Abstract Function vs Virtual Function in C#
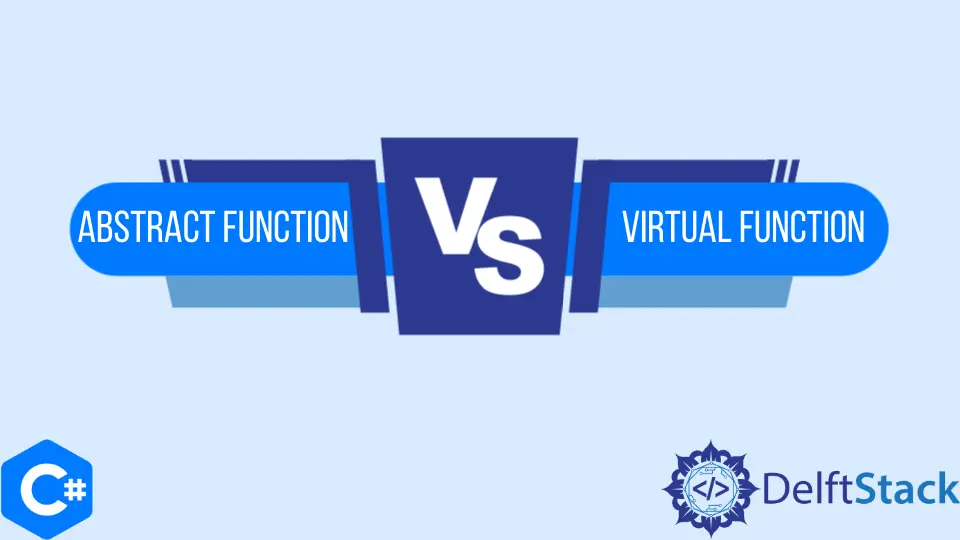
This tutorial will compare the abstract function and virtual function in C#.
Abstract Function in C#
An abstract function has no definition of itself in C#. It means that each child class has to override the abstract function and provide its own definition of the abstract function. An abstract function can only be declared inside an abstract class. The abstract
keyword is used to create abstract classes and functions in C#. The following code example shows us how to create an abstract function with the abstract
keyword in C#.
using System;
namespace abstract_vs_virtual {
public abstract class parentClass {
public abstract void name();
}
class Program : parentClass {
public override void name() {
Console.WriteLine("This is Child Class");
}
static void Main(string[] args) {
Program p = new Program();
p.name();
}
}
}
Output:
This is Child Class
We created an abstract class parentClass
and declared an abstract function name
inside the parentClass
class. We inherited the Program
class from the parentClass
and override the name()
function. In the main
function, we initialize an instance of the Program
class and call the name()
function.
Virtual Function in C#
A virtual function has its own definition, but it also allows the child classes to override it and have their own definition of the same function. The virtual
keyword is used to specify that a function is a virtual function in C#. The following code example shows us how we can create a virtual function in C#.
using System;
namespace abstract_vs_virtual {
public class parentClass {
public virtual void name() {
Console.WriteLine("This is the Parent Class");
}
}
class Program : parentClass {
static void Main(string[] args) {
Program p = new Program();
p.name();
parentClass pc = new parentClass();
pc.name();
}
}
}
Output:
This is the Parent Class
This is the Parent Class
In the above code, we created a class parentClass
and defined a virtual function name()
inside the parentClass
class. We then inherited the Program
class from the parentClass
and did not override the name()
function inside the Program
class. In the main function, we created instances of both Program
class and the parentClass
and called the name()
function with both instances. The function call p.name()
gave us the output This is the Parent Class
because there is no definition of the name()
function inside the Program
class.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn