High Performance TCP Server in C#
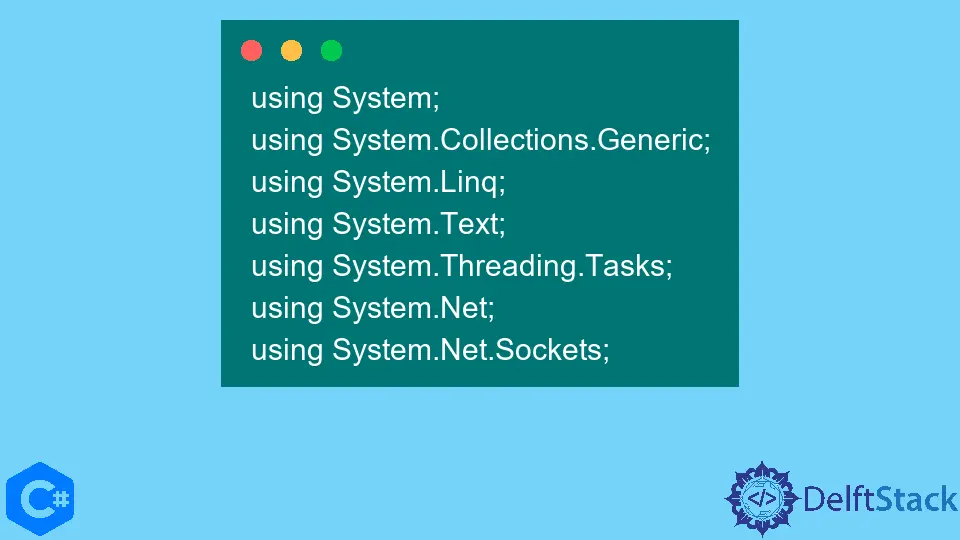
The following article will teach you how to use the C# programming language to create a TCP server that has excellent performance, is scalable, and can manage many connections.
High-Performance TCP Server in C#
An approach referred to as an Asynchronous TCP
server allows numerous clients to be connected at once, with each client operating on its thread. All of this is accomplished using relatively brief and straightforward code to keep in your head.
Example:
To begin, import the following libraries:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
First, we’ll create an object of TcpListener
and give it the name Tcpserver
.
TcpListener Tcpserver = new TcpListener(IPAddress.Any, 9999);
Now, to accept a connection from a client to the server, we’ll need to build a method called StartOperations()
. This method will be called by using the BeginAcceptTcpClient
.
public void StartOperations() {
Tcpserver.Start();
Console.WriteLine("The Current Server is : 9999");
Console.WriteLine("The local End point :" + Tcpserver.LocalEndpoint);
Console.WriteLine("Waiting for a connection request..");
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
}
We will send the ServerClientCommunication()
function to the BeginAcceptTcpClient
function, executing on a separate thread picked from the threadpool. This will save you from the need to construct threads manually.
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
The ServerClientCommunication()
function is given an IAsyncResult
argument, and it is this argument that is responsible for keeping the connection active. This method will then repeatedly call StartOperations()
, causing the program to switch continuously between several threads.
public void ServerClientCommunication(IAsyncResult res) {
s TcpClient tcpClient = Tcpserver.EndAcceptTcpClient(res);
NetworkStream ns = tcpClient.GetStream();
Console.WriteLine("Here Write your Logic");
}
Furthermore, you can specify the logic or code you wish to execute in the ServerClientCommunication()
function.
Complete Source Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
namespace tcpServerbyZeeshan {
class Program {
static void Main(string[] args) {
Program sample = new Program();
sample.StartOperations();
Console.ReadLine();
}
TcpListener Tcpserver = new TcpListener(IPAddress.Any, 9999);
public void StartOperations() {
Tcpserver.Start();
Console.WriteLine("The Current Server is : 9999");
Console.WriteLine("The local End point :" + Tcpserver.LocalEndpoint);
Console.WriteLine("Waiting for a connection request..");
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
}
public void ServerClientCommunication(IAsyncResult res) {
TcpClient tcpClient = Tcpserver.EndAcceptTcpClient(res);
NetworkStream ns = tcpClient.GetStream();
Console.WriteLine("Here Write your Logic");
}
}
}
Output:
The Current Server is : 9999
The local End point :0.0.0.0:9999
Waiting for a connection request..
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn