How to Create the Auto Property in C#
-
Use Classes to Implement the Auto Property in
C#
-
Use the
Mono.Reflection
Library or NuGet Package to Assign Values to Backing Fields of Auto Property inC#
-
Create Class Object to Modify the Auto Property in
C#
-
Use Encapsulation to Create Read-Only or Write-Only Auto Property in
C#
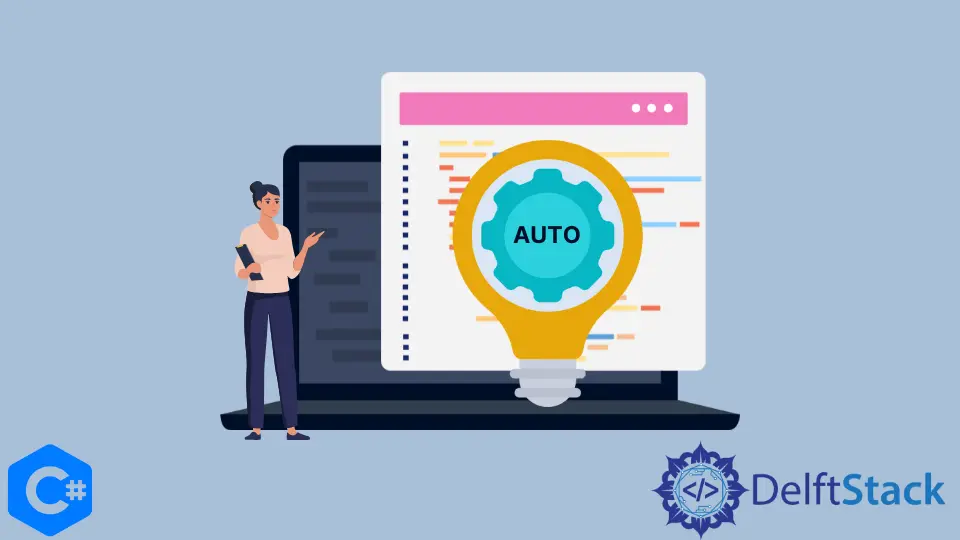
You can always refactor a variable into a property and vice versa. There are many valid reasons to make a trivial property because if you rely on reflection and want to bind data, it’s easier to use all properties.
In this tutorial, you will learn everything related to auto property and ways to create it in C#.
In modern C# programming, using auto-properties is a great practice and the best option to maintain efficiency in code writing while maintaining performance and best practices.
These properties generally represent methods but can work all right when implemented in interfaces. These properties perform as contracts to guarantee the presence of certain methods within any interface, class, or namespace that implements them.
Use Classes to Implement the Auto Property in C#
A proper way to initialize auto-properties is by using classes, i.e.:
public class my_class {
public int my_property { get; set; }
}
This way, the code becomes more readable and easier to understand as the auto properties convey intent and ensure consistency. Also, they are the quickest way to define pairs of get
and set
methods.
It is often considered the best approach to define auto properties because as your code evolves and you introduce different logic, your code doesn’t need to re-compile. Furthermore, the auto property is a coding shortcut to save you a few keystrokes by only typing public string new_property {get; set;}
and letting the compiler generate the rest by default.
using System;
using System.Windows.Forms;
namespace auto_property {
public class NetworkDevice {
public string IP { get; set; } // custom types | represent elements and belongs to the property
public string drr_add { get; set; }
public string num_serial { get; set; }
}
// pre-defined part of the Windows Form
public partial class Form1 : Form {
public Form1() {
// initialize the design or other components created at design time
InitializeComponent();
}
// represents the on-click event for the button to create the auto property
private void button1_Click(object sender, EventArgs e) {
NetworkDevice new_device = new NetworkDevice();
new_device.IP = "Red_dead Search 12-D Class A";
new_device.drr_add = "NYC, New York";
new_device.num_serial = "S11-E2237654-C";
MessageBox.Show(
$"The device name: {new_device.IP} \nThe location: {new_device.drr_add} \nThe serial: {new_device.num_serial}",
"Auto Property", MessageBoxButtons.OKCancel, MessageBoxIcon.Warning);
}
}
}
Output:
The device name: Red_dead Search 12-D Class A
The location: NYC, New York
The serial: 511-E2237654-C
Since C# version 3.0, the object-oriented programming dilemma is no longer present. You can skip lines with private
and don’t need the definitions for the get
and set
methods, either.
In conclusion, the auto properties helped many programmers to recognize their, and the result was maintainable code. It is not a primary requirement to use field declaration for the get
and set
methods to modify the fields, as the compiler handles each one perfectly.
It is by default in the compiler’s functionality to create or generate the private fields and ensure mapping. Additionally, it populates the get
and set
methods to allow the read and write of the specific fields belonging to the auto properties.
Use the Mono.Reflection
Library or NuGet Package to Assign Values to Backing Fields of Auto Property in C#
Creating auto-properties is a more concrete approach to creating properties without requiring additional or in-depth logic in the property accessors.
The anonymous backing fields of auto-properties are modifiable using the property’s get
and set
methods. The property declaration enables you to write code that can create objects and enable compilers to create private backing fields.
In general, the property is automatic if it has a backing field generated by the compiler, which saves you from writing primitive getters and setters that must return the value of the backing field or assign it to it. Its syntax is much shorter than creating a general property and requires a public class and a public string with the get
and set
methods.
The CompilerGenerated
attribute makes the auto property unique as its name is visible for users, but the compiler generates the body of its get
and set
accessors. You can assign values to the backing fields of an auto property; however, doing so is not recommended and not considered a best practice because it affects performance and decreases security and protection, which, in a sense, removes the purpose of creating these properties in the first place.
using System;
using System.Windows.Forms;
using Mono.Reflection; // include after installing `Mono.Relection 2.0.0` NuGet package for your C#
// project
namespace auto_property {
public class mono_exp {
public const string name_official = "Great organization name with an excellent track record!";
public string name_company { get; } = name_official;
private string code_registration;
// to assign new values to the `code_registration`
public string pri_reg {
get { return code_registration; } // read
set { code_registration = value; } // write
}
// to read-only as an output
public string name_display {
// return the new value of `code_registration`
get {
return name_company + ": " + code_registration;
}
set {} // empty
}
}
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
var company_name =
typeof(mono_exp).GetProperty(nameof(mono_exp.name_company)).GetBackingField();
var registration_no =
typeof(mono_exp).GetProperty(nameof(mono_exp.pri_reg)).GetBackingField();
MessageBox.Show($"{company_name.Name}, {registration_no.Name}", "Auto Property",
MessageBoxButtons.OKCancel, MessageBoxIcon.Warning);
}
}
}
Output:
<name_company>k__BackingField, code_registration
You can write some helper methods, assign values using reflection, or assign expected states to objects when unit testing them. It’s recommended to set the expected state of a class directly without calling the setters because doing so can start a chain of events not important in testing or optimizing your code.
It’s crucial to find the backing fields for auto properties before assigning them values. One idea is to use the BackingFieldResolver
by Jb Evain and add the Mono.Reflection
NuGet package to your solution to add this library.
Throw ArgumentException
at every search for backing fields to make your code more efficient and enhance its readability.
Create Class Object to Modify the Auto Property in C#
Using automatic properties can make the application programming interfaces future-proof and make data binding possible. The concept of the auto-property implementation is completely understandable using the class objects, and making changes to objects can enhance your understanding of the different behaviors of the auto properties.
The auto property accessors (get
and set
methods) to modify and access properties are introduced with C# 3.0, and the functionality of these properties is further enhanced with the introduction of init
accessors in the C# 9.0 version. As you can’t declare auto-properties in interfaces, you must define a body that can be a class, and to call any function or attribute from the auto property, you need to create a new class object.
A class object can help you access the auto property elements in an interface and opens many possibilities to the developers. One way of initializing auto-properties and their elements is by using:
public string {get; set;} = "example_text"
Doing so also enables your complex classes to contain significant behavior (behavioral includes methods) and data.
using System;
using System.Windows.Forms;
namespace auto_property {
public class new_element {
public double element_ip { get; set; }
public string element_serial { get; set; }
public int element_drr { get; set; }
public new_element(double IP_add, string ser_no, int counter_ddr) {
element_ip = IP_add;
element_serial = ser_no;
element_drr = counter_ddr;
}
}
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
new_element element_new = new new_element(2860.01, "Red_Dead", 9743822);
element_new.element_ip += 499.99;
MessageBox.Show(
$"{element_new.element_ip}, {element_new.element_serial}, {element_new.element_drr}",
"Auto Property", MessageBoxButtons.OKCancel, MessageBoxIcon.Warning);
}
}
}
Output:
3360, Red_Dead, 9743822
When property accessors do not require any additional logic, that’s where auto-properties are used and defined as public int some_property {get; set;}
. Automatic properties allow you to create something concise and concrete as the design philosophy behind them, e.g., never exposing fields as public but always accessing everything via properties.
The 3.0 or later versions of C# enable client code to create and modify objects, leading to more concise property declaration and the creation of high-performance auto-properties. Developing in Visual Studio allows breakpoints on get
and set
as you can modify them without breaking any contract with the property caller and make changes using reflection because of an unobvious source of change.
Use Encapsulation to Create Read-Only or Write-Only Auto Property in C#
Encryption makes it easy to handle and modify auto-properties, but you still can make changes inside the class, and it is not immutable, as you can still access the private setter through reflection. As of C# 6.0 and later, you can create true read-only
and write-only
auto-properties.
The read-only
automatic properties can be immutable properties that cannot be changed outside of the constructer:
public string new_property { get; }
public new_class() {
this.new_property = "something";
}
Afterward, this immutable (read-only) property at compile time will become something like this:
readonly string new_name;
public string new_property {
get { return this.new_name; }
}
public new_class() {
this.new_name = "something";
}
As a result, doing so can save you a lot of time by optimizing the code and terminating the excess code. You must have a basic understanding of encapsulation to achieve a successful auto property by declaring fields/variables as private and providing public get
and set
methods to access and update the value of a private field.
Besides increased security and enhanced flexibility, the auto properties can enable you to make properties either read-only (by only using the get
method) or write-only (by only using the set
method).
Directly assigning values to automatic properties seems clean and beautiful. Still, it’s an illusion, and after building code in release mode, removing the program debug database, and opening the resulting library with a decompiler, you will see the difference.
using System;
using System.Windows.Forms;
namespace auto_property {
public class new_element {
/*
// write only auto property example
private int _int;
public int _newint
{
set { _int = value; }
}
*/
public double element_ip { get; set; }
public string element_serial { get; } // read-only
public int element_drr { get; } // read-only
public new_element(double IP_add, string ser_no, int counter_ddr) {
element_ip = IP_add;
element_serial = ser_no;
element_drr = counter_ddr;
}
}
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
new_element element_new = new new_element(2860.01, "Red_Dead", 9743822);
element_new.element_ip += 499.99; // modification
MessageBox.Show(
$"{element_new.element_ip}, {element_new.element_serial}, {element_new.element_drr}",
"Auto Property", MessageBoxButtons.OKCancel, MessageBoxIcon.Warning);
}
}
}
Output:
3360, Red_Dead, 9743822
As there is so much friction between variables and properties, Kevin Dente introduced a new syntax, public property int example_var;
, as the properties do the exact thing as variables but with better granular control over visibility. The auto properties should be lightweight and can be refactored into explicit methods if they incur significant effort.
The auto property is more effective than the regular property, and it’s not anti-pattern, as it also receives the same optimizations as the regular one; the only difference is that backing fields are automatically generated. This tutorial taught you how to define, optimize, and modify the auto property in C#.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub