Field vs Property in C#
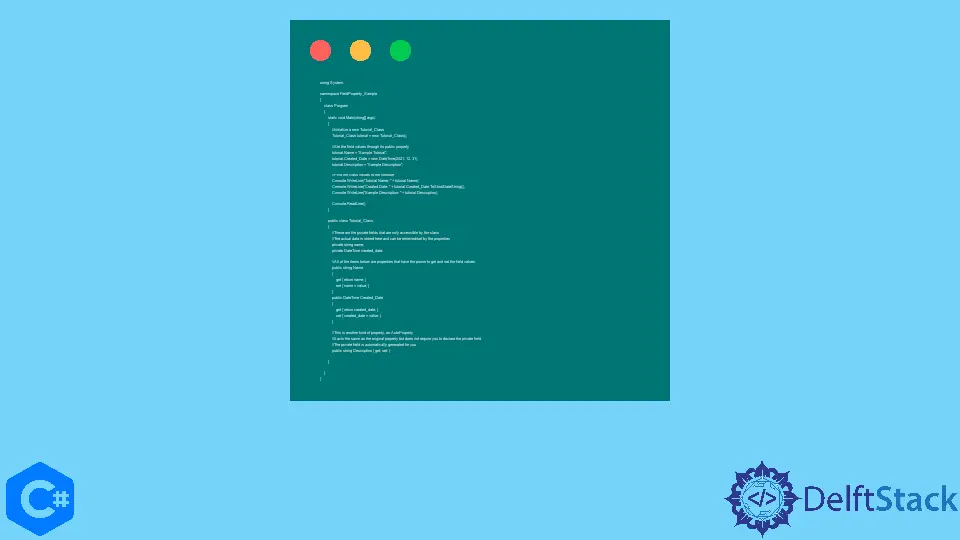
This tutorial will explain the difference between fields and properties in classes in C#. To better illustrate this, you must first know the following concepts.
What Is a Class?
A Class is a collection of data members that enables you to create your custom types by acting as a blueprint or a template for your objects. Within a class, fields, and properties are similar in that they can store data, but the difference is in how they are meant to be used.
What Is a Field?
A field
saves the data as a variable. It can be made public and then accessed through the class, but it is best practice to limit and hide as much data as possible. This provides you more control and avoids unexpected errors by ensuring that the only way a person can access the data is by utilizing one of the class’s public methods.
What Is a Property?
A property exposes fields. Using the properties instead of the fields directly provides a level of abstraction where you can change the fields while not affecting the external way they are accessed by the objects that use your class. Properties also allow you to do calculations before setting the field’s value or ensuring valid data.
Example:
using System;
namespace FieldProperty_Sample {
class Program {
static void Main(string[] args) {
// Initialize a new Tutorial_Class
Tutorial_Class tutorial = new Tutorial_Class();
// Set the field values through its public property
tutorial.Name = "Sample Tutorial";
tutorial.Created_Date = new DateTime(2021, 12, 31);
tutorial.Description = "Sample Description";
// Print the class values to the console
Console.WriteLine("Tutorial Name: " + tutorial.Name);
Console.WriteLine("Created Date: " + tutorial.Created_Date.ToShortDateString());
Console.WriteLine("Sample Description: " + tutorial.Description);
Console.ReadLine();
}
public class Tutorial_Class {
// These are the private fields that are only accessible by the class
// The actual data is stored here and can be retrieved/set by the properties
private string name;
private DateTime created_date;
// All of the items below are properties that have the power to get and set the field values
public string Name {
get { return name; }
set { name = value; }
}
public DateTime Created_Date {
get { return created_date; }
set { created_date = value; }
}
// This is another kind of property, an AutoProperty
// It acts the same as the original property but does not require you to declare the private
// field The private field is automatically generated for you
public string Description { get; set; }
}
}
}
In the example above, we have a sample class that uses fields, properties, and AutoProperties. After initializing an instance of Tutorial_Class, we set the values using the class’s public properties. You can note that if you try to access the fields directly, you will get an error Program.Tutorial_Class.x is inaccessible due to its protection level
. Finally, we print the values to the console to show that the changes have been made.
Output:
Tutorial Name: Sample Tutorial
Created Date: 31/12/2021
Sample Description: Sample Description