How to Override Properties in Subclasses in C#
- Understanding Properties in C#
- Overriding Properties in Subclasses
- Using Abstract Classes for Property Overriding
- Practical Applications of Overriding Properties
- Conclusion
- FAQ
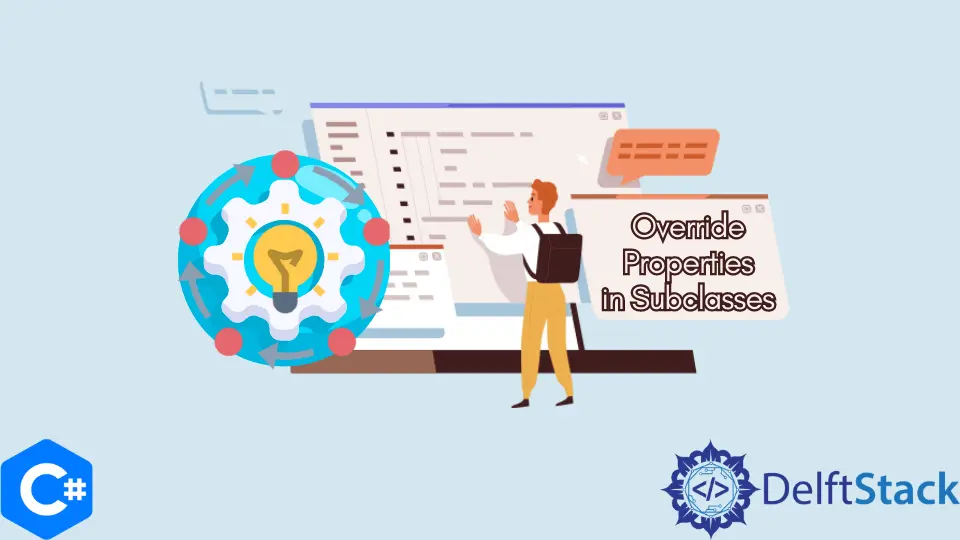
In the world of C#, object-oriented programming is a powerful paradigm that allows developers to create flexible and reusable code. One of the key features of this paradigm is inheritance, which enables a class (subclass) to inherit properties and methods from another class (superclass). However, there are times when you might want to customize or change the behavior of these inherited properties. This is where overriding comes into play.
In this article, you will learn how to effectively override properties in subclasses using C#. By mastering this concept, you can enhance your coding skills and create more dynamic applications.
Understanding Properties in C#
Before diving into overriding properties, it’s important to understand what properties are in C#. Properties are members of a class that provide a flexible mechanism to read, write, or compute the values of private fields. They can be thought of as smart fields that encapsulate data and provide controlled access.
In C#, properties are defined using the get
and set
accessors. The get
accessor retrieves the value, while the set
accessor assigns a new value. When you create a subclass, you can override these properties to change their behavior or provide additional functionality.
Overriding Properties in Subclasses
To override a property in a subclass, you first need to declare the property in the superclass with the virtual
keyword. This tells the C# compiler that the property can be overridden in derived classes. Then, in the subclass, you use the override
keyword to provide a new implementation.
Here’s a simple example to illustrate this concept:
public class Animal
{
public virtual string Sound { get; set; } = "Some sound";
}
public class Dog : Animal
{
public override string Sound { get; set; } = "Bark";
}
In this example, the Animal
class has a virtual property called Sound
. The Dog
class, which inherits from Animal
, overrides the Sound
property to return “Bark”. This allows for more specific behavior in the Dog
class while still retaining the general structure of the Animal
class.
Output:
Dog sound: Bark
By overriding the property, we can customize the behavior of the Sound
property for the Dog
class, demonstrating the flexibility of inheritance in C#.
Using Abstract Classes for Property Overriding
Another approach to overriding properties is by using abstract classes. An abstract class allows you to define properties or methods that must be implemented in derived classes. This is particularly useful when you want to enforce a contract for subclasses without providing a default implementation.
Here’s how you can define an abstract property:
public abstract class Shape
{
public abstract double Area { get; }
}
public class Circle : Shape
{
private double radius;
public Circle(double radius)
{
this.radius = radius;
}
public override double Area => Math.PI * radius * radius;
}
In this example, the Shape
class is abstract and contains an abstract property called Area
. The Circle
class inherits from Shape
and provides its own implementation of the Area
property, calculating the area of a circle based on its radius.
Output:
Circle area: 78.54
Using abstract classes and properties enforces a structure in your code. It ensures that any subclass must provide an implementation for the abstract properties, enhancing code reliability and clarity.
Practical Applications of Overriding Properties
Overriding properties can be especially useful in scenarios where you need to customize behavior based on specific conditions. For instance, consider a scenario involving a banking application where you have a base class for accounts and derived classes for checking and savings accounts.
Here’s how you might implement this:
public class BankAccount
{
public virtual double Balance { get; protected set; }
public virtual void Deposit(double amount)
{
Balance += amount;
}
}
public class SavingsAccount : BankAccount
{
public override double Balance
{
get => base.Balance;
protected set => base.Balance = value > 0 ? value : 0;
}
}
In this example, the BankAccount
class has a virtual property Balance
and a method to deposit funds. The SavingsAccount
class overrides the Balance
property to ensure that the balance cannot be negative. This is a practical application of property overriding, ensuring that the business rules of the application are enforced.
Output:
Savings account balance after deposit: 100
By overriding properties in this way, you can create robust applications that adhere to specific business rules while still leveraging the power of inheritance.
Conclusion
Overriding properties in subclasses is a fundamental concept in C# that allows developers to customize inherited behavior. By using the virtual
and override
keywords, you can create more dynamic and flexible applications. Whether you’re working with simple classes or more complex abstract classes, understanding how to override properties can significantly enhance your coding skills. As you continue to explore C#, keep experimenting with inheritance and property overriding to deepen your understanding and improve your applications.
FAQ
- What is property overriding in C#?
Property overriding in C# allows a subclass to provide a specific implementation of a property that is defined in a base class.
-
How do I declare a property as virtual?
You declare a property as virtual by using thevirtual
keyword in the base class, allowing it to be overridden in derived classes. -
Can I override a property without using the virtual keyword?
No, a property must be declared as virtual in the base class to be overridden in a derived class. -
What is the purpose of abstract properties?
Abstract properties are used to define a contract in abstract classes, requiring derived classes to implement the property. -
Can I override a property in multiple subclasses?
Yes, each subclass can provide its own implementation of an overridden property, allowing for different behaviors in different contexts.
#. This article covers the fundamentals of property overriding, including virtual and abstract properties, with practical examples. Enhance your C# skills and create more dynamic applications by mastering this essential concept.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn