C#의 필드 대 속성
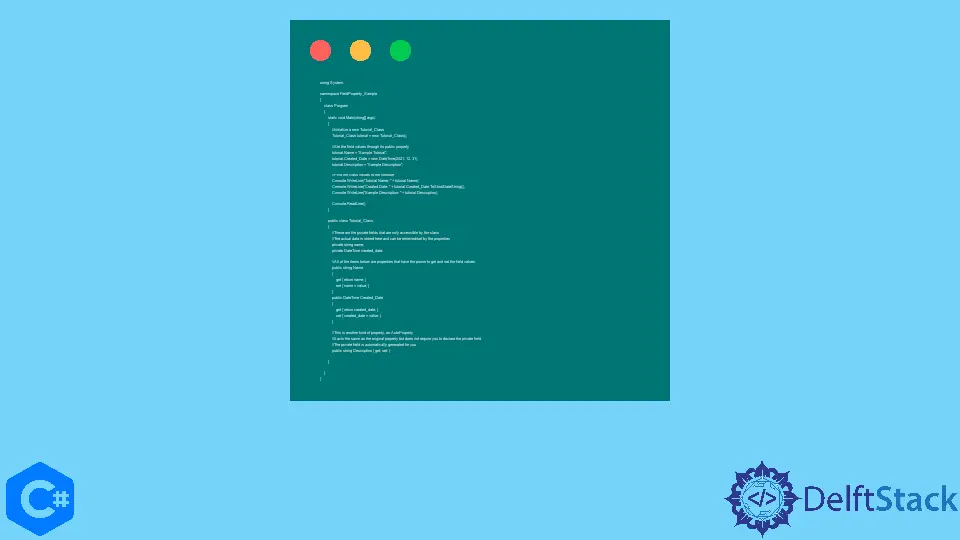
이 자습서에서는 C# 클래스의 필드와 속성의 차이점을 설명합니다. 이를 더 잘 설명하려면 먼저 다음 개념을 알아야 합니다.
수업이란 무엇입니까?
클래스는 개체에 대한 청사진 또는 템플릿 역할을 하여 사용자 정의 유형을 생성할 수 있는 데이터 멤버의 모음입니다. 클래스 내에서 필드 및 속성은 데이터를 저장할 수 있다는 점에서 유사하지만 차이점은 사용 방법에 있습니다.
필드란?
필드
는 데이터를 변수로 저장합니다. 공개한 다음 클래스를 통해 액세스할 수 있지만 가능한 한 많은 데이터를 제한하고 숨기는 것이 가장 좋습니다. 이렇게 하면 사람이 데이터에 액세스할 수 있는 유일한 방법은 클래스의 공용 메서드 중 하나를 사용하는 것임을 확인하여 더 많은 제어를 제공하고 예기치 않은 오류를 방지할 수 있습니다.
속성이란 무엇입니까?
속성은 필드를 노출합니다. 필드 대신 속성을 직접 사용하면 클래스를 사용하는 개체가 액세스하는 외부 방식에 영향을 주지 않으면서 필드를 변경할 수 있는 추상화 수준을 제공합니다. 또한 속성을 사용하면 필드 값을 설정하거나 유효한 데이터를 확인하기 전에 계산을 수행할 수 있습니다.
예시:
using System;
namespace FieldProperty_Sample {
class Program {
static void Main(string[] args) {
// Initialize a new Tutorial_Class
Tutorial_Class tutorial = new Tutorial_Class();
// Set the field values through its public property
tutorial.Name = "Sample Tutorial";
tutorial.Created_Date = new DateTime(2021, 12, 31);
tutorial.Description = "Sample Description";
// Print the class values to the console
Console.WriteLine("Tutorial Name: " + tutorial.Name);
Console.WriteLine("Created Date: " + tutorial.Created_Date.ToShortDateString());
Console.WriteLine("Sample Description: " + tutorial.Description);
Console.ReadLine();
}
public class Tutorial_Class {
// These are the private fields that are only accessible by the class
// The actual data is stored here and can be retrieved/set by the properties
private string name;
private DateTime created_date;
// All of the items below are properties that have the power to get and set the field values
public string Name {
get { return name; }
set { name = value; }
}
public DateTime Created_Date {
get { return created_date; }
set { created_date = value; }
}
// This is another kind of property, an AutoProperty
// It acts the same as the original property but does not require you to declare the private
// field The private field is automatically generated for you
public string Description { get; set; }
}
}
}
위의 예에는 필드, 속성 및 AutoProperties를 사용하는 샘플 클래스가 있습니다. Tutorial_Class의 인스턴스를 초기화한 후 클래스의 public 속성을 사용하여 값을 설정합니다. 필드에 직접 액세스하려고 하면 Program.Tutorial_Class.x는 보호 수준으로 인해 액세스할 수 없습니다.
라는 오류가 표시됩니다. 마지막으로 콘솔에 값을 인쇄하여 변경 사항이 적용되었음을 보여줍니다.
출력:
Tutorial Name: Sample Tutorial
Created Date: 31/12/2021
Sample Description: Sample Description