C# の高性能 TCP サーバー
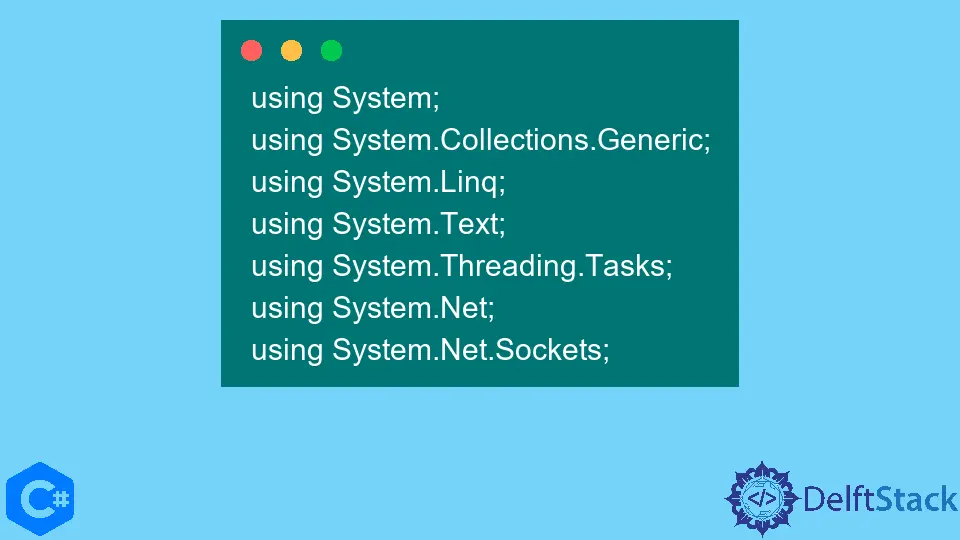
次の記事では、C# プログラミング言語を使用して、優れたパフォーマンスとスケーラビリティを備え、多くの接続を管理できる TCP サーバーを作成する方法について説明します。
C#
の高性能 TCP サーバー
Asynchronous TCP
サーバーと呼ばれるアプローチにより、多数のクライアントを一度に接続し、各クライアントをそのスレッドで動作させることができます。 これらはすべて、頭の中に入れておきたい比較的簡潔で単純なコードを使用して実行されます。
例:
まず、次のライブラリをインポートします。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
まず、TcpListener
のオブジェクトを作成し、Tcpserver
という名前を付けます。
TcpListener Tcpserver = new TcpListener(IPAddress.Any, 9999);
ここで、クライアントからサーバーへの接続を受け入れるために、StartOperations()
というメソッドを作成する必要があります。 このメソッドは、BeginAcceptTcpClient
を使用して呼び出されます。
public void StartOperations() {
Tcpserver.Start();
Console.WriteLine("The Current Server is : 9999");
Console.WriteLine("The local End point :" + Tcpserver.LocalEndpoint);
Console.WriteLine("Waiting for a connection request..");
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
}
ServerClientCommunication()
関数を BeginAcceptTcpClient
関数に送信し、スレッドプールから選択された別のスレッドで実行します。 これにより、手動でスレッドを構築する必要がなくなります。
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
ServerClientCommunication()
関数には IAsyncResult
引数が与えられ、接続をアクティブに保つ役割を担うのはこの引数です。 その後、このメソッドは StartOperations()
を繰り返し呼び出し、プログラムが複数のスレッド間で継続的に切り替えられるようにします。
public void ServerClientCommunication(IAsyncResult res) {
s TcpClient tcpClient = Tcpserver.EndAcceptTcpClient(res);
NetworkStream ns = tcpClient.GetStream();
Console.WriteLine("Here Write your Logic");
}
さらに、ServerClientCommunication()
関数で実行するロジックまたはコードを指定できます。
完全なソース コード:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
namespace tcpServerbyZeeshan {
class Program {
static void Main(string[] args) {
Program sample = new Program();
sample.StartOperations();
Console.ReadLine();
}
TcpListener Tcpserver = new TcpListener(IPAddress.Any, 9999);
public void StartOperations() {
Tcpserver.Start();
Console.WriteLine("The Current Server is : 9999");
Console.WriteLine("The local End point :" + Tcpserver.LocalEndpoint);
Console.WriteLine("Waiting for a connection request..");
Tcpserver.BeginAcceptTcpClient(ServerClientCommunication, Tcpserver);
}
public void ServerClientCommunication(IAsyncResult res) {
TcpClient tcpClient = Tcpserver.EndAcceptTcpClient(res);
NetworkStream ns = tcpClient.GetStream();
Console.WriteLine("Here Write your Logic");
}
}
}
出力:
The Current Server is : 9999
The local End point :0.0.0.0:9999
Waiting for a connection request..
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn