is the image container. By setting top: 50%;
and left: 50%;
, we position the text in the center. The transform: translate(-50%, -50%);
shifts the text back by half its width and height, centering it perfectly. The text color and shadow enhance readability against varying image backgrounds.
Method 2: Using Flexbox
Flexbox is another powerful tool for positioning elements within a container. It allows for more flexible layouts compared to traditional methods. Here’s how you can use flexbox to position text over an image:
<div class="flex-container">
<img src="your-image.jpg" alt="Example Image">
<div class="flex-text">Your Text Here</div>
</div>
.flex-container {
display: flex;
justify-content: center;
align-items: center;
position: relative;
}
.flex-container img {
width: 100%;
height: auto;
}
.flex-text {
position: absolute;
color: white;
font-size: 24px;
text-shadow: 2px 2px 4px rgba(0, 0, 0, 0.7);
}
Output:
Text positioned over the center of the image using flexbox
In this method, we use a flex container to center the text. The display: flex;
style on the .flex-container
allows us to easily align items. However, since we want the text to overlay the image, we still set the text’s position to absolute. This way, we can control its placement while still benefiting from flexbox’s alignment features. The text remains centered over the image, and the same color and shadow styles enhance its visibility. This method is particularly useful when you want to create responsive designs that adapt well to different screen sizes.
Method 3: Using CSS Grid
CSS Grid is a powerful layout system that can also be used to position text over an image. It provides a two-dimensional layout, making it perfect for complex designs. Here’s how to use CSS Grid for this purpose:
<div class="grid-container">
<img src="your-image.jpg" alt="Example Image">
<div class="grid-text">Your Text Here</div>
</div>
.grid-container {
display: grid;
place-items: center;
position: relative;
}
.grid-container img {
width: 100%;
height: auto;
}
.grid-text {
position: absolute;
color: white;
font-size: 24px;
text-shadow: 2px 2px 4px rgba(0, 0, 0, 0.7);
}
Output:
Text positioned over the center of the image using CSS Grid
In this example, we set up a grid container with display: grid;
and place-items: center;
, which centers the contents of the grid. However, like the previous methods, we still position the text absolutely to overlay it on the image. This allows for precise control of the text’s position, while the grid layout can be beneficial for more complex arrangements. The text color and shadow enhance its visibility against the image, ensuring that it stands out regardless of the background.
Conclusion
Positioning text over an image with CSS is a straightforward yet powerful design technique that can significantly enhance your website’s aesthetics. Whether you choose absolute positioning, flexbox, or CSS grid, each method offers unique advantages. By mastering these techniques, you can create visually appealing layouts that engage your audience and improve user experience. Experiment with different styles and placements to find what works best for your design needs!
FAQ
-
How do I ensure text is readable over an image?
Use contrasting colors for the text and add text shadows or a semi-transparent background to improve visibility. -
Can I use background images instead of img tags?
Yes, you can use CSS background images with the same positioning techniques to overlay text. -
What is the best method for responsive design?
Flexbox and CSS Grid are both excellent choices for responsive designs, allowing for flexible layouts. -
How do I center text vertically and horizontally over an image?
Using absolute positioning withtop: 50%;
,left: 50%;
, andtransform: translate(-50%, -50%);
will center text both vertically and horizontally. -
Are there any accessibility considerations?
Always ensure that text over images is readable and consider providing alternative text for images for screen readers.