C#의 고성능 TCP 서버
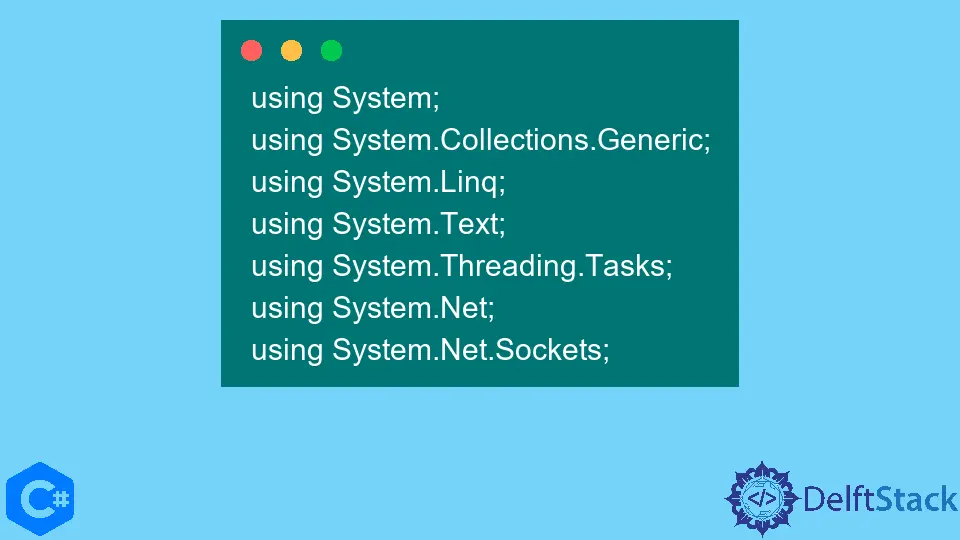
다음 기사에서는 C# 프로그래밍 언어를 사용하여 성능이 뛰어나고 확장 가능하며 많은 연결을 관리할 수 있는 TCP 서버를 만드는 방법을 설명합니다.
C#
의 고성능 TCP 서버
비동기 TCP
서버라고 하는 접근 방식을 사용하면 각 클라이언트가 해당 스레드에서 작동하는 여러 클라이언트를 한 번에 연결할 수 있습니다. 이 모든 작업은 비교적 간단하고 직관적인 코드를 사용하여 수행됩니다.
예:
시작하려면 다음 라이브러리를 가져옵니다.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
먼저 TcpListener
개체를 만들고 Tcpserver
라는 이름을 지정합니다.
TcpListener Tcpserver = new TcpListener(IPAddress.Any, 9999);
이제 클라이언트에서 서버로의 연결을 수락하려면 StartOperations()
라는 메서드를 빌드해야 합니다. 이 메서드는 BeginAcceptTcpClient
를 사용하여 호출됩니다.
public void StartOperations() {
Tcpserver.Start();
Console.WriteLine("The Current Server is : 9999");
Console.WriteLine("The local End point :" + Tcpserver.LocalEndpoint);
Console.WriteLine("Waiting for a connection request..");
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
}
ServerClientCommunication()
함수를 BeginAcceptTcpClient
함수로 보내 스레드 풀에서 선택한 별도의 스레드에서 실행합니다. 이렇게 하면 스레드를 수동으로 구성할 필요가 없습니다.
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
ServerClientCommunication()
함수에는 IAsyncResult
인수가 제공되며 연결을 활성 상태로 유지하는 역할을 하는 것이 바로 이 인수입니다. 그런 다음 이 메서드는 StartOperations()
를 반복적으로 호출하여 프로그램이 여러 스레드 간에 계속 전환되도록 합니다.
public void ServerClientCommunication(IAsyncResult res) {
s TcpClient tcpClient = Tcpserver.EndAcceptTcpClient(res);
NetworkStream ns = tcpClient.GetStream();
Console.WriteLine("Here Write your Logic");
}
또한 ServerClientCommunication()
기능에서 실행하려는 논리 또는 코드를 지정할 수 있습니다.
완전한 소스 코드:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
namespace tcpServerbyZeeshan {
class Program {
static void Main(string[] args) {
Program sample = new Program();
sample.StartOperations();
Console.ReadLine();
}
TcpListener Tcpserver = new TcpListener(IPAddress.Any, 9999);
public void StartOperations() {
Tcpserver.Start();
Console.WriteLine("The Current Server is : 9999");
Console.WriteLine("The local End point :" + Tcpserver.LocalEndpoint);
Console.WriteLine("Waiting for a connection request..");
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
}
public void ServerClientCommunication(IAsyncResult res) {
TcpClient tcpClient = Tcpserver.EndAcceptTcpClient(res);
NetworkStream ns = tcpClient.GetStream();
Console.WriteLine("Here Write your Logic");
}
}
}
출력:
The Current Server is : 9999
The local End point :0.0.0.0:9999
Waiting for a connection request..
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn