How to Implement a Read-Only Property in C#
-
Declare the Read-Only Property on a Variable When Initializing in
C#
-
Implement a Read-Only Property Using a Simple
get
Property inC#
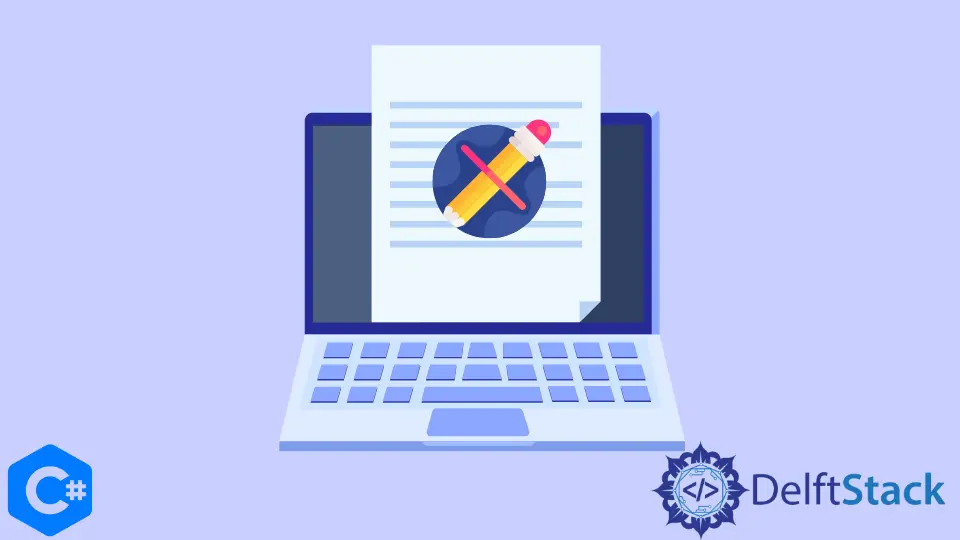
Today we will be learning how to make a read-only property in C#, so it can only be read and not modified.
Declare the Read-Only Property on a Variable When Initializing in C#
We have worked on the class CAR
for the last two articles. Let’s use the same class and try to implement a read-only property. Let’s say that our class CAR
has a property known as the maker_id
that we want to keep read-only for security purposes.
using System;
class CAR {
private readonly int maker_id;
public CAR(int maker_id) {
this.maker_id = maker_id;
}
public int get_maker_id() {
return maker_id;
}
}
public class Program {
static void Main(String[] args) {
CAR x = new CAR(5);
Console.WriteLine(x.get_maker_id());
}
}
So we have declared the maker_id
as readonly
. If you run the above, the output will be:
5
So let’s say we now desire to add another setter for our maker_id
field. We can then do something as follows:
public void set_maker_id(int maker_id) {
this.maker_id = maker_id;
}
But, you will now notice that it throws an error:
So you can see that the readonly
property only lets us assign the value once to a variable in the constructor. Else, it tends to throw errors and denies modification due to accessibility.
The full code is shown below:
using System;
class CAR {
private readonly int maker_id;
public CAR(int maker_id) {
this.maker_id = maker_id;
}
public void set_maker_id(int maker_id) {
this.maker_id = maker_id; // line ERROR
}
public int get_maker_id() {
return maker_id;
}
}
public class Program {
static void Main(String[] args) {
CAR x = new CAR(5);
Console.WriteLine(x.get_maker_id());
}
}
The error has been mentioned in the comments in the code.
Implement a Read-Only Property Using a Simple get
Property in C#
We can write the maker_id
property as follows:
private int maker_id { get; }
And if you try to write a function as follows:
public void set_val(int val) {
this.maker_id = val;
}
An error will be produced as follows:
So you see how setting the get
property makes it a read-only. The complete code is as follows:
using System;
class CAR {
private int maker_id { get; set; }
public CAR(int maker_id) {
this.maker_id = maker_id;
}
}
class Program {
static void Main(String[] args) {
CAR x = new CAR(5);
}
}
Not only does this follow convention rules, but it also tends to break serializers. Also, it is not changing and tends to be immutable.
In case you want to make sure get
returns something, make the code as follows:
class CAR {
public int maker_id {
get { return maker_id; }
}
}
So that is how you implement a read-only in C#.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub