Servidor TCP de alto rendimiento en C#
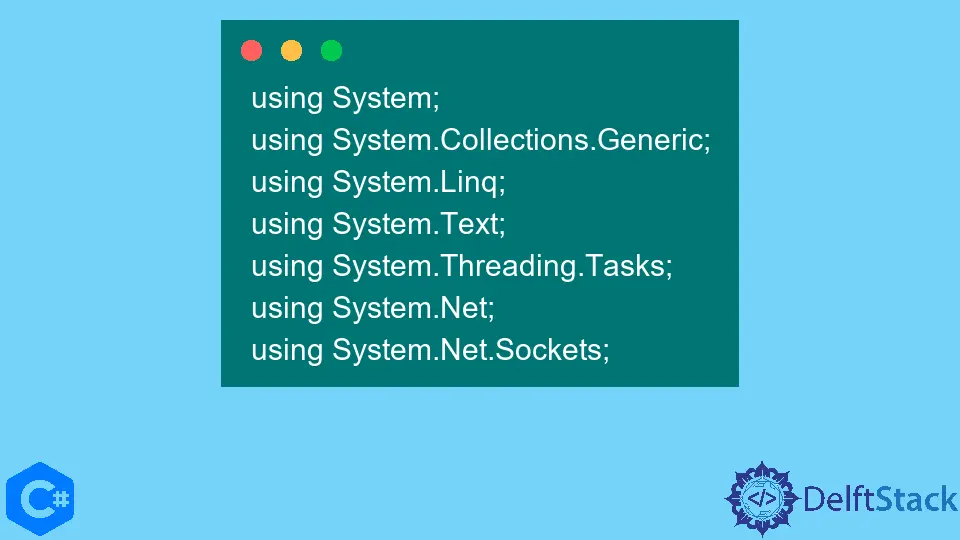
El siguiente artículo le enseñará cómo usar el lenguaje de programación C# para crear un servidor TCP que tenga un rendimiento excelente, sea escalable y pueda administrar muchas conexiones.
Servidor TCP de Alto Rendimiento en C#
Un enfoque denominado servidor TCP asíncrono
permite que numerosos clientes se conecten a la vez, con cada cliente operando en su subproceso. Todo esto se logra utilizando un código relativamente breve y sencillo para tenerlo en la cabeza.
Ejemplo:
Para comenzar, importe las siguientes bibliotecas:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
Primero, crearemos un objeto de TcpListener
y le daremos el nombre Tcpserver
.
TcpListener Tcpserver = new TcpListener(IPAddress.Any, 9999);
Ahora, para aceptar una conexión de un cliente al servidor, necesitaremos construir un método llamado StartOperations()
. Este método será llamado usando el BeginAcceptTcpClient
.
public void StartOperations() {
Tcpserver.Start();
Console.WriteLine("The Current Server is : 9999");
Console.WriteLine("The local End point :" + Tcpserver.LocalEndpoint);
Console.WriteLine("Waiting for a connection request..");
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
}
Enviaremos la función ServerClientCommunication()
a la función BeginAcceptTcpClient
, ejecutándose en un subproceso separado seleccionado del grupo de subprocesos. Esto le ahorrará la necesidad de construir subprocesos manualmente.
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
A la función ServerClientCommunication()
se le asigna un argumento IAsyncResult
, y es este argumento el responsable de mantener activa la conexión. Este método llamará repetidamente a StartOperations()
, lo que hará que el programa cambie continuamente entre varios subprocesos.
public void ServerClientCommunication(IAsyncResult res) {
s TcpClient tcpClient = Tcpserver.EndAcceptTcpClient(res);
NetworkStream ns = tcpClient.GetStream();
Console.WriteLine("Here Write your Logic");
}
Además, puedes especificar la lógica o el código que deseas ejecutar en la función ServerClientCommunication()
.
Código fuente completo:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
namespace tcpServerbyZeeshan {
class Program {
static void Main(string[] args) {
Program sample = new Program();
sample.StartOperations();
Console.ReadLine();
}
TcpListener Tcpserver = new TcpListener(IPAddress.Any, 9999);
public void StartOperations() {
Tcpserver.Start();
Console.WriteLine("The Current Server is : 9999");
Console.WriteLine("The local End point :" + Tcpserver.LocalEndpoint);
Console.WriteLine("Waiting for a connection request..");
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
}
public void ServerClientCommunication(IAsyncResult res) {
TcpClient tcpClient = Tcpserver.EndAcceptTcpClient(res);
NetworkStream ns = tcpClient.GetStream();
Console.WriteLine("Here Write your Logic");
}
}
}
Producción :
The Current Server is : 9999
The local End point :0.0.0.0:9999
Waiting for a connection request..
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn