How to Get the Current Method Name in C#
-
Obtain the Current Method Name With
MethodBase.GetCurrentMethod()
in C# -
Obtain the Current Method Name With the
StackTrace
Class in C# -
Obtain the Current Method Name With the
nameof
Operator in C# -
Obtain the Current Method Name With
CallerMemberName
in C# - Conclusion
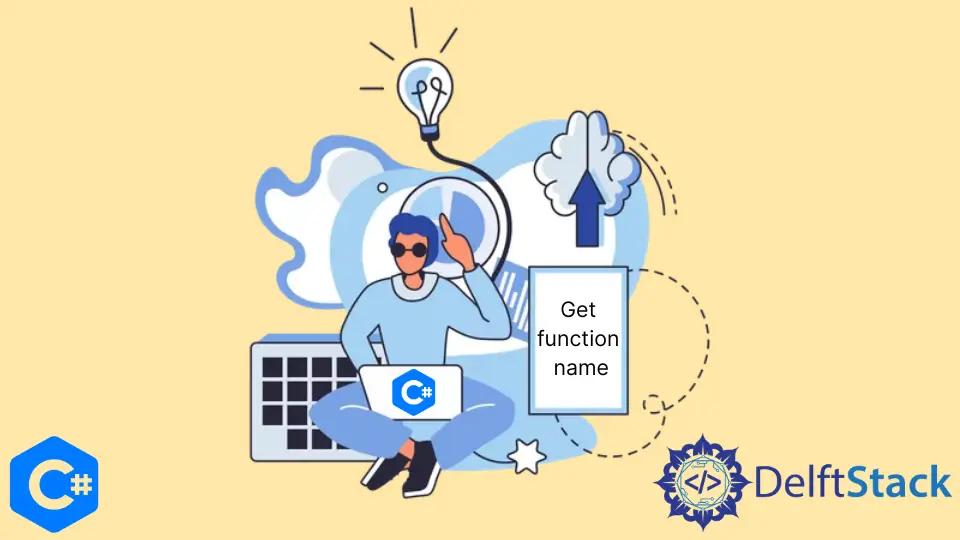
In this article, we’ll learn how to utilize C# to get the name of the current method being executed. In C#, there is more than one way to get the method’s name, which is currently being executed.
The following procedures, which operate similarly, are outlined, discussed, and carried out below.
Obtain the Current Method Name With MethodBase.GetCurrentMethod()
in C#
MethodBase.GetCurrentMethod()
is a method provided by the .NET Framework in the System.Reflection
namespace. It returns a MethodBase
object that represents the method that is currently being executed.
MethodBase
is an abstract class that provides information about methods, constructors, and properties, and it is part of the broader reflection functionality in C#.
From this MethodBase
object, you can extract information about the method, including its name.
Here’s a step-by-step guide on how to use MethodBase.GetCurrentMethod()
to get the current method name:
To use MethodBase.GetCurrentMethod()
, you need to include the System.Reflection
namespace in your C# code file. You can do this using the using
directive at the beginning of your file:
using System.Reflection;
Once you have imported the System.Reflection
namespace, you can call MethodBase.GetCurrentMethod()
to get a MethodBase
object representing the current method and then extract the method name.
Here’s an example:
using System;
using System.Reflection;
class Program
{
static void Main(string[] args)
{
string methodName = MethodBase.GetCurrentMethod().Name;
Console.WriteLine("Current method name: " + methodName);
AnotherMethod();
}
static void AnotherMethod()
{
string methodName = MethodBase.GetCurrentMethod().Name;
Console.WriteLine("Current method name: " + methodName);
}
}
In this code, we start with the Main
method, which is the entry point of our program. It retrieves the name of the current method using MethodBase.GetCurrentMethod()
, stores it in a variable called methodName
, and prints it to the console using Console.WriteLine
.
However, to ensure the AnotherMethod
gets executed and its name displayed, we call it within the Main
method using AnotherMethod();
.
AnotherMethod
is a separate method defined in our program. Just like Main
, it also retrieves the current method’s name using MethodBase.GetCurrentMethod()
, and then it prints this name to the console.
Compile and run your C# program. You will see that the method name of the currently executing method is displayed in the console. In this example, the output will be:
Current method name: Main
Current method name: AnotherMethod
As you can see, MethodBase.GetCurrentMethod().Name
correctly identifies the names of the methods from which it is called.
Here’s an alternative way to obtain the name of the currently executing method. Here’s the code:
using System;
class CurrentMethodName {
private static void Main(string[] args) {
var methodName = System.Reflection.MethodBase.GetCurrentMethod().Name;
Console.WriteLine("Current method is " + methodName);
}
}
When you run this program, you will see the following output:
Current method is Main
While using MethodBase.GetCurrentMethod()
can be handy for various purposes, it’s essential to keep a few considerations in mind:
-
Retrieving method information through reflection (such as
MethodBase.GetCurrentMethod()
) can be relatively slow compared to other methods. Therefore, it should be used judiciously and not in performance-critical code. -
Be prepared for exceptions, especially when using
MethodBase.GetCurrentMethod()
in scenarios where the calling method might be dynamic or unknown. -
This approach is particularly useful for logging and debugging. It allows you to record the method name when an error occurs or to trace the flow of your application.
-
To make the most of this technique, it’s a good practice to use meaningful method names in your code. Clear and descriptive method names help you understand the context better.
While it’s a useful feature, it should be used with caution due to potential performance implications, and it may not always behave as expected in all scenarios. In modern .NET applications, you may prefer to use the CallerMemberName
attribute for improved efficiency and compatibility.
Obtain the Current Method Name With the StackTrace
Class in C#
Another way to obtain the name of the currently executing method, which can be useful for logging, debugging, and error handling, is to use the StackTrace
class from the System.Diagnostics
namespace.
The StackTrace
class is part of the System.Diagnostics
namespace in C# and provides a powerful tool for retrieving information about the call stack. It allows you to inspect the methods that have been called up to the current point in your code execution.
To get the name of the currently executing method using the StackTrace
class, you will need to access a specific frame in the call stack and extract the method name from it.
To access the current method, you typically want to get the top frame, which is where the execution is at the moment. You can do this using stackTrace.GetFrame(0)
, where 0
indicates the top frame.
After that, you extract the method name from the frame using GetMethod().Name
. The GetMethod()
method provides access to method-related information, and .Name
retrieves the method name.
Here’s a demonstration of how to use StackTrace
to get the current method name:
Before you can use the StackTrace
class, you need to include the System.Diagnostics
namespace in your C# code file. You can do this by adding the using
directive at the beginning of your code:
using System.Diagnostics;
Once you have imported the System.Diagnostics
namespace, you can create an instance of the StackTrace
class and use it to retrieve the name of the currently executing method.
using System;
using System.Diagnostics;
class Program
{
static void Main(string[] args)
{
string methodName = GetCurrentMethodName();
Console.WriteLine("Current method name: " + methodName);
}
static string GetCurrentMethodName()
{
StackTrace stackTrace = new StackTrace();
StackFrame stackFrame = stackTrace.GetFrame(1); // Index 1 represents the current method
MethodBase methodBase = stackFrame.GetMethod();
return methodBase.Name;
}
}
In this code, we start with the Main
method, which is the entry point of our program. We create a StackTrace
object and use it to access the current method’s name.
To do this, we retrieve a StackFrame
object at index 1
, which represents the current method. From the StackFrame
, we access the MethodBase
and return its name.
Compile and run your C# program. It will display the name of the currently executing method, which, in this example, will be the Main
method. The output will look like this:
Current method name: Main
While using StackTrace
to retrieve the current method name is a valuable approach, it’s important to consider a few key points:
-
Retrieving method information through
StackTrace
can be relatively slow compared to other methods, such asMethodBase.GetCurrentMethod()
. Therefore, it should be used judiciously and not in performance-critical code. -
In the code example, we retrieve the current method’s name by accessing the
StackFrame
at index1
. You may need to adjust the index if you are working with different scenarios or calling methods. -
This method is particularly useful for debugging and logging, as it allows you to track method names during the program’s execution.
-
Be prepared for exceptions when using
StackTrace
, especially if the calling method might be dynamic or unknown.
Obtain the Current Method Name With the nameof
Operator in C#
The nameof
operator is a powerful feature introduced in C# 6.0. It enables you to obtain the name of a variable, type, property, or method as a string at compile time.
This operator is particularly advantageous when you want to retrieve the name of the current method, as it bypasses the need for complex techniques like reflection or reliance on external libraries.
Here’s how to use the nameof
operator to retrieve the current method name:
using System;
class Program
{
static void Unique()
{
string methodName = nameof(Unique);
Console.WriteLine("Current method is " + methodName);
}
public static void Main(string[] args)
{
Unique();
}
}
In the provided code, we start with the Main
method, which serves as the entry point for our program.
To obtain the name of the current method, we simply use the nameof
operator followed by the method name we want to extract, which, in this instance, is nameof(Unique)
. This operator is then applied to the Unique
method.
Compile and run your C# program, and you’ll witness the display of the currently executing method’s name.
In this example, the output will be:
Current method is Unique
The code successfully identifies and showcases the names of the methods where the nameof
operator is utilized.
While the nameof
operator is a straightforward and efficient way to acquire the current method name, there are a few key considerations to remember:
-
The
nameof
operator evaluates at compile time, not at runtime. This ensures efficiency and accuracy in obtaining the method’s name. -
It’s imperative to ensure that the method names used with
nameof
exactly match the method names in your code. Any typos or inconsistencies can result in incorrect outcomes. -
nameof
solely provides the name of the method in which it is used and doesn’t offer access to other methods or the complete call stack. -
The
nameof
operator is especially beneficial for tasks such as debugging and logging. It simplifies the identification of the current method where issues may arise and facilitates tracking application flow.
By using nameof
, your code for checking arguments or referencing method names becomes more straightforward to maintain. When method names change, you won’t need to manually update string constants throughout your code.
Unlike other approaches that require attaching additional using
statements (such as reflection
namespaces), the nameof
operator doesn’t necessitate attaching using System.Diagnostics;
or using System.Reflection;
.
It’s important to note that the nameof
operator is available in C# version 6 and later. If you are using an older version of C#, you may not have access to this feature.
Obtain the Current Method Name With CallerMemberName
in C#
The CallerMemberName
attribute is a valuable addition to the .NET framework introduced in C# 5.0. It allows you to obtain the name of the calling method, property, or event, which is particularly useful for logging and debugging purposes.
This attribute is part of the System.Runtime.CompilerServices
namespace, and it can be used as a method parameter with a default value, allowing you to omit it when making method calls.
Here’s how to use the CallerMemberName
attribute:
using System;
using System.Runtime.CompilerServices;
public class Example
{
public void LogMethod([CallerMemberName] string caller = "")
{
Console.WriteLine($"The current method is: {caller}");
}
}
class Program
{
static void Main()
{
Example example = new Example();
example.LogMethod();
}
}
In this example, the LogMethod
method takes an optional parameter, caller
, which is tagged with the [CallerMemberName]
attribute. When you call LogMethod()
without specifying the caller
argument, the compiler will automatically provide the name of the calling method.
As a result, the output will be the name of the method that invoked LogMethod
.
Output:
The current method is: Main
Similar to the nameof
operator, the CallerMemberName
attribute is evaluated at compile time, ensuring efficiency and accuracy in acquiring the method name.
It’s imperative to ensure that the method names provided to CallerMemberName
match the method names in your code. Any typos or inconsistencies can result in incorrect outcomes.
Using the CallerMemberName
attribute offers several advantages.
By using CallerMemberName
, your code for checking arguments or referencing method names becomes more straightforward to maintain. When method names change, you won’t need to manually update string constants throughout your code.
Additionally, unlike other approaches that require attaching additional using
statements, the CallerMemberName
attribute doesn’t necessitate attaching using System.Runtime.CompilerServices;
.
Conclusion
In conclusion, this article explored four methods for obtaining the current method name in C#: MethodBase.GetCurrentMethod()
, StackTrace
, nameof
operator, and CallerMemberName
attribute. Each method has its strengths and should be chosen based on specific use cases, balancing performance, and code simplicity.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn